How to Find Number of Digits in a Number in Python
-
Find the Number of Digits Inside a Number With the
math.log10()
Function in Python -
Find the Number of Digits Inside a Number With the
len()
Function in Python -
Find the Number of Digits Inside a Number Using a
while
Loop in Python - Find the Number of Digits Inside a Number Using Recursion in Python
- Conclusion
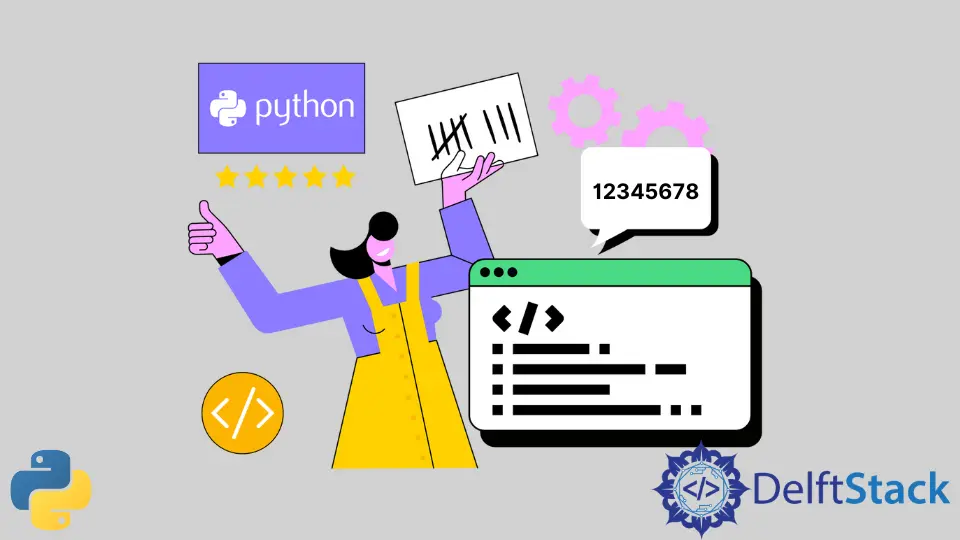
In this tutorial, we’ll explore multiple methods to count the number of digits inside a number. These methods give versatility and address various scenarios requiring digit counting, assuring reliable results.
Find the Number of Digits Inside a Number With the math.log10()
Function in Python
The math.log10()
function inside the math
module of Python is used to find the log of base 10
for any specified number. As the integers are also in base 10
, we can get the number of digits inside the specified integer with this method.
Basic Syntax:
math.log10(x)
Parameter:
x
: The number for which you want to find the base-10 logarithm. It can be a positive floating-point number, integer, or any numeric value.
In the following code, we are determining the number of digits in a given number, which is assigned to the variable n
with the value of -10
. We start by importing the math
module to make use of the log10()
function, which allows us to find the logarithm base 10
of a number.
Then, we check different conditions based on the value of n
. If n
is greater than 0
, we calculate the number of digits using math.log10(n)
and add 1
to the result, and this accounts for positive numbers.
In the case where n
equals 0
, we directly set the number of digits to 1
since 0
is a single-digit number.
Finally, if n
is less than 0
, we calculate the number of digits for the absolute value of n
using math.log10(-n)
and add 2
to account for the minus sign, and this is necessary for negative numbers.
import math
n = -10
if n > 0:
digits = int(math.log10(n)) + 1
elif n == 0:
digits = 1
elif n < 0:
digits = int(math.log10(-n)) + 2
print(digits)
Output:
3
The output of this code, when n
is -10
, will be 3
. This is because the code follows the elif
condition for negative numbers and correctly calculates the number of digits as 3
, taking into account the minus sign.
This method is great to use if we want to determine the number of digits inside an integer. However, it doesn’t work with decimal numbers or any numbers with a floating decimal point.
Find the Number of Digits Inside a Number With the len()
Function in Python
The len()
function is a Python built-in function used to calculate the number of characters inside a string variable. The function len()
takes a string as an input parameter and returns the number of characters inside that string.
To calculate the number of digits inside a number using the len()
function, we first have to convert that number into a string with the str()
function. The str()
function is also a built-in function in Python used to convert objects of different types into a string variable.
Basic Syntax:
len(s)
Parameter:
s
: The sequence (string, list, tuple, or any iterable) for which you want to find the length.
In the following code, we are tasked with finding the number of digits in a given number, which is assigned to the variable n
, with the value of -100.90
. To achieve this, we start by converting the number to a string using str(n)
.
This transformation allows us to treat the number as a sequence of characters. We then apply the len()
function to this string, which calculates the length of the string, effectively counting the number of characters. In this case, the output is 6
.
n = -100.90
digits = len(str(n))
print(digits)
Output:
6
This result accounts for the minus sign, the two integer digits (1
and 0
), the decimal point, and the two decimal digits (9
and 0
), totaling six characters in the string representation of -100.90
.
The len()
method is far superior to the math.log10()
method for finding the number of digits inside a decimal number in Python. The reason is the len()
method is clear and concise and also handles floating decimal points, unlike the math.log10()
method, which is unnecessarily complex and doesn’t handle floating decimal points.
Find the Number of Digits Inside a Number Using a while
Loop in Python
Finding the number of digits inside a number using a while
loop in Python is a common programming task.
This method involves iteratively dividing the number by 10
while keeping count of the divisions with each iteration. By repeatedly dividing the number until it becomes 0
, we can identify the number of digits in the original number.
In the following code, we aim to determine the number of digits in a given number, which is assigned to the variable n
with a value of -100
. We begin by checking a special case: if n
is equal to 0
, we directly set the digits variable to 1
since 0
is a single-digit number.
However, since the value of n
is not 0
, we initialize the digits variable to 0
and then proceed to work with the absolute value of n
, which ensures we handle negative numbers correctly. We utilize a while
loop to iteratively divide n by 10
.
In each iteration, we increment the digits count by 1
, effectively counting the number of digits in the number. This process continues until the entire number is broken down to 0
, at which point the loop terminates.
n = -100
if n == 0:
digits = 1
else:
digits = 0
n = abs(n)
while n > 0:
digits += 1
n //= 10
print(digits)
Output:
3
As a result, the variable digits
holds the count of digits in the original number. In this specific case, when n
is -100
, we find that there are 3 digits (1
, 0
, and 0
), and thus, the output is 3
.
Find the Number of Digits Inside a Number Using Recursion in Python
In Python, we can use recursion to find the number of digits inside a number by creating a recursive function that breaks down the number into its digits one by one until it reaches the base case. Because a single-digit number has only one digit, the base case is often when the number becomes less than ten.
In the following code, we define a recursive function called count_digits_recursive
to determine the number of digits in a given number, which is initially set to n
with a value of -10
. The function first ensures that the number is non-negative by taking its absolute value if it’s negative.
Then, we check whether the absolute value of n
is less than 10
, serving as the base case for the recursion. When n
becomes a single-digit number, the function returns 1
since a single-digit number contains only one digit.
In the recursive case, where n
has more than one digit, the function divides n
by 10
, effectively removing the rightmost digit. It then makes a recursive call to count the remaining part of the number while adding 1
to accumulate the digit count.
When we invoke the function with n
set to -10
, it follows these steps and returns an output of 2
.
def count_digits_recursive(n):
if n < 0:
n = abs(n)
if n < 10:
return 1
return 1 + count_digits_recursive(n // 10)
n = -10
digits = count_digits_recursive(n)
print(digits)
Output:
2
The output correctly represents the number of digits in the original number -10
. This is because -10
is a two-digit number, and the recursive function accurately calculates the digit count, providing a result of 2
.
Conclusion
We’ve explored an array of methods to count the number of digits in a number, with each technique offering unique advantages. The math.log10()
function provides a mathematical foundation, accommodating both positive and negative integers.
On the other hand, the len()
function simplifies digit counting by treating numbers as strings, including those with decimal points. Our exploration also includes the use of a while
loop, which dynamically calculates digit counts, adding versatility to our toolkit.
Lastly, the power of recursion allows for efficient and elegant solutions to complex digit-counting challenges.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn