Python new Keyword
-
What Is the
__new__
Keyword in Python? - How to Use the New Keyword in Python?
-
How Can We Explicitly Implement the
__new__()
Method? - Conclusion
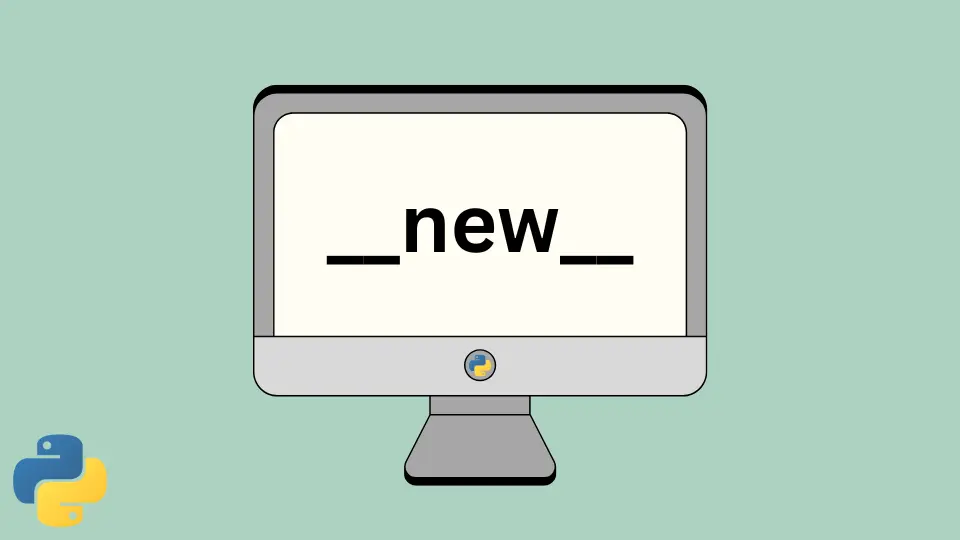
In programming languages like C++ and Java, you can use the new
keyword explicitly to create an object from a class. However, you cannot use the new
keyword directly in Python.
In this article, we will discuss how the new keyword works in Python. We will also see how we can explicitly use the new
keyword to observe the process of object creation in Python.
What Is the __new__
Keyword in Python?
In python, __new__
is an attribute associated with the objects. You can check all the attributes of an object using the dir()
function. The dir()
function takes the object as input and returns a list of all the object attributes as follows.
class Car:
def __init__(self, model, brand, price, country):
self.Brand = brand
self.Model = model
self.Price = price
self.Country = country
myCar = Car("Tesla", "Cybertruck", 40000, "USA")
print(dir(myCar))
Output:
['Brand', 'Country', 'Model', 'Price', '__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__']
You can observe that there is a __new__
attribute present in the list.
How to Use the New Keyword in Python?
We cannot explicitly use the new keyword in Python as we do in other programming languages. However, the __new__
attribute in Python works the same as the new keyword in other programming languages. The __new__()
method is defined in the base class object
and is used to allocate storage for an object.
When we create a new object by calling the class name using the syntax className()
, the __call__()
method of the class is executed. The __call__()
method calls the __new__()
method. Here, the __new__()
method allocates memory for the object and returns the control to the __call__()
method.
The __call__()
method then calls the __init__()
method. The __init__()
method initializes the class variables and returns the control to the __call__()
method. The __call__()
method then finishes the execution and returns the reference to the created object to us.
How Can We Explicitly Implement the __new__()
Method?
We don’t need to explicitly implement the __new__()
method in Python. However, if you want to see the order in which the __new__()
method and the __init__()
method are executed, we can override the __new__()
method. As we have seen above, the __new__()
method is a static method and is defined in the object class. When we create an object using the class name, its __new__()
method is automatically invoked.
The __new__()
method takes an input argument cls
that represents the current class. Then the __new__()
method calls the __new__()
method of its superclass with the current class as the input argument. This process needs to continue until we reach the base class object
. In the base class object
, the __new__()
method is executed, and storage is allocated for the object that we want to create. Then, an instance of the object is created. The __new__()
method then returns the instance, after which the __init__()
method is used to initialize the variables in the instance.
You can observe this entire process in the following example.
class Car:
def __init__(self, brand, model, price, country):
print("I am in the __init__() method.")
self.Brand = brand
self.Model = model
self.Price = price
self.Country = country
def __new__(cls, *args, **kwargs):
print("I am in the __new__() method.")
return super(Car, cls).__new__(cls)
myCar = Car("Tesla", "Cybertruck", 40000, "USA")
Output:
I am in the __new__() method.
I am in the __init__() method.
Here, the __new__()
method that we have implemented belongs to the current class, and it doesn’t affect the way in which an instance of any object is created. To change that, we will need to change the __new__()
method in the base class object
. But, we are not allowed to do so, and you should not try to do any such experiment. Also, you can verify in the output that the __new__()
method is executed before the __init__()
method.
Conclusion
We have discussed the new keyword in Python in this article. We also saw how we could obtain all the attributes associated with a class using the dir()
method. Moreover, we also saw how an object is created in Python and how the __new__()
method works. We have also implemented the __new__()
method on our own. However, it is not very useful, and I would suggest you not implement the __new__()
method on your own.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub