Python 在檔案中查詢字串
-
在 Python 中使用檔案
readlines()
方法查詢檔案中的字串 -
在 Python 中使用檔案
read()
方法搜尋檔案中的字串 -
在 Python 中使用
find
方法搜尋檔案中的字串 -
在 Python 中使用
mmap
模組搜尋檔案中的字串
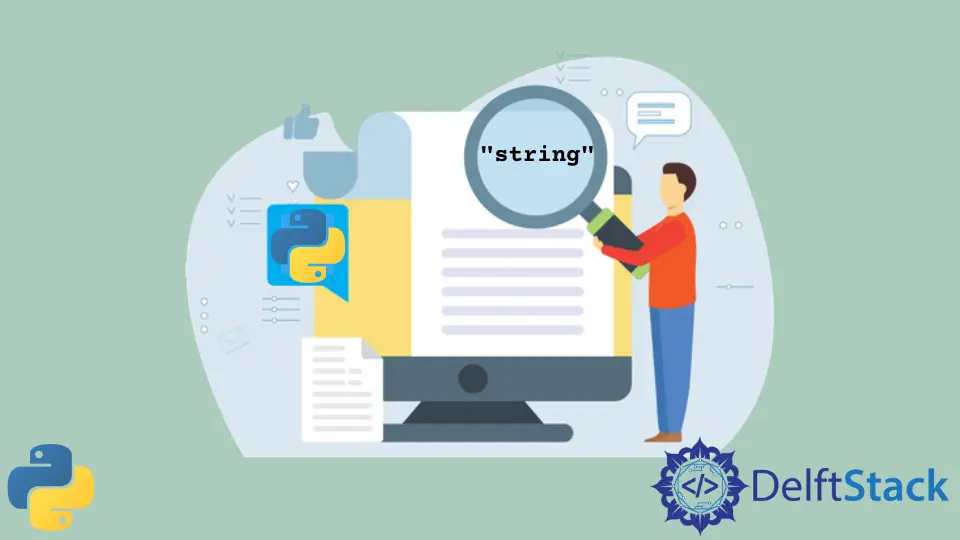
本教程介紹瞭如何在 Python 中查詢文字檔案中的特定字串。
在 Python 中使用檔案 readlines()
方法查詢檔案中的字串
Pyton 檔案 readlines()
方法將檔案內容按新行分割成一個列表返回。我們可以使用 for
迴圈對列表進行遍歷,並在每次迭代中使用 in
操作符檢查字串是否在行中。
如果在行中找到了字串,則返回 True
並中斷迴圈。如果在迭代所有行後沒有找到字串,它最終返回 False
。
下面給出了這種方法的示例程式碼。
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
def check_string():
with open("temp.txt") as temp_f:
datafile = temp_f.readlines()
for line in datafile:
if "blabla" in line:
return True # The string is found
return False # The string does not exist in the file
if check_string():
print("True")
else:
print("False")
輸出:
True
在 Python 中使用檔案 read()
方法搜尋檔案中的字串
檔案 read()
方法將檔案的內容作為一個完整的字串返回。然後我們可以使用 in
操作符來檢查該字串是否在返回的字串中。
下面給出一個示例程式碼。
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
if "blabla" in f.read():
print("True")
輸出:
True
在 Python 中使用 find
方法搜尋檔案中的字串
一個簡單的 find
方法可以與 read()
方法一起使用,以找到檔案中的字串。find
方法被傳遞給所需的字串。如果找到了字串,它返回 0
,如果沒有找到,則返回 -1
。
下面給出一個示例程式碼。
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
print(open("temp.txt", "r").read().find("blablAa"))
輸出:
-1
在 Python 中使用 mmap
模組搜尋檔案中的字串
mmap
模組也可以用來在 Python 中查詢檔案中的字串,如果檔案大小比較大,可以提高效能。mmap.mmap()
方法在 Python 2 中建立了一個類似字串的物件,它只檢查隱含的檔案,不讀取整個檔案。
下面給出一個 Python 2 中的示例程式碼。
# python 2
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find("blabla") != -1:
print("True")
輸出:
True
然而,在 Python 3 及以上版本中,mmap
並不像字串物件一樣,而是建立一個 bytearray
物件。所以 find
方法是尋找位元組而不是字串。
下面給出一個示例程式碼。
import mmap
file = open("temp.txt", "w")
file.write("blabla is nothing.")
file.close()
with open("temp.txt") as f:
s = mmap.mmap(f.fileno(), 0, access=mmap.ACCESS_READ)
if s.find(b"blabla") != -1:
print("True")
輸出:
True
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn相關文章 - Python String
- 在 Python 中從字串中刪除逗號
- 如何以 Pythonic 方式檢查字符串是否為空
- 在 Python 中將字串轉換為變數名
- Python 如何去掉字串中的空格/空白符
- 如何在 Python 中從字串中提取數字
- Python 如何將字串轉換為時間日期 datetime 格式