Python 中的結構體
Vaibhav Vaibhav
2023年10月10日
Python
Python Dataclass
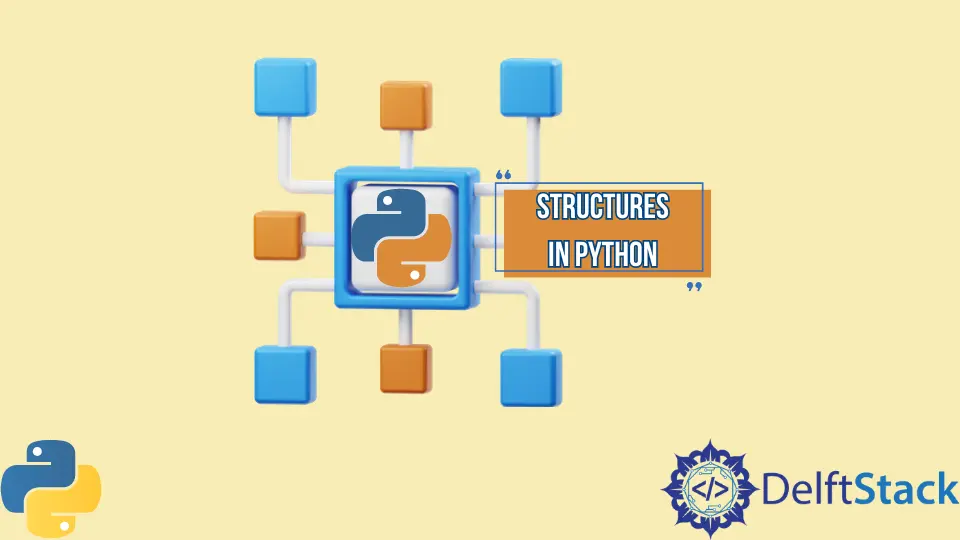
Python 是一種物件導向的程式語言,這意味著它具有類和物件。而 C 不是一種物件導向的程式語言,這意味著只能使用它執行函數語言程式設計。但是 C 語言中有結構,它是使用者定義的資料型別。
我們在 Python 中沒有結構,但我們可以使用其他物件(即資料類)實現它們或表示它們的行為。資料類是隻包含欄位和用於操作這些欄位的 CRUD 方法(getter 和 setter)的類。這些類的主要目標是以包的形式儲存資料或表示一些資料。資料類代表實體,並且由於實體具有多個引數或屬性,因此資料類可以更輕鬆地將所有內容打包到單個物件下。
在本文中,我們將學習如何在 Python 中使用資料類。
Python 中的 dataclass
Python 3.7 引入了資料類。儘管可以使用原始 Python 類來實現資料類,但資料類在幕後實現了大部分所需的功能,從而使資料類的 Python 程式碼更短且可讀。使用原始 Python 類的實現將允許更多的控制,但對於更大的資料類,實現可能會變得混亂和龐大,使其難以管理。
Python 有一個裝飾器 dataclass
,定義在 dataclasses
模組中。該裝飾器會自動新增資料類中所需的特殊方法,例如 __init__()
、__repr__()
等。
這是一個例子。
from dataclasses import dataclass
@dataclass
class Item:
name: str
price: float
quantity: int
def total_cost(self) -> float:
return self.price * self.quantity
如上所述,@dataclass
裝飾器會自動新增特殊方法。這意味著將自動新增以下 __init__()
方法。
def __init__(self, name: str, price: float, quantity: int = 0):
self.name = name
self.price = price
self.quantity = quantity
此外,該資料類可用於建立 Item
型別的物件,並且可以將這些物件視為普通類物件。
from dataclasses import dataclass
@dataclass
class Item:
name: str
price: float
quantity: int
def total_cost(self):
return self.price * self.quantity
a = Item("Chocolate", 25, 100)
b = Item("Chips", 35, 150)
c = Item("Cookie", 10, 50)
print(a)
print(b)
print(c)
print(a.total_cost())
print(b.total_cost())
print(c.total_cost())
dataclass
裝飾器有一些引數,即
init
-True
預設情況下。如果True
,將定義資料類的__init__()
方法。repr
-True
預設情況下。如果True
,將定義資料類的__repr__()
方法。eq
-True
預設情況下。如果True
,將定義資料類的__eq__()
方法。order
-False
預設情況下。如果為True
,將定義資料類的__lt__()
、__le__()
、__gt__()
和__ge__()
方法。unsafe_hash
- 預設情況下為False
。如果為False
,將定義資料類的__hash__()
方法。frozen
- 預設情況下是False
。如果為True
,資料類屬性將被凍結。這意味著,一旦初始化,它們就不能被進一步操縱。match_args
- 預設情況下為True
。kw_only
- 預設情況下為False
。如果True
,資料類的所有欄位都將被標記為僅關鍵字。slots
-False
預設情況下。
當在類實現上放置 dataclass
裝飾器時,可以配置這些引數。下面是一些相同的例子。
@dataclass
class A:
pass
@dataclass(init=True, repr=True, order=True)
class B:
pass
@dataclass(
eq=True,
order=False,
unsafe_hash=True,
)
class C:
pass
要了解有關模組
dataclasses
的更多資訊,請參閱此處官方文件。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav