Python 中的資料類
Vaibhav Vaibhav
2021年12月4日
Python
Python Class
Python Dataclass
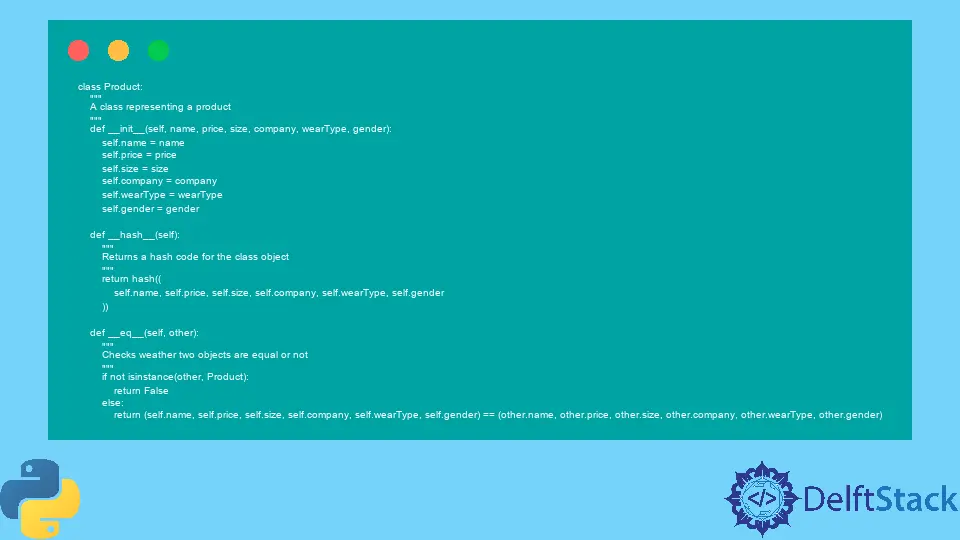
Python 或任何其他程式語言中的通用類旨在表示實體。由於它們代表一個實體,因此它們充滿了許多函式、邏輯和屬性,其中每個函式執行特定任務,並且使用類函式來操作類屬性。
除了通用類之外,還有一種類,即資料類。本文將討論不僅在 Python 中而且在其他程式語言中都可以找到的資料類。
Python 資料類
與這些常規類不同,資料類旨在儲存實體的狀態。它們不包含很多邏輯。它們儲存一些表示物件或實體的統計資料的資料。
讓我們通過一個例子更好地理解資料類。例如,你正在編寫一個程式,試圖模擬服裝店購物車的行為。購物車將放置產品或衣服。考慮有三種型別的衣服,即 Headwear
、Topwear
和 Bottomwear
。由於並非每種性別都可以穿每種布料,因此我們還掌握了有關製造布料的性別的資訊。讓我們只考慮三個類別:男
、女
和中性(兩者都可以穿)
。每個產品都有名稱、價格、尺寸和製造公司。
現在,如果我們必須建立一個程式來模擬購物車,我們可以建立兩個類,即 ShoppingCart
和 Product
。ShoppingCart
類將儲存所有邏輯,例如新增和刪除產品、操作產品數量、跟蹤購物車內的產品等。並且,單個產品將由 Product
類表示. Product
是一個資料類,它只代表一個產品。
Product
類看起來像這樣。
class Product:
"""
A class representing a product
"""
def __init__(self, name, price, size, company, wearType, gender):
self.name = name
self.price = price
self.size = size
self.company = company
self.wearType = wearType
self.gender = gender
def __hash__(self):
"""
Returns a hash code for the class object
"""
return hash(
(self.name, self.price, self.size, self.company, self.wearType, self.gender)
)
def __eq__(self, other):
"""
Checks weather two objects are equal or not
"""
if not isinstance(other, Product):
return False
else:
return (
self.name,
self.price,
self.size,
self.company,
self.wearType,
self.gender,
) == (
other.name,
other.price,
other.size,
other.company,
other.wearType,
other.gender,
)
如你所見,Product
類沒有任何邏輯。它所做的只是代表一個產品。這就是資料類的樣子,只有屬性和最少的邏輯。
如果我們希望資料類是可雜湊的,那麼實現的兩個函式是必需的。當一個類是可雜湊的時,它的物件可以用作字典中的鍵並且可以對映到固定值。由於需要雜湊碼來對映值,因此這兩個函式是必不可少的。
還可以實現 __repr__
函式,這是一種返回類物件的字串表示的特定方法。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav