Python の new キーワード
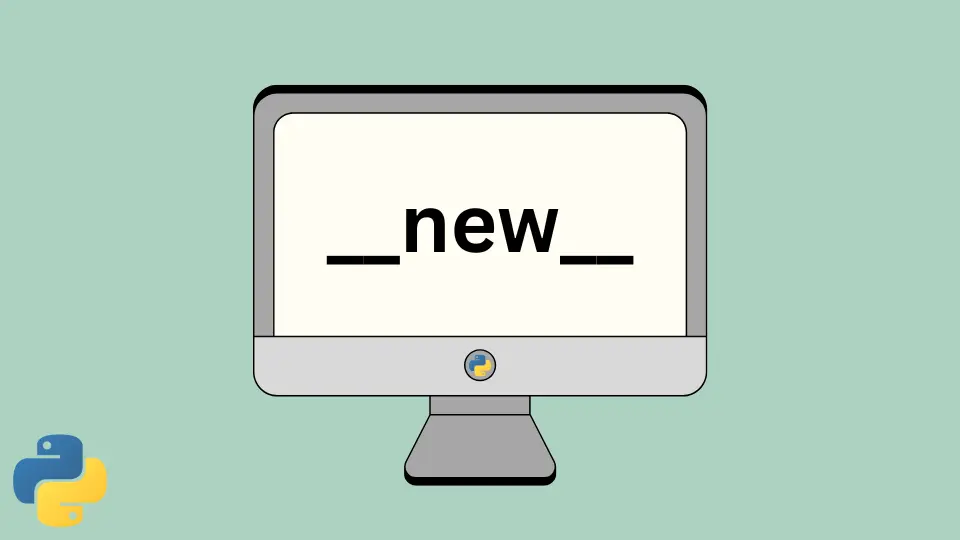
C++ や Java などのプログラミング言語では、new
キーワードを明示的に使用して、クラスからオブジェクトを作成できます。ただし、Python で new
キーワードを直接使用することはできません。この記事では、Python で new
キーワードがどのように機能するかについて説明します。また、new
キーワードを明示的に使用して、Python でのオブジェクト作成のプロセスを観察する方法についても説明します。
Python の new
キーワードは何ですか
Python では、__new__
はオブジェクトに関連付けられた属性です。dir()
関数を使用して、オブジェクトのすべての属性を確認できます。dir()
関数は、オブジェクトを入力として受け取り、次のようにすべてのオブジェクト属性のリストを返します。
class Car:
def __init__(self, model, brand, price, country):
self.Brand = brand
self.Model = model
self.Price = price
self.Country = country
myCar = Car("Tesla", "Cybertruck", 40000, "USA")
print(dir(myCar))
出力:
['Brand', 'Country', 'Model', 'Price', '__class__', '__delattr__', '__dict__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__le__', '__lt__', '__module__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', '__weakref__']
リストに __new__
属性が存在することがわかります。
Python で new
キーワードを使用する方法は
他のプログラミング言語のように、Python で new キーワードを明示的に使用することはできません。ただし、Python の __new__
属性は、他のプログラミング言語の new キーワードと同じように機能します。__new__
メソッドは基本クラス object
で定義され、オブジェクトにストレージを割り当てるために使用されます。
構文 className()
を使用してクラス名を呼び出して新しいオブジェクトを作成すると、クラスの __call__()
メソッドが実行されます。__call__()
メソッドは __new__()
メソッドを呼び出します。ここで、__new__()
メソッドはオブジェクトにメモリを割り当て、制御を __call__()
メソッドに返します。
次に、__call__()
メソッドが __init__()
メソッドを呼び出します。__init__()
メソッドはクラス変数を初期化し、制御を __call__()
メソッドに返します。次に、__call__()
メソッドは実行を終了し、作成されたオブジェクトへの参照を返します。
__new__()
メソッドを明示的に実装するにはどうすればよいですか
Python で __new__()
メソッドを明示的に実装する必要はありません。ただし、__new__()
メソッドと __init__()
メソッドが実行される順序を確認したい場合は、__new__()
メソッドをオーバーライドできます。上で見たように、__new__()
メソッドは静的メソッドであり、オブジェクトクラスで定義されています。クラス名を使用してオブジェクトを作成すると、その __new__()
メソッドが自動的に呼び出されます。
__new__()
メソッドは、現在のクラスを表す入力引数 cls
を取ります。次に、__new__()
メソッドは、現在のクラスを入力引数として、そのスーパークラスの __new__()
メソッドを呼び出します。このプロセスは、基本クラスオブジェクト
に到達するまで継続する必要があります。基本クラス object
では、__new__()
メソッドが実行され、作成するオブジェクトにストレージが割り当てられます。次に、オブジェクトのインスタンスが作成されます。次に、__new__()
メソッドがインスタンスを返し、その後、__init__()
メソッドを使用してインスタンス内の変数を初期化します。
次の例では、このプロセス全体を観察できます。
class Car:
def __init__(self, brand, model, price, country):
print("I am in the __init__() method.")
self.Brand = brand
self.Model = model
self.Price = price
self.Country = country
def __new__(cls, *args, **kwargs):
print("I am in the __new__() method.")
return super(Car, cls).__new__(cls)
myCar = Car("Tesla", "Cybertruck", 40000, "USA")
出力:
I am in the __new__() method.
I am in the __init__() method.
ここで、実装した __new__()
メソッドは現在のクラスに属しており、オブジェクトのインスタンスが作成される方法には影響しません。これを変更するには、基本クラス object
の __new__()
メソッドを変更する必要があります。ただし、そうすることは許可されていないため、そのような実験を試みるべきではありません。また、出力で、__init__()
メソッドが __init__()
メソッドの前に実行されていることを確認できます。
まとめ
この記事では、Python の new キーワードについて説明しました。また、dir()
メソッドを使用して、クラスに関連付けられているすべての属性を取得する方法も確認しました。さらに、Python でオブジェクトがどのように作成され、__new__()
メソッドがどのように機能するかも確認しました。また、__new__()
メソッドを独自に実装しました。ただし、これはあまり有用ではないため、__new__()
メソッドを自分で実装しないことをお勧めします。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub