How to Stringify JSON in Python
-
Difference Between JavaScript’s
JSON.stringify()
and Python’sjson.dumps()
in Handling Lists -
Make the Python
json.dumps()
Function Work Like the JavaScriptJSON.stringify()
Function - Conclusion
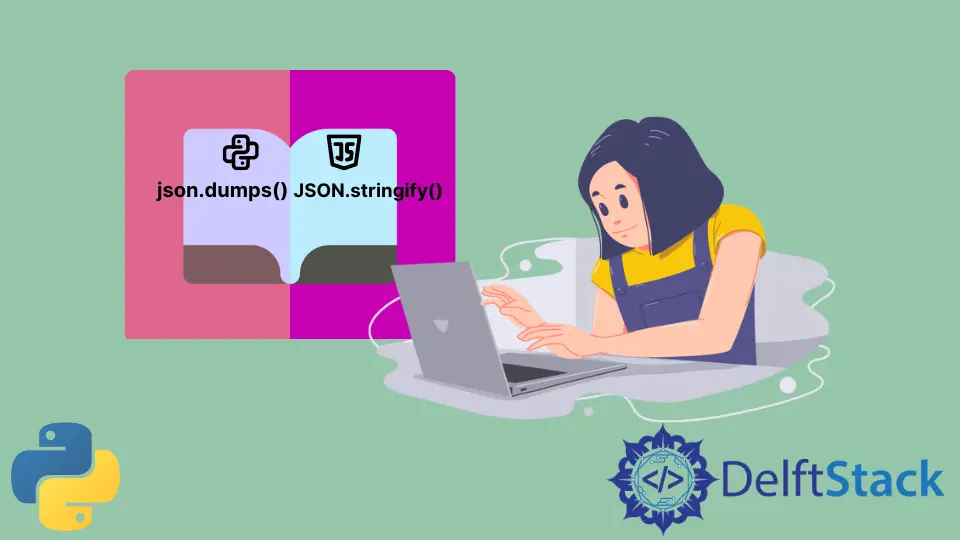
JSON, or JavaScript Object Notation, is like the common language computers use to share information on the web. It is used when sending data between web servers and clients.
To make this happen, a process called serialization takes place, which is like turning complicated data into a simple text form. In the programming languages JavaScript and Python, there are specific functions that handle this serialization task.
In JavaScript, there’s a function called JSON.stringify()
, and in Python, it’s json.dumps()
. These functions help convert data structures into a format that can be easily shared and understood.
Difference Between JavaScript’s JSON.stringify()
and Python’s json.dumps()
in Handling Lists
In the realm of data serialization, the JavaScript JSON.stringify()
function and its Python counterpart, json.dumps()
, play crucial roles in converting complex data structures into a string representation. One notable distinction between the two lies in their treatment of whitespace when applied to lists.
The JavaScript JSON.stringify()
function, by default, removes all whitespaces from the resulting JSON string. This behavior is particularly useful for minimizing data payload and improving transmission efficiency.
Sample JavaScript code:
var arr = [7, 8, 6];
JSON.stringify(arr);
Output:
[7,8,6]
The example code above initialized an array and passed it to the JSON.stringify()
function. The output shows that the function removed all the white spaces between the array elements.
In contrast to JavaScript JSON.stringify()
function, the Python json.dumps()
function retains whitespace by default when dealing with lists. A working implementation of this function is given in the coding example below.
Sample Python code:
import json
arr = [7, 8, 6]
print(json.dumps(arr))
Output:
[7, 8, 6]
The code above initialized a list and passed it to the json.dumps()
function. The output shows that the function keeps one white space between all the list elements.
Make the Python json.dumps()
Function Work Like the JavaScript JSON.stringify()
Function
To align the Python json.dumps()
function more closely with the JavaScript JSON.stringify()
function, developers often need to customize the serialization process. Fortunately, the json.dumps()
function in Python provides a parameter called separators
that allows users to specify custom string delimiters for different parts of the JSON representation.
By setting separators
to a tuple containing an empty string for the item separator, Python developers can achieve a similar whitespace-free output as the JavaScript counterpart. This modification effectively compresses the JSON representation of a list, making it similar to the compact, whitespace-stripped result produced by JSON.stringify()
in JavaScript.
For a working demonstration of this, take a look at the code below:
import json
arr = [7, 8, 6]
print(json.dumps(arr, separators=(",", ":")))
This Python code uses the json
module to convert a Python list, arr = [7, 8, 6]
, into a JSON-formatted string. The json.dumps()
function is employed for this conversion. The noteworthy aspect is the use of the separators
parameter within the function.
In simple terms, the separators=(",", ":")
part instructs the json.dumps()
function to use a comma (,
) as the item separator and a colon (:
) as the key-value pair separator. This customization eliminates unnecessary whitespaces from the resulting JSON string, making it more concise and similar to the output produced by the JavaScript JSON.stringify()
function.
Output:
[7,8,6]
In the output above, the numbers 7
, 8
, and 6
are enclosed within square brackets ([]
), indicating a JSON array. The absence of spaces between the numbers and the use of commas as separators aligns with the desired outcome of removing whitespaces.
Conclusion
In summary, Python’s json.dumps()
and JavaScript’s JSON.stringify()
initially treat whitespaces in lists differently.
Despite this, Python developers have a useful tool at their disposal – the separators
parameter. By applying this parameter, they can make the two functions behave similarly.
This adjustment not only brings their outputs in line but also empowers developers to customize how lists appear in strings, catering to their specific requirements and preferences. It’s a handy trick for achieving consistency and flexibility in handling JSON-like data structures.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn