How to Remove Element From Set in Python
-
Remove Single Element From a Set With the
remove()
Function in Python -
Remove Single Element From a Set With the
discard()
Function in Python -
Remove Multiple Elements From a Set With the
-
Operator in Python -
Remove Multiple Elements From a Set With the
difference()
Function in Python -
Remove Multiple Elements From a Set With the
difference_update()
Function in Python
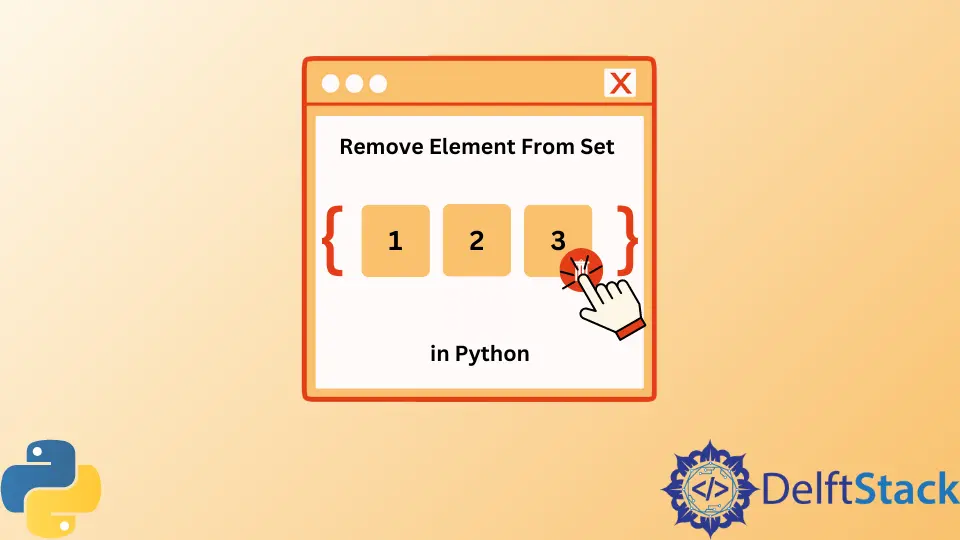
This tutorial will discuss different methods to remove elements from a set in Python.
Remove Single Element From a Set With the remove()
Function in Python
The set.remove()
function in Python is used with a set to remove a specific element from the calling set. The remove()
function takes the element to be removed as an argument and removes the specified element from the calling set. If the element is not found inside the calling set, the remove()
function raises the KeyError
exception. See the following code example.
set1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}
set1.remove(5)
print(set1)
Output:
{0, 1, 2, 3, 4, 6, 7, 8, 9}
We removed the element 5
from set1
with the remove(5)
function in the above code. The scenario where the specified value is not present in the calling set is shown in the coding example below.
set1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}
set1.remove(51)
print(set1)
Output:
KeyError Traceback (most recent call last)
<ipython-input-1-841e997b50f3> in <module>()
1 #remove()
2 set1 = {1,2,3,4,5,6,7,8,9,0}
----> 3 set1.remove(51)
4 print(set1)
KeyError: 51
We tried to remove the value 51
from set1
in the above code. As there is no value 51
present in set1
, the remove()
function raises a KeyError
.
Remove Single Element From a Set With the discard()
Function in Python
The discard()
function is very similar to the remove()
function. It can also be used to remove only a single element from a set in Python. The discard()
function takes the element to be removed as an input parameter and removes it from the calling set if the value is present in that particular set. The only difference is that the discard()
function does not raise a KeyError
if the value is not present in the calling set. The following code snippet shows us how to remove a single element from a set with the discard()
function.
set1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}
set1.discard(5)
print(set1)
Output:
{0, 1, 2, 3, 4, 6, 7, 8, 9}
We removed the value 5
from set1
with the discard()
function in the above code. As discussed above, if we try to pass a value to the discard()
function that is not present in the set1
, the code is executed successfully, and the interpreter raises no exception. This phenomenon is demonstrated in the following coding example.
set1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}
set1.discard(51)
print(set1)
Output:
{0, 1, 2, 3, 4, 5, 6, 7, 8, 9}
We tried to remove 51
from set1
with the discard()
function in the above code. The code is executed successfully because the specified value does not exist in set1
, and no element is removed from set1
.
Remove Multiple Elements From a Set With the -
Operator in Python
We can use the -
operator is used to perform the set difference operation in Python. The -
operator removes all the elements of the right set from the left set and returns the result in the form of another set. This method does not raise any exceptions if the elements of the left set are not present in the right set and returns the right set as its result. The following code snippet shows us how to remove multiple elements from a set with the -
operator.
set1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}
set2 = {2, 4, 6, 8, 0}
set1 = set1 - set2
print(set1)
Output:
{1, 3, 5, 7, 9}
We removed all the elements of set2
from set1
with the -
operator and stored the resultant set in set3
in the above code. The following code example demonstrates the scenario where both set1
and set2
have unique values.
set1 = {1, 3, 5, 7, 9}
set2 = {2, 4, 6, 8, 0}
set1 = set1 - set2
print(set1)
Output:
{1, 3, 5, 7, 9}
As discussed earlier, if all the elements of the right-sided set are not present in the left-sided set, the code executes successfully, and the -
operator returns the left-sided set as the resultant set.
Remove Multiple Elements From a Set With the difference()
Function in Python
In set difference, we remove all the elements of one set from another set. In Python, the set.difference()
function is used for performing set difference operation. The working of this difference()
function is the same as the -
operator. It takes the set to be removed as an input parameter, removes all of its elements from the calling set, and returns the results in the form of another set. See the following code snippet.
set1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}
set2 = {2, 4, 6, 8, 0}
set1 = set1.difference(set2)
print(set1)
Output:
{1, 3, 5, 7, 9}
We removed all the elements of set2
from set1
with the difference()
function and stored the results inside set3
in the above code. The scenario for the difference()
function where all the values of the input set are not present in the calling set is the same as the -
operator.
Remove Multiple Elements From a Set With the difference_update()
Function in Python
In the previous two sections, we have discussed how to remove multiple elements from a set, and both approaches involve a third set where we need to store the results. This approach does not require a new set to store the results. It updates our calling set and stores the results inside the calling set. The difference_update()
function takes the set whose elements need to be removed from the calling set as input parameter and removes all the common elements from the calling set.
set1 = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0}
set2 = {2, 4, 6, 8, 0}
set1.difference_update(set2)
print(set1)
Output:
{1, 3, 5, 7, 9}
We removed all the elements of set2
from set1
and updated set1
with the resultant set by using the difference_update()
function in the above code. Unlike the previous two methods, there was no need to create a new set just to store the results. Instead, the result was automatically inserted into set1
.
The difference_update()
function is equivalent to the -=
operator in Python.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn