在 Python 中获取长度不确定的输入值
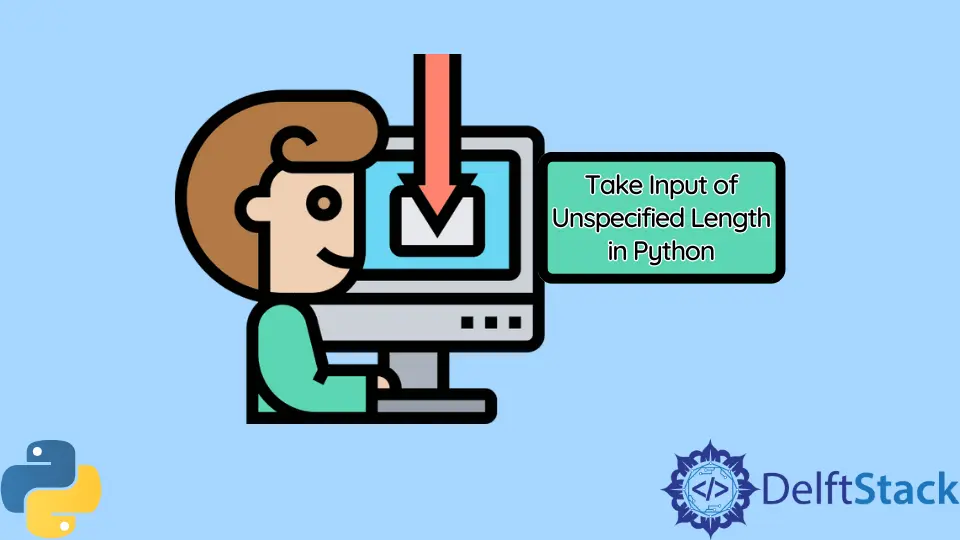
本文将介绍在输入长度未知的情况下,我们如何在 Python 中获取多个输入。
在 Python 中使用 stdin
获取未指定长度的输入
要使用 sys
模块获取输入,我们可以使用 sys.stdin
。
Python stdin
代表标准输入,它使我们能够直接从命令行获取输入。在接受输入时,stdin
在内部调用 input()
方法并在每一行之后添加一个 \n
。
例子:
import sys
print("Input something")
for x in sys.stdin:
if "end" == x.rstrip():
break
print(f"Either input something or input end to quit: {x}")
print("Exit")
输出:
Input something
Pasta
Either input something or input end to quit: Pasta
Watermelon
Either input something or input end to quit: Watermelon
Banana
Either input something or input end to quit: Banana
Noodles
Either input something or input end to quit: Noodles
end
Exit
在这里,我们首先导入 sys
模块。然后,我们使用 for
循环来连续获取输入。
在这个 for
循环中,我们有一个 if
语句来检查输入是否等于字符串 end
。如果是,那么我们跳出循环。
请注意,我们可以指定任何字符串,例如 quit
或 q
来代替 end
。我们必须告诉用户输入什么才能退出。
请注意,我们还可以在此代码中输入整数数据类型。但是,最好将输入显式更改为整数,因为 Python 会将每个输入转换为字符串。
我们可以使用 int()
函数来执行此操作。
import sys
print("Give an input")
for val in sys.stdin:
if "end" == val.rstrip():
break
val = int(val)
print(f"Either input something or input end to quit: {val}")
print("Exit")
输出:
Give an input
10
Either input something or input end to quit: 10
12
Either input something or input end to quit: 12
Dumbledore
Traceback (most recent call last):
File "tutorial.py", line 6, in <module>
x = int(x)
ValueError: invalid literal for int() with base 10: 'Dumbledore\n'
如上图,我们只能输入整数数据类型,否则会报错。
在 Python 中使用 while
循环获取未指定长度的输入
当我们不知道必须接受输入的次数时,我们可以使用 while
循环。
例子:
while True:
x = input("Grocery: ")
if x == "done":
break
输出:
Grocery: Vinegar
Grocery: Soya sauce
Grocery: done
在这里,我们使用 True
关键字来定义一个每次都会评估为真的条件。这样,用户不断得到输入提示。
但是,如果我们不定义中断条件,这个程序将永远不会终止。因此,在 while
块中,我们定义了一个 break
条件,当它接收到值 done
时将把控制带出循环。
请注意,我们可以使用诸如 quit
、bye
、0
等任何字符串来代替字符串 done
。
例子:
while True:
print("Input 0 to exit")
x = int(input("Enter the number: "))
if x == 0:
print("Bye")
break
输出:
Input 0 to exit
Enter the number: 4
Input 0 to exit
Enter the number: 5
Input 0 to exit
Enter the number: 3
Input 0 to exit
Enter the number: 0
Bye
这个例子告诉用户输入 0
退出。除了在 while 循环中使用 True
关键字外,我们还可以指定一个评估为 true 的条件。
在 Python 中使用等价的 C++ cin
函数获取未指定长度的输入
让我们在 Python 中创建与下面的 C++ 代码类似的辅助函数。
while (cin >> var) {
// code here
}
在 C++ 中,我们可以使用上述方法来获取未指定长度的输入。请注意,在 C++ 中,cin>>var
以两种方式工作,作为输入运算符和作为布尔表达式。
在 Python 中,接受输入的等价物是 var = input()
,它仅充当输入通道。我们将使用一个名为 ‘var’ 的列表对象来模拟 Python 的 cin>>var
行为。
例子:
# Function that works like the cin function in C++
def cin(var):
try:
var[0] = int(input())
return True
except:
return False
# main function similar to that of C++
def main():
var = [0]
while cin(var):
print("Value:", var[0])
main()
输出
5
Value: 5
7
Value: 7
3
Value: 3
9
Value: 9
6
Value: 6
4
Value: 4
>>>
首先,要知道 var
是一个列表对象。因此,如果我们在辅助方法中更改 var[0]
的值,更改将发生在 main
方法中。
在 cin
函数中,我们在变量 var[0]
中获取输入。此外,使用 try
语句,我们每次获得整数输入时都返回 True
。
请注意,如果我们按下 Enter 键而不是输入,则上述代码中的代码将停止执行。不仅如此,输入除整数以外的任何内容都会停止代码执行。
在 main
函数内部,我们不断地使用 while
循环来调用 cin
函数。在此示例中,我们采用整数输入,但我们可以根据需要进行更改。