How to Create Ordered Set in Python
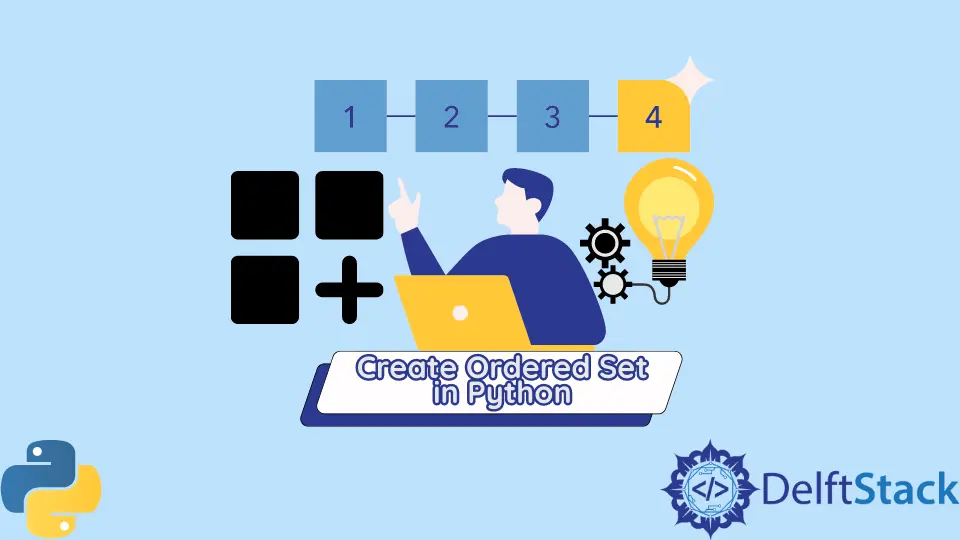
This tutorial will discuss the OrderedSet
class in Python.
Create Ordered Sets With the OrderedSet
Class in Python
To start, let’s first define what a set means in Python. It’s a well-defined collection of distinct objects that are somehow related. This definition does not contain any order. So by definition, a set has no order in it.
However, if such a scenario arises that we have to preserve the original order of the set elements, we can use the OrderedSet
class. To use the OrderedSet
class, we have to install the ordered-set
package first on our device with the Python package manager. The command to install the ordered-set
package is given below.
pip install ordered-set
Now, we can create a set that preserves the order of each set element. This process is demonstrated in the following program.
from ordered_set import OrderedSet
setABC = OrderedSet(["A", "B", "C"])
print(setABC)
Output:
OrderedSet(['A', 'B', 'C'])
We created an ordered set and displayed the value at each index in order with the OrderedSet
class in the code above. This OrderedSet
is a mutable data structure that is a hybrid between the list and set data structures. So, we have to initialize this set with a list; this way, the set keeps the index of each element.
To simplify the indexing, the add()
function returns the index of the new element added into the set; this is shown in the following process below.
print(setABC.add("D"))
Output:
3
We added another element to our setABC
and displayed the value returned by the add()
function in the code above. We can also get the index of a specific element with the index()
function; this is shown in the following code snippet.
print(setABC.index("C"))
Output:
2
We retrieved the index of the element C
inside the setABC
set with the index()
function in the code above. We can also perform the usual operations of sets like union, intersection, and difference on these ordered sets with the |
, &
, and -
operators, respectively.
The following code example demonstrates how we can perform union on an ordered set.
from ordered_set import OrderedSet
setABC = OrderedSet(["A", "B", "C", "D"])
setDEF = OrderedSet(["D", "E", "F", "G"])
solutionSet = setABC | setDEF
print(solutionSet)
Output:
OrderedSet(['A', 'B', 'C', 'D', 'E', 'F', 'G'])
We calculated the union of the sets setABC
and setDEF
with the union operator |
in the code above. The following program below shows us how we can perform intersection on an ordered set.
from ordered_set import OrderedSet
setABC = OrderedSet(["A", "B", "C", "D"])
setDEF = OrderedSet(["D", "E", "F", "G"])
solutionSet = setABC & setDEF
print(solutionSet)
Output:
OrderedSet(['D'])
We calculated the intersection of the sets setABC
and setDEF
with the intersection operator &
in the code above. The following code example below shows us how we can calculate the difference between two ordered sets.
from ordered_set import OrderedSet
setABC = OrderedSet(["A", "B", "C", "D"])
setDEF = OrderedSet(["D", "E", "F", "G"])
solutionSet = setABC - setDEF
print(solutionSet)
Output:
OrderedSet(['A', 'B', 'C'])
We calculated the difference of the sets setABC
and setDEF
with the difference operator -
in the code above.
By default, Python does not support ordered sets. Still, we can install an external package, ordered-set
, which gives us the functionality of creating ordered sets. This process is supported on Python version 2.6 and higher.
These ordered sets are a hybrid of the original lists and sets in Python. So, we have to initialize these sets with a list. These new sets provide us all the usual functionalities of sets like union, intersection, and difference.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn