How to Fix Operands Could Not Be Broadcast Together With Shapes Error in Python
- Understanding the Broadcasting Mechanism
- Method 1: Reshape Your Arrays
- Method 2: Use np.newaxis for Dimension Expansion
- Method 3: Adjust Array Dimensions with Broadcasting Rules
- Conclusion
- FAQ
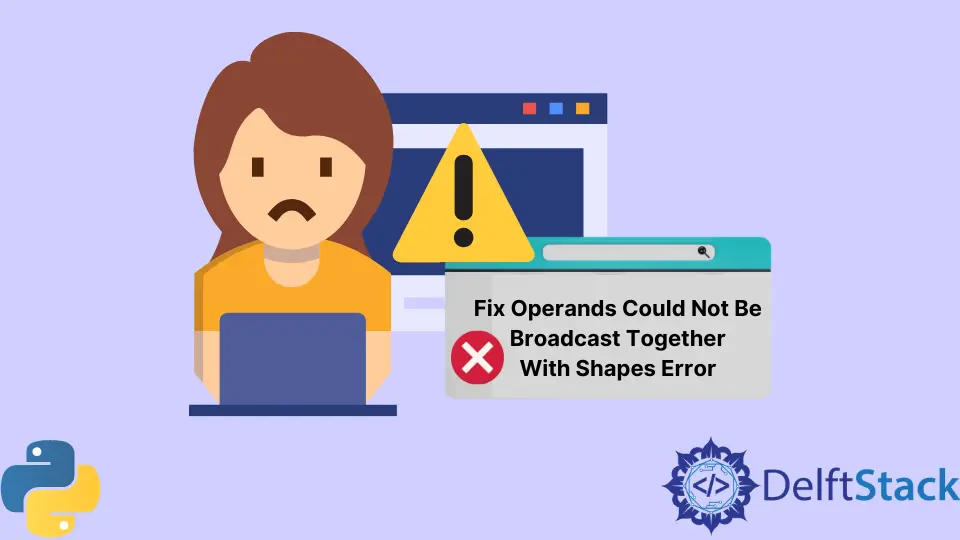
When working with numerical data in Python, especially using libraries like NumPy, you might encounter the infamous “operands could not be broadcast together with shapes” error. This error can be frustrating, especially when you’re trying to perform operations on arrays of different shapes. Understanding how broadcasting works in Python is crucial for resolving this issue.
In this article, we will explore common causes of this error and provide practical solutions to fix it. Whether you’re a beginner or an experienced programmer, you’ll find valuable insights that can help you troubleshoot and overcome this broadcasting error effectively.
Understanding the Broadcasting Mechanism
Before diving into solutions, it’s essential to grasp what broadcasting means in the context of NumPy. Broadcasting allows NumPy to perform arithmetic operations on arrays of different shapes. For instance, if you have a 2D array and a 1D array, NumPy can stretch the 1D array across the 2D array to perform element-wise operations. However, if the shapes are incompatible, you’ll face the broadcasting error.
For example, consider two arrays:
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.array([1, 2])
Attempting to add these two arrays will raise an error because their shapes cannot be aligned for broadcasting.
Output:
ValueError: operands could not be broadcast together with shapes (2,3) (2,)
The key to resolving this issue lies in ensuring that the shapes of the arrays are compatible for broadcasting. Let’s explore some methods to fix this error.
Method 1: Reshape Your Arrays
One of the most straightforward ways to fix the broadcasting error is to reshape the arrays involved in the operation. The reshape
method in NumPy allows you to change the dimensions of an array without changing its data. This can help align the shapes of the arrays for broadcasting.
Here’s an example:
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.array([1, 2]).reshape(2, 1)
result = a + b
print(result)
Output:
[[2 3 4]
[6 7 8]]
In this code, we reshape the 1D array b
from shape (2,)
to (2, 1)
, making it compatible with the shape of a
. Now, NumPy can successfully perform the addition operation, and the result is a new array where each element of b
is added to the corresponding row of a
.
Reshaping is a powerful technique that can often resolve broadcasting issues. However, it’s crucial to ensure that the total number of elements remains the same after reshaping. Otherwise, you may encounter a different error.
Method 2: Use np.newaxis for Dimension Expansion
Another effective method to fix the broadcasting error is to use np.newaxis
, which allows you to add a new axis to your array. This is particularly useful when you want to convert a 1D array into a 2D array, making it compatible with other arrays during operations.
Here’s how you can use np.newaxis
:
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.array([1, 2])[np.newaxis, :]
result = a + b
print(result)
Output:
[[2 4 6]
[5 7 9]]
In this example, we added a new axis to the array b
, transforming it from shape (2,)
to (1, 2)
. This change allows NumPy to broadcast the array correctly across the rows of a
, resulting in a successful addition operation.
Using np.newaxis
is a clean and efficient way to manage array shapes, especially when dealing with multiple dimensions. It’s a handy tool in your NumPy toolkit for preventing broadcasting errors.
Method 3: Adjust Array Dimensions with Broadcasting Rules
Sometimes, the solution to the broadcasting error lies in understanding and applying the broadcasting rules effectively. NumPy follows specific rules to determine how two arrays can be broadcast together. If you can align the dimensions of your arrays according to these rules, you can often avoid errors.
The rules are as follows:
- If the arrays have a different number of dimensions, prepend the shape of the smaller-dimensional array with ones until both shapes are the same.
- The sizes of the dimensions must either be the same or one of them must be one.
For example, consider the following arrays:
import numpy as np
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.array([[1], [2]])
result = a + b
print(result)
Output:
[[2 3 4]
[6 7 8]]
Here, b
has the shape (2, 1)
, and a
has the shape (2, 3)
. When we attempt to add them, NumPy automatically broadcasts b
across the columns of a
, resulting in a successful addition.
Understanding these rules can help you manipulate your arrays’ shapes more effectively, allowing for successful broadcasting without needing to reshape or expand dimensions explicitly.
Conclusion
Encountering the “operands could not be broadcast together with shapes” error in Python can be a common hurdle when working with numerical data. However, by understanding broadcasting and employing techniques such as reshaping arrays, using np.newaxis
, and applying broadcasting rules, you can effectively troubleshoot and resolve these issues. With practice, you’ll find that these methods become second nature, enabling you to work more efficiently with NumPy and other numerical libraries in Python.
FAQ
-
What is broadcasting in NumPy?
Broadcasting is a mechanism that allows NumPy to perform arithmetic operations on arrays of different shapes by stretching the smaller array across the larger one. -
How can I reshape an array in NumPy?
You can reshape an array using thereshape
method, which allows you to specify the new dimensions while keeping the total number of elements constant.
-
What does np.newaxis do in NumPy?
np.newaxis
is used to increase the dimensions of an array by one, allowing for easier broadcasting during arithmetic operations. -
What are the broadcasting rules in NumPy?
The broadcasting rules state that if the dimensions of arrays are different, the smaller array’s shape is padded with ones until both shapes are the same, and then the sizes must be either the same or one of them must be one. -
Can I perform broadcasting with more than two arrays?
Yes, broadcasting can be applied to multiple arrays as long as they all adhere to the broadcasting rules.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python