How to Find String in File using Python
-
Use the File
readlines()
Method to Find a String in a File in Python -
Use the File
read()
Method to Search a String in a File in Python -
Use
find
Method to Search a String in a File in Python -
Use
mmap
Module to Search a String in a File in Python
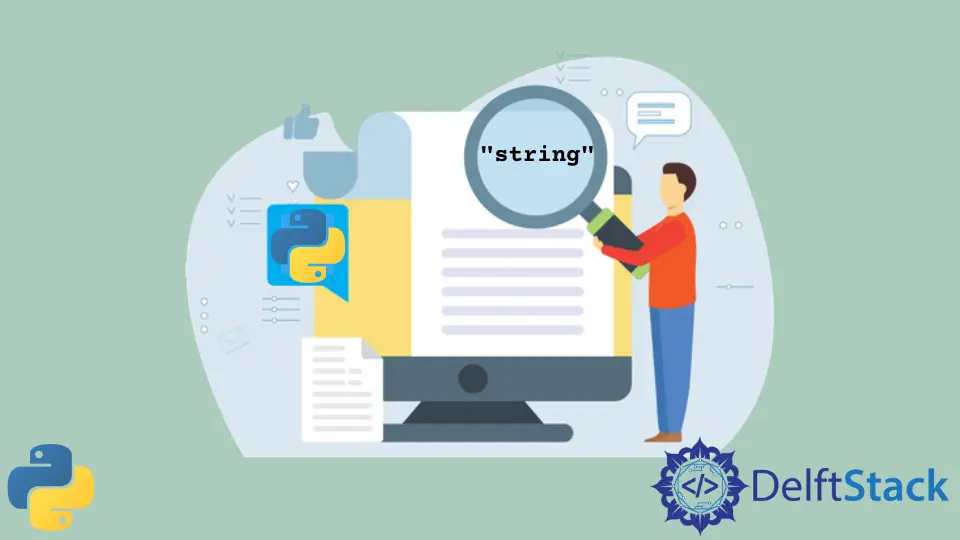
The tutorial explains how to find a specific string in a text file in Python.
Use the File readlines()
Method to Find a String in a File in Python
Pyton file readlines()
method returns the file content split to a list by the new line. We can use the for
loop to iterate through the list and use the in
operator to check whether the string is in the line in every iteration.
If the string is found in the line, it returns True
and breaks the loop. If the string is not found after iterating all lines, it returns False
eventually.
An example code for this approach is given below:
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
def check_string():
with open("temp.txt") as temp_f:
datafile = temp_f.readlines()
for line in datafile:
if "blabla" in line:
return True # The string is found
return False # The string does not exist in the file
if check_string():
print("True")
else:
print("False")
Output:
True
Use the File read()
Method to Search a String in a File in Python
The file read()
method returns the content of the file as a whole string. Then we can use the in
operator to check whether the string is in the returned string.
An example code is given below:
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
if "blabla" in f.read():
print("True")
Output:
True
Use find
Method to Search a String in a File in Python
A simple find
method can be used with the read()
method to find the string in the file. The find
method is passed the required string. It returns 0
if the string is found and -1
if the string is not found.
An example code is given below.
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
print(open("temp.txt", "r").read().find("blablAa"))
Output:
-1
Use mmap
Module to Search a String in a File in Python
The mmap
module can also be used to find a string in a file in Python and can improve the performance if the file size is relatively big. The mmap.mmap()
method creates a string-like object in Python 2 that checks the implicit file only and does not read the whole file.
An example code in Python 2 is given below:
# python 2
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find("blabla") != -1:
print("True")
Output:
True
However, in Python3 and above, mmap
doesn’t behave like the string-like object but creates a bytearray
object. So the find
method looks for bytes and not for strings.
An example code for this is given below:
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find(b"blabla") != -1:
print("True")
Output:
True
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python