Structures in Python
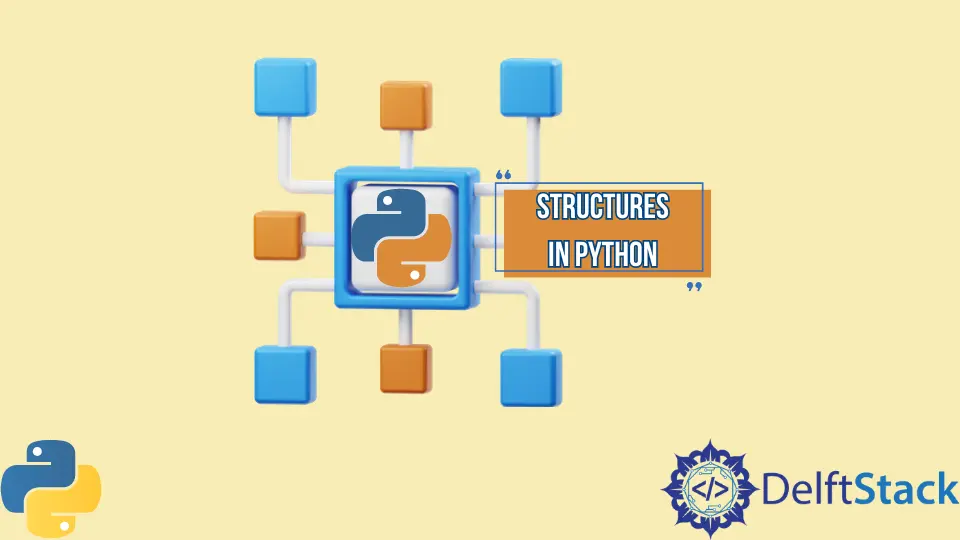
Python is an object-oriented programming language which means it has classes and objects. Whereas C is not an object-oriented programming language, meaning one can only perform functional programming using it. But C has structures in it which is a user-defined datatype.
We don’t have structures in Python, but we can implement them or represent their behavior using other objects, namely, data classes. A data class is a class that only contains fields and CRUD methods (getters and setters) to manipulate these fields. The main objective of these classes is to store data or represent some data in the form of a package. A data classes represent entities, and since entities have multiple parameters or properties, data classes make it easier to package everything under a single object.
In this article, we will learn how to use data classes in Python.
Data Class in Python
Python 3.7 introduced Data Classes. Although data classes can be implemented using primitive Python classes, a data class implements most of the required functionalities behind the scenes, making the Python code for a data class shorter and readable. Implementation using primitive Python classes will allow more control, but for bigger data classes, the implementation might get messy and massive, making it unmanageable.
Python has a decorator dataclass
, defined inside dataclasses
module. This decorator automatically adds special methods required inside a data classes such as __init__()
, __repr__()
, etc.
Here is an example.
from dataclasses import dataclass
@dataclass
class Item:
name: str
price: float
quantity: int
def total_cost(self) -> float:
return self.price * self.quantity
As mentioned above, @dataclass
decorator adds special methods automatically. This means that the following __init__()
method will be added automatically.
def __init__(self, name: str, price: float, quantity: int = 0):
self.name = name
self.price = price
self.quantity = quantity
Further, this data class can be used to create objects of type Item
, and these objects can be treated like normal class objects.
from dataclasses import dataclass
@dataclass
class Item:
name: str
price: float
quantity: int
def total_cost(self):
return self.price * self.quantity
a = Item("Chocolate", 25, 100)
b = Item("Chips", 35, 150)
c = Item("Cookie", 10, 50)
print(a)
print(b)
print(c)
print(a.total_cost())
print(b.total_cost())
print(c.total_cost())
The dataclass
decorator has some parameters, namely,
init
-True
by default. IfTrue
, the__init__()
method of the data class will be defined.repr
-True
by default. IfTrue
, the__repr__()
method of the data class will be defined.eq
-True
by default. IfTrue
, the__eq__()
method of the data class will be defined.order
-False
by default. IfTrue
, the__lt__()
,__le__()
,__gt__()
, and__ge__()
methods of the data class will be defined.unsafe_hash
-False
by default. IfFalse
, the__hash__()
method of the data class will be defined.frozen
-False
by default. IfTrue
, the data class attributes will get frozen. This means, once initialized, they can’t further be manipulated.match_args
-True
by default.kw_only
-False
by default. IfTrue
, all fields of the data class will be marked as keyword-only.slots
-False
by default.
These parameters can be configured when placing a dataclass
decorator over a class implementation. Some examples of the same are as follows.
@dataclass
class A:
pass
@dataclass(init=True, repr=True, order=True)
class B:
pass
@dataclass(
eq=True,
order=False,
unsafe_hash=True,
)
class C:
pass
To learn more about the module
dataclasses
refer to the official documentation here.