How to Get Set Intersection in Python
-
Set Intersection With the
intersection()
Function in Python -
Get Set Intersection With the
&
Operator in Python -
Set Intersection With the
intersection_update()
Function in Python
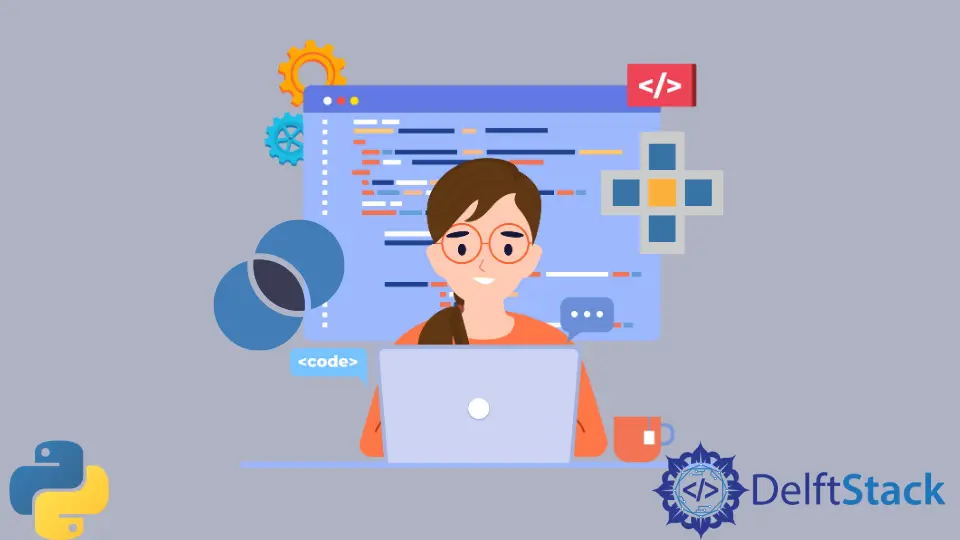
This tutorial will discuss different methods that can be used to get set intersections in Python.
Set Intersection With the intersection()
Function in Python
In the set intersection, we select all the common elements inside two or more sets. In Python, we have the intersection()
function that can be used to perform set intersection. The intersection()
function takes one or more sets as an input and returns their intersection in the form of another set. The following code snippet shows us how to perform set intersection on two sets with the intersection()
function.
set1 = {1, 2, 3, 4}
set2 = {2, 4, 6, 8}
set3 = set1.intersection(set2)
print(set3)
Output:
{2, 4}
We performed set intersection on set1
and set2
with the intersection()
function in the above code. We can also use the same intersection()
function to perform set intersection on more than two sets.
set1 = {1, 2, 3, 4}
set2 = {2, 4, 6, 8}
set3 = {2, 3, 5, 7}
set4 = set1.intersection(set2, set3)
print(set4)
Output:
{2}
We performed set intersection on set1
, set2
, and set3
with the intersection()
function in the above code. The result of this operation was stored inside the set4
and displayed to the user. The output shows 2
because it is the only element present in all three sets.
Get Set Intersection With the &
Operator in Python
The &
operator can also be used to perform set interesection in Python. The &
operator returns the common in both of its operands. The return type of the &
operator is a set. See the following code snippet.
set1 = {1, 2, 3, 4}
set2 = {2, 4, 6, 8}
set3 = set1 & set2
print(set3)
Output:
{2, 4}
We performed set intersection on set1
and set2
with the &
operator in the above code. We can also use the same &
operator to perform set intersection on more than two sets.
set1 = {1, 2, 3, 4}
set2 = {2, 4, 6, 8}
set3 = {2, 3, 5, 7}
set4 = set1 & set2 & set3
print(set4)
Output:
{2}
We performed set intersection on set1
, set2
, and set3
with the &
operator in the above code. The result of this operation was stored inside the set4
and displayed to the user. The output shows 2
because it is the only element present in all three sets.
Set Intersection With the intersection_update()
Function in Python
In the previous two sections, we have noticed that we need to create a new set that stores all the resultant values to perform the set intersection operation. With the intersection_update()
function, we do not need to create a new set to store the results. Instead, the calling set is updated, and the resultant values are stored inside the calling set. The following code snippet shows us how to perform set intersection on two sets with the intersection_update()
function.
set1 = {1, 2, 3, 4}
set2 = {2, 4, 6, 8}
set1.intersection_update(set2)
print(set1)
Output:
{2, 4}
We performed set intersection on set1
and set2
with the intersection_update()
function in the above code. As discussed above, the resulting values were stored inside the calling set, which is set1
in our case. We can also use the same intersection_update()
function to perform set intersection on more than two sets. The following code snippet demonstrates how to perform set intersection on more than two sets with the intersection_update()
function.
set1 = {1, 2, 3, 4}
set2 = {2, 4, 6, 8}
set3 = {2, 3, 5, 7}
set1.intersection_update(set2, set3)
print(set1)
Output:
{2}
We performed set intersection on set1
, set2
, and set3
with the intersection_update()
function in the above code. The result of this operation was stored inside the set1
and displayed to the user. The output shows 2
because it is the only element present in all three sets.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn