Python new 關鍵字
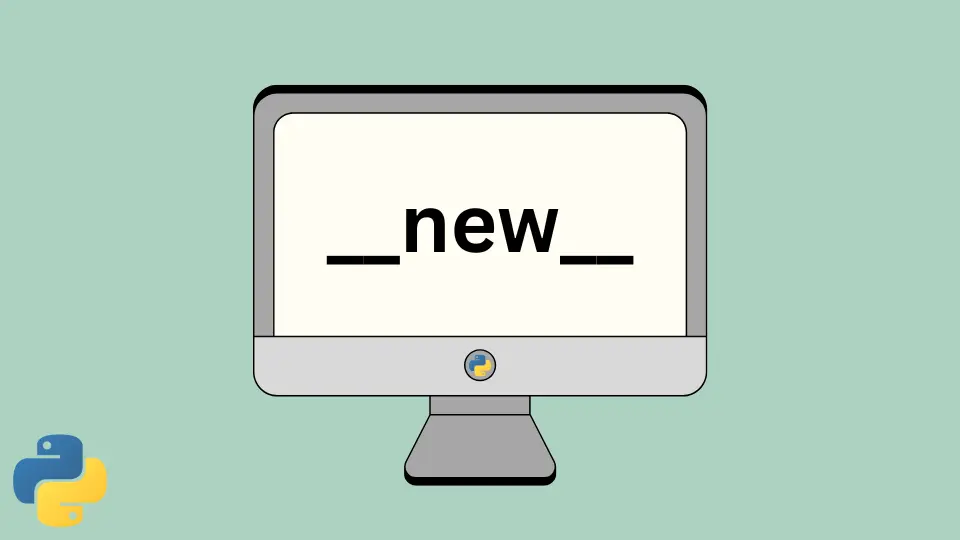
在 C++ 和 Java 等程式語言中,你可以顯式使用 new 關鍵字從類建立物件。但是,你不能直接在 Python 中使用 new 關鍵字。在本文中,我們將討論 new 關鍵字在 Python 中的工作原理。我們還將看到如何顯式地使用 new 關鍵字來觀察 Python 中物件建立的過程。
Python 中的 new
關鍵字是什麼
在 Python 中,__new__
是與物件關聯的屬性。你可以使用 dir()
函式檢查物件的所有屬性。dir()
函式將物件作為輸入並返回所有物件屬性的列表,如下所示。
class Car:
def __init__(self, model, brand, price, country):
self.Brand = brand
self.Model = model
self.Price = price
self.Country = country
myCar = Car("Tesla", "Cybertruck", 40000, "USA")
print(dir(myCar))
輸出:
['Brand', 'Country', 'Model', 'Price', '__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__']
你可以觀察到列表中存在一個 __new__
屬性。
在 Python 中如何使用 new
關鍵字
我們不能像在其他程式語言中那樣顯式地在 Python 中使用 new 關鍵字。但是,Python 中的 __new__
屬性與其他程式語言中的 new
關鍵字的作用相同。__new__()
方法在基類object
中定義,用於為物件分配儲存空間。
當我們通過使用語法 className()
呼叫類名來建立一個新物件時,會執行該類的 __call__()
方法。__call__()
方法呼叫 __new__()
方法。在這裡,__new__()
方法為物件分配記憶體並將控制權返回給 __call__()
方法。
__call__()
方法然後呼叫 __init__()
方法。__init__()
方法初始化類變數並將控制權返回給 __call__()
方法。__call__()
方法然後完成執行並將對建立的物件的引用返回給我們。
我們如何顯式實現 __new__()
方法
我們不需要在 Python 中顯式實現 __new__()
方法。但是,如果你想檢視 __new__()
方法和 __init__()
方法的執行順序,我們可以覆蓋 __new__()
方法。正如我們在上面看到的,__new__()
方法是一個靜態方法,並在物件類中定義。當我們使用類名建立一個物件時,它的 __new__()
方法會被自動呼叫。
__new__()
方法接受一個代表當前類的輸入引數 cls
。然後 __new__()
方法使用當前類作為輸入引數呼叫其超類的 __new__()
方法。這個過程需要繼續,直到我們到達基類 object
。在基類 object
中,執行 __new__()
方法,併為我們要建立的物件分配儲存空間。然後,建立物件的例項。__new__()
方法然後返回例項,之後 __init__()
方法用於初始化例項中的變數。
你可以在以下示例中觀察整個過程。
class Car:
def __init__(self, brand, model, price, country):
print("I am in the __init__() method.")
self.Brand = brand
self.Model = model
self.Price = price
self.Country = country
def __new__(cls, *args, **kwargs):
print("I am in the __new__() method.")
return super(Car, cls).__new__(cls)
myCar = Car("Tesla", "Cybertruck", 40000, "USA")
輸出:
I am in the __new__() method.
I am in the __init__() method.
這裡,我們實現的 __new__()
方法屬於當前類,它不會影響任何物件例項的建立方式。為了改變它,我們需要改變基類 object
中的 __new__()
方法。但是,我們不允許這樣做,你不應該嘗試進行任何此類實驗。此外,你可以在輸出中驗證 __new__()
方法在 __init__()
方法之前執行。
まとめ
我們在本文中討論了 Python 中的 new 關鍵字。我們還看到了如何使用 dir()
方法獲取與類關聯的所有屬性。此外,我們還看到了如何在 Python 中建立物件以及 __new__()
方法是如何工作的。我們還自己實現了 __new__()
方法。但是,它不是很有用,我建議你不要自己實現 __new__()
方法。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub