How to Round Up a Number in Python
-
Use the
math.ceil()
Function to Round Up a Number in Python 2.x - Use Simple Arithmetic to Round Up a Number in Python
- Use Floor Division Operator to Round Up a Number in Python
-
Use the
numpy.ceil()
Method to Round Up a Number in Python
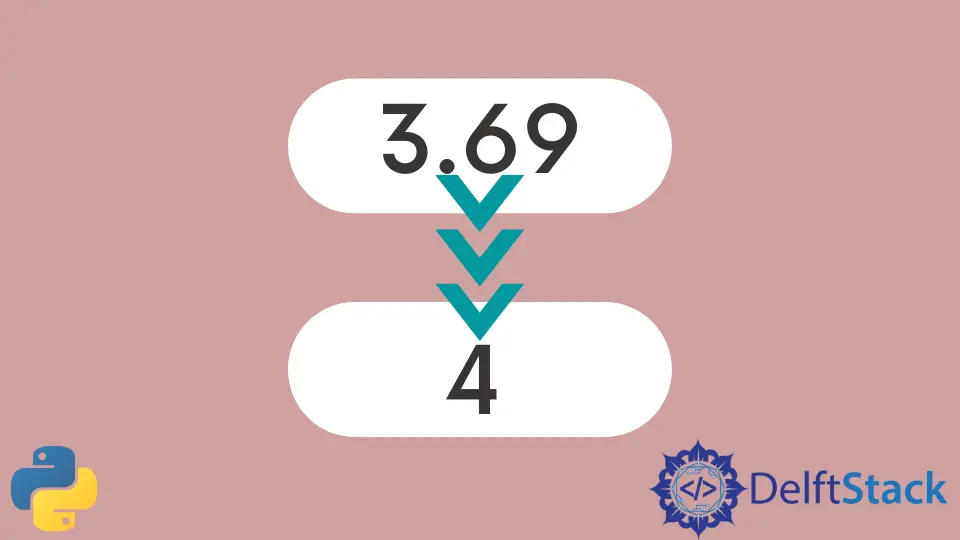
This tutorial explains different methods to explain the concept of rounding up a number. There are various ways to round up a number in the correct way. For example, a number can be rounded using the math
module provided by Python, using the NumPy
module, and so on. The tutorial will explain these different methods using example code snippets.
Use the math.ceil()
Function to Round Up a Number in Python 2.x
If you are using the Python 2.x version, you can use the math.ceil()
function to round up a number correctly. The ceil()
function is provided by the math
library of Python. So we need to import the math
library first. The ceil
function takes the number that needs to be rounded. It can take an expression and round the resulting number as per the results.
However, it should be noted that in Python 2.x, int/int
produces int
, and int/float
results in a float
. So we need to give one of the values in float
to the ceil
function to get accurate results. If both values of an expression in the ceil
function is an integer, it can produce wrong results.
An example code to illustrate the concept of how to use math.ceil()
to round up a number in Python 2.x is given below.
import math
print(math.ceil(27 / 4))
print(math.ceil(27.0 / 4))
Output:
6
7
Use Simple Arithmetic to Round Up a Number in Python
A number can also be rounded up by using simple arithmetic in Python. This method is applicable to all versions of Python. It casts the first expression into an integer
data type and adds 0 or 1 value based on the result of another expression. Other expression finds the modulus of the number with the same denominator and checks if it is greater than 0 or not. If the remainder is greater than 0, it adds one to the first expression, and if it is false, it adds 0 to the first expression.
An example code is given below to explain how to use simple arithmetic to round up a number in Python without importing the math
library.
n = 22
div = 5
print(int(n / div) + (n % div > 0))
Output:
5
Use Floor Division Operator to Round Up a Number in Python
The symbol for the floor division operator is //
. It works in the same way as a simple division operator, /
, but it also rounds the number down. So, It is usually used to round down the number in Python. However, we can modify its use to round up a number as well. We can do so by negating the answer by dividing negative numbers. It will save the cost of any import or use of float and any other conditions. It works with the integers only.
An example code is given below to elaborate on how to use the floor division operator to round up a number in Python.
n = 22
div = 5
answer = -(-n // div)
print(answer)
Output:
5
Similarly, we can round up a number by adding the denominator to the numerator and subtracting 1 from it. Then the whole expression will be floor divided with the denominator. It is a straightforward method that does not involve any floating points and external modules also. An example code of this method is given below.
numerator = 22
denominator = 5
answer = (numerator + denominator - 1) // denominator
print(answer)
Output:
5
Use the numpy.ceil()
Method to Round Up a Number in Python
Another method to round up a number is to use the numpy.ceil()
method. First, we need to import the NumPy
module in the script and then use the ceil()
method to round up a number. The return type of the ceil()
function is float, so even if the expression is in integers, the output will be in the float. The output can be explicitly cast to integer data type by explicitly casting it to be an integer.
An example code of this method to round up a number in Python is given below.
import numpy as nmp
n = 22
div = 5
answer = n / div
print(nmp.ceil(answer))
print(int(nmp.ceil(answer)))
Output:
5.0
5
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn