Data Classes in Python
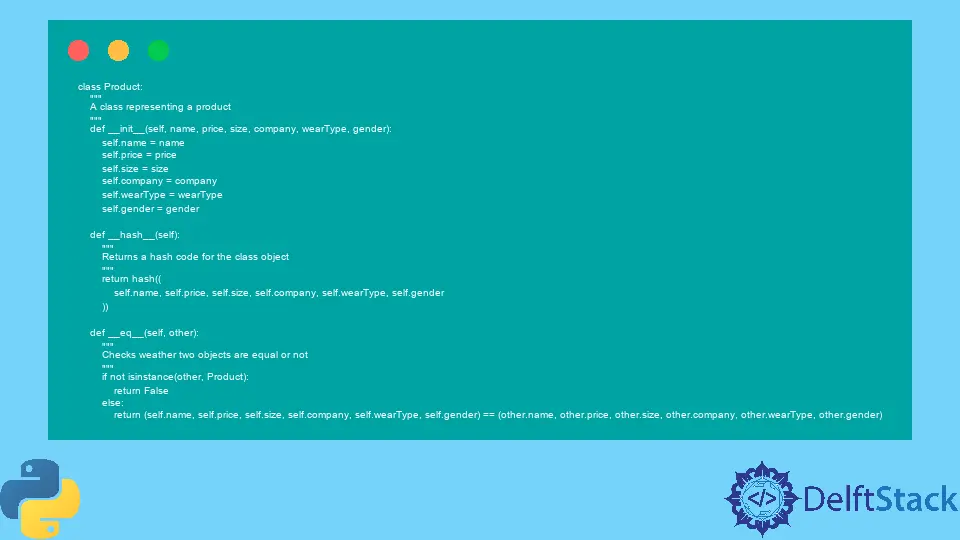
The general classes found in Python or any other programming language are designed to represent an entity. Since they represent an entity, they are filled with lots of functions, logic, and attributes, where each function performs a specific task, and the class attributes are manipulated using the class functions.
Apart from the general classes, there is yet another type of class, namely, data class. This article will talk about data classes found not only in Python but also in other programming languages.
Python DataClass
Unlike these regular classes, data classes are geared towards storing the state of an entity. They don’t contain a lot of logic. They store some data that represents a stats of an object or an entity.
Let’s understand the data classes better with an example. For example, you are writing a program that tries to mimic the behavior of a shopping cart at a clothing shop. The shopping cart will hold products or clothes. Consider there are three types of clothes, namely, Headwear
, Topwear
, and Bottomwear
. Since every gender can wear not every cloth, we also have information about the gender a cloth is manufactured for. Let’s consider only three categories: Male
, Female
, and Neutral (Both can wear it)
. Every product has a name, price, size, and company it was manufactured by.
Now, if we have to create a program to mimic a shopping cart, we can create two classes, namely, ShoppingCart
and Product
. The ShoppingCart
class will hold all the logic, such as adding and removing products, manipulating the number of products, keeping track of the products present inside the cart, etc. And, a single product will be represented by the Product
class. Product
is a data class, and it will just represent a product.
The Product
class will look something like this.
class Product:
"""
A class representing a product
"""
def __init__(self, name, price, size, company, wearType, gender):
self.name = name
self.price = price
self.size = size
self.company = company
self.wearType = wearType
self.gender = gender
def __hash__(self):
"""
Returns a hash code for the class object
"""
return hash(
(self.name, self.price, self.size, self.company, self.wearType, self.gender)
)
def __eq__(self, other):
"""
Checks weather two objects are equal or not
"""
if not isinstance(other, Product):
return False
else:
return (
self.name,
self.price,
self.size,
self.company,
self.wearType,
self.gender,
) == (
other.name,
other.price,
other.size,
other.company,
other.wearType,
other.gender,
)
As you can see, the Product
class does not have any logic. All it does is represent a product. This is what a data class looks like, just attributes and minimal logic.
The two functions implemented are necessary if we want the data class to be hashable. When a class is hashable, its object can be used as a key in the dictionary and can map to fixed values. Since a hash code will be needed to map values, these two functions are essential.
One can also implement the __repr__
function, a particular method that returns the string representation of a class object.
Related Article - Python Class
- Python Generator Class
- Data Class Inheritance in Python
- How to Serialize a Python Class Object to JSON
- Python Abstract Property
- Python Class Factory
- Python Class Equality