How to Generate a Random Prime Number in Python
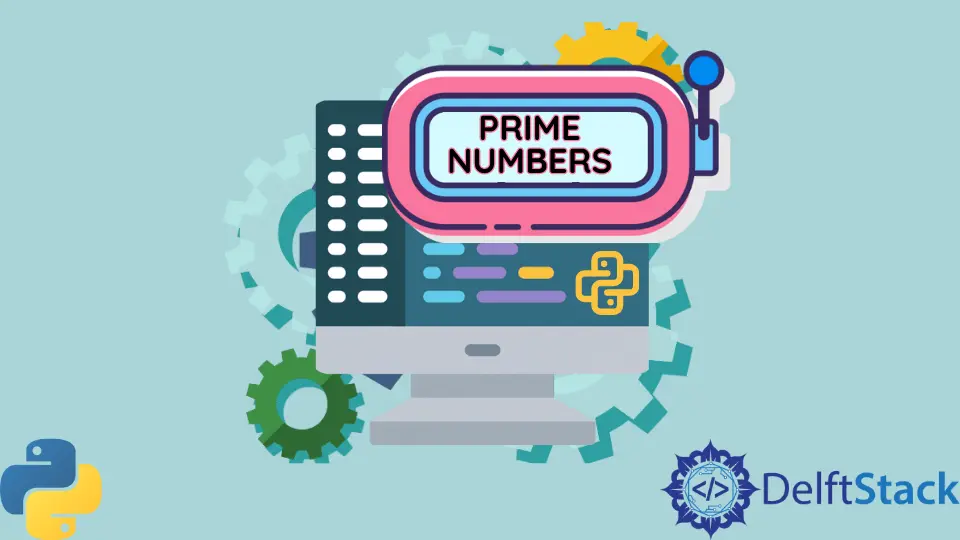
This tutorial demonstrates ways to generate and output any random prime number in Python.
Prime numbers are very useful constants used in programming, especially in cryptography. The use of prime numbers is vital to encryption and hashing as they can be used to encrypt and hash sensitive data and prevent them from being haphazardly decrypted.
Create a Function to Generate a Random Prime Number With a Given Range
Prime numbers only have 2 factors (factors are numbers that can divide another number and result to a whole number). Prime numbers are only divisible by 1 and themselves.
It is technically impossible to generate a random prime number ranging from 0 to infinity since this will take a lot of processing power to keep it up until huge numbers.
Given that, a range of numbers would need to be included as parameters to gather prime numbers within a set range.
The first thing to do is create a function to gather all the prime numbers within the given range into a list. For this function, loop all the numbers within the range and check if the only divisible numbers are 1 and itself.
def primesInRange(x, y):
prime_list = []
for n in range(x, y):
isPrime = True
for num in range(2, n):
if n % num == 0:
isPrime = False
if isPrime:
prime_list.append(n)
return prime_list
print(primesInRange(100, 250))
The output will print all the prime numbers from the given range 100 until 250.
Output:
[101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241]
The next step is to generate a random number from the given range of prime numbers returned by the function primesInRange()
.
Under the Python random
module, it has a function named choice()
that chooses a random element from a given iterable or sequence.
Given the function primesInRange()
implemented above, catch the return with a list variable and choose a random prime number within the list using random.choice()
. Don’t forget to import the random
module before using the choice()
function.
import random
prime_list = primesInRange(100, 250)
randomPrime = random.choice(prime_list)
print("Generated random prime number: ", randomPrime)
Sample randomized Output:
Generated random prime number: 191
This is the full source code of this solution:
import random
def primesInRange(x, y):
prime_list = []
for n in range(x, y):
isPrime = True
for num in range(2, n):
if n % num == 0:
isPrime = False
if isPrime:
prime_list.append(n)
return prime_list
prime_list = primesInRange(100, 250)
randomPrime = random.choice(prime_list)
print("Generated random prime number: ", randomPrime)
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn