How to Read CSV Line by Line in Python
- File Structure of a CSV File
-
Read CSV File Line by Line Using
csv.reader
in Python -
Read CSV File Line by Line Using
DictReader
Object in Python - Conclusion
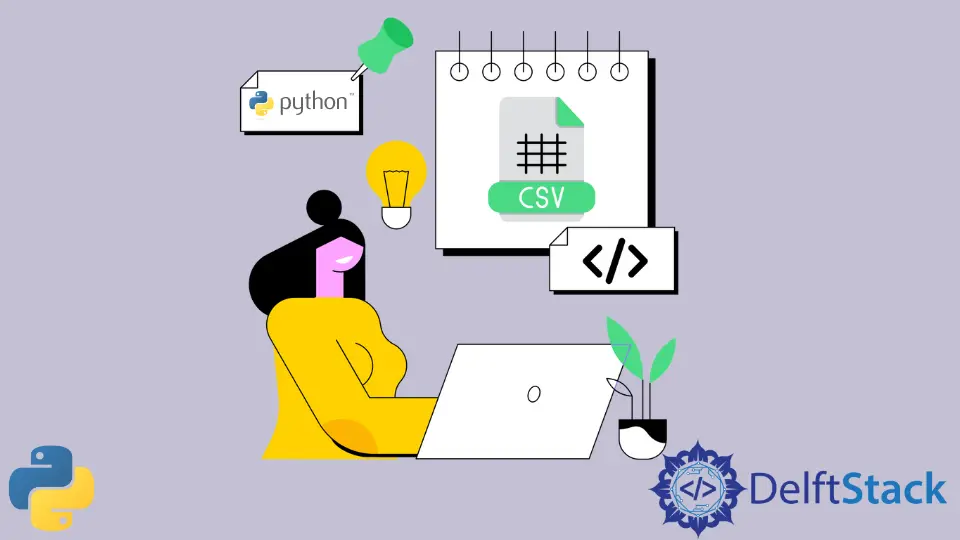
In Python, reading a file and printing it column-wise is common. But reading the file row by row might get a bit confusing sometimes.
This article will tackle how to read a CSV file line by line in Python. We will use the Python csv
module to deal with the CSV files in Python.
Before reading the CSV file line by line, let us first look at the file format of the CSV files. This will help us to manipulate the CSV files in a better manner.
File Structure of a CSV File
CSV
stands for Comma Separated Values
; it is a simple file format that stores data in tables. These tables could be in the form of a spreadsheet or a database.
We can also make a CSV file in a simple text editor like Notepad
. Each CSV file line corresponds to one record of the table.
Moreover, each record has one or more fields. The cross-section of a field and a record is called a cell. These fields are separated by commas (,
).
Sometimes, we also call this comma a delimiter. Note that this format gets its name from using the comma as a field separator. CSV files are widely used because of their compatibility with many programs, databases, spreadsheets, and word processing software.
Let us now create a CSV file using the file structure described above. After creating the file, we will read the CSV file line by line using different functions.
We can create a CSV file using a spreadsheet in Microsoft Excel. However, if you don’t have Microsoft Excel installed in your system, you can use Notepad or other text editors to make a CSV file.
We can change the file extension to .csv
to do this. Also, do not forget to follow the format of a CSV file. Here are all the steps we need to perform.
-
Open a text editor and write the content in the correct CSV format. The headers, as well as the records, are comma-separated. Each record starts in a new line. This is shown below:
Roll Number,Name,Subject 1,Harry Potter,Magical Creatures 2,Ron Weasley,Divination 3,Hermione Granger,Dark arts
Save this file as Demo.csv
. The CSV file will be created successfully.
We can use the open()
function to open the CSV file in Python. However, we prefer to use the python csv module made solely for this purpose. To use the csv module, we must import it first.
import csv
We will use the Demo.csv
file that we have already created to demonstrate. The file looks as follows:
Roll Number,Name,Subject
1,Harry Potter,Magical Creatures
2,Ron Weasely,Divination
3,Hermione Granger,Dark arts
To read the content of this CSV line by line in Python, we will use the csv module, which further provides two classes. These classes are csv.reader
and csv.DictReader
.
Let us look at these classes one by one.
Read CSV File Line by Line Using csv.reader
in Python
The csv.reader
class of the csv module enables us to read and iterate over the lines in a CSV file as a list of values. Look at the example below:
from csv import reader
# open file
with open("Demo.csv", "r") as my_file:
# pass the file object to reader()
file_reader = reader(my_file)
# do this for all the rows
for i in file_reader:
# print the rows
print(i)
We use the reader object to iterate over the rows of the Demo.csv
file. The reader object acts as an iterator. This makes sure that only one line stays in the memory at one time.
Output:
['Roll Number', 'Name', 'Subject']
['1', 'Harry Potter', 'Magical Creatures']
['2', 'Ron Weasley', 'Divination']
['3', 'Hermione Granger', 'Dark arts']
Let us look at the functions used here.
Python’s open()
function is used to open a file. Once it opens a file, it returns a file object.
Syntax:
open(file_name, mode)
The parameter mode
specifies the mode we want to open the file. It can be read
, append
, write
, or create
.
The reader()
function is used to read a file. It returns an iterable reader object. In the above example, this iterable object is file_reader
, which must be clear from the use of for loop.
In the above example, the headers are also printed. We can print a CSV file without a header as well. Look at the following example:
from csv import reader
# skip the first line(the header)
with open("Demo.csv", "r") as my_file:
file_csv = reader(my_file)
head = next(file_csv)
# check if the file is empty or not
if head is not None:
# Iterate over each row
for i in file_csv:
# print the rows
print(i)
Output:
['1', 'Harry Potter', 'Magical Creatures']
['2', 'Ron Weasley', 'Divination']
['3', 'Hermione Granger', 'Dark arts']
Here, the headers are not printed. This approach works similarly to the previous approach except that we skip the first row during iteration. We have used the next()
function to skip the header.
The next()
function in Python returns the next item present in an iterator. Its syntax is described below.
Syntax:
next(iterable_object / iterable, default)
Iterable
or iterable object
is the set of values through which we have to iterate. default
is an optional parameter that is returned by the iterable if it reaches its end.
Read CSV File Line by Line Using DictReader
Object in Python
csv.reader
reads and prints the CSV file as a list.
However, the DictReader
object iterates over the rows of the CSV file as a dictionary. The way csv.reader
returns each row as a list, ObjectReader
returns each row as a dictionary.
Look at the example below:
from csv import DictReader
# open the file
with open("Demo.csv", "r") as my_file:
# passing file object to DictReader()
csv_dict_reader = DictReader(my_file)
# iterating over each row
for i in csv_dict_reader:
# print the values
print(i)
Output:
{'Roll Number': '1', 'Name': 'Harry Potter', 'Subject': 'Magical Creatures'}
{'Roll Number': '2', 'Name': 'Ron Weasley', 'Subject': 'Divinition'}
{'Roll Number': '3', 'Name': 'Hermione Granger', 'Subject': 'Dark arts'}
The DictReader
function is similar to the reader
function except for how it returns the information. It maps and returns the values as a dictionary
where the field names act as keys of the dictionary and the values consist of the data in a particular row.
Conclusion
In this article, we discussed the basics of CSV. We also saw the two ways to read a CSV line by line in Python. We also saw how we could create a CSV file on our own using a text editor like Notepad.
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python