How to Fix String Indices Must Be Integers Error in Python
- Understanding the Error
- Solution Method 1: Using Integer Indices
- Solution Method 2: Using Dictionaries Correctly
- Solution Method 3: Debugging the Code
- Conclusion
- FAQ
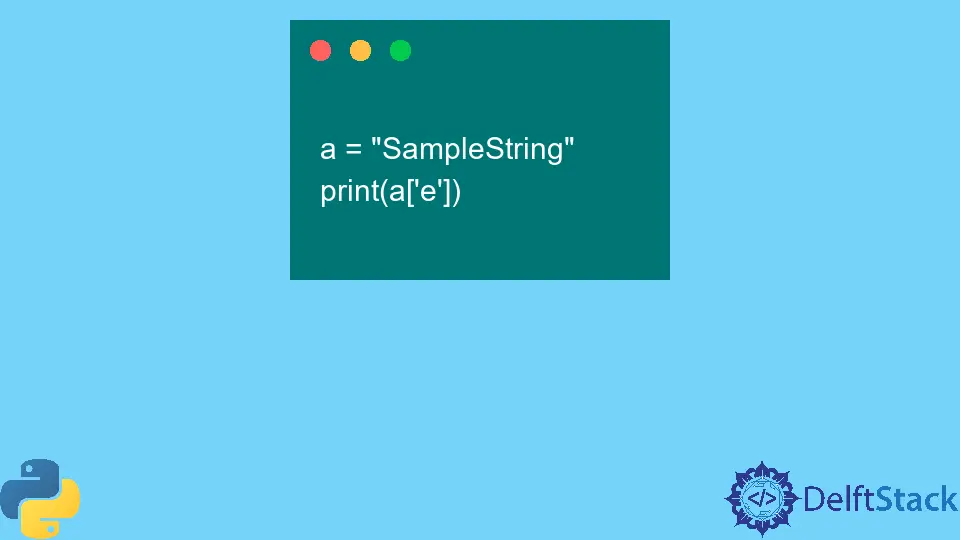
When working with Python, encountering errors can be frustrating, especially when they seem cryptic. One common error that developers face is the “string indices must be integers” error. This error typically arises when you try to access elements of a string or a list using a string key instead of an integer index.
In this tutorial, we will explore what causes this error, how to fix it, and provide practical examples to help you understand the solution better. Whether you’re a beginner or an experienced developer, this guide will equip you with the knowledge to resolve this issue and improve your coding skills.
Understanding the Error
Before diving into solutions, it’s essential to understand why this error occurs. The “string indices must be integers” error happens when you attempt to access a string using a string index instead of an integer. For example, if you have a string and try to access a character using another string as an index, Python raises this error.
Here’s a simple illustration of the error:
my_string = "Hello, World!"
print(my_string["0"])
Output:
TypeError: string indices must be integers
In this code snippet, we mistakenly used a string “0” as an index, which leads to the error. To fix this, we need to use an integer index.
Solution Method 1: Using Integer Indices
The most straightforward way to fix the “string indices must be integers” error is to ensure that you’re using integer indices when accessing characters in a string. Python strings are indexed starting from 0, so the first character is at index 0, the second at index 1, and so on.
Here’s how you can correctly access the first character of a string:
my_string = "Hello, World!"
print(my_string[0])
Output:
H
In this corrected code, we used the integer index 0 to access the first character of the string “Hello, World!”. This resolves the error, allowing us to retrieve the character without any issues.
Using integer indices is crucial when dealing with strings, lists, or any other indexed data structures in Python. Always remember that indices must be integers, and if you’re unsure about the type of your index, you can use the type()
function to check.
Solution Method 2: Using Dictionaries Correctly
Another common scenario where the “string indices must be integers” error occurs is when working with dictionaries. If you mistakenly try to access a dictionary value using a string key without the correct syntax, you might encounter this error.
Let’s take a look at an example:
my_dict = {"name": "Alice", "age": 30}
print(my_dict["name"])
Output:
Alice
In this example, we correctly accessed the value associated with the key “name”. However, if we mistakenly tried to use an integer index instead of the string key, we would encounter the error:
print(my_dict[0])
Output:
KeyError: 0
To avoid this error, always ensure that you’re using the correct key type when accessing dictionary values. If you need to iterate over the keys or values, consider using methods like .keys()
or .values()
to avoid confusion.
Solution Method 3: Debugging the Code
Sometimes, the “string indices must be integers” error can stem from more complex code logic. In such cases, debugging is essential. You can use print statements or a debugger to track down where the error is occurring.
For instance, consider the following code:
data = [{"name": "Alice"}, {"name": "Bob"}]
for item in data:
print(item["name"])
Output:
Alice
Bob
This code correctly accesses the “name” key in each dictionary within the list. However, if you accidentally modify the structure of data
to include a string instead of a dictionary, you might encounter the error:
data = [{"name": "Alice"}, "This is a string", {"name": "Bob"}]
for item in data:
print(item["name"])
Output:
TypeError: string indices must be integers
To debug this, you can add a check to ensure that item
is indeed a dictionary before trying to access it:
data = [{"name": "Alice"}, "This is a string", {"name": "Bob"}]
for item in data:
if isinstance(item, dict):
print(item["name"])
else:
print("Not a dictionary")
Output:
Alice
Not a dictionary
Bob
This approach not only prevents the error but also helps you understand the data structure you’re working with. Debugging is a vital skill in programming, and learning how to effectively troubleshoot errors will make you a more proficient developer.
Conclusion
The “string indices must be integers” error in Python can be easily fixed once you understand its causes. By ensuring that you use integer indices when accessing strings and correctly handling dictionary keys, you can avoid this common pitfall. Debugging your code is also a valuable strategy for identifying and resolving errors. With the knowledge gained from this tutorial, you can confidently tackle this error and enhance your Python coding skills. Remember, practice makes perfect, so keep coding and exploring!
FAQ
-
What causes the string indices must be integers error?
This error occurs when you try to access a string using a string index instead of an integer index. -
How can I avoid this error when working with dictionaries?
Always use the correct key type when accessing dictionary values. Ensure that you’re using string keys for dictionary lookups. -
Can debugging help me fix this error?
Yes, debugging can help you identify where the error is occurring in your code and allow you to correct it effectively. -
What should I do if I encounter this error in a loop?
Check the type of the variable you are iterating over to ensure it is what you expect, and use appropriate checks before accessing elements. -
Is this error specific to Python?
While similar errors can occur in other programming languages, the specific message “string indices must be integers” is unique to Python.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python