Python 中的数据类
Vaibhav Vaibhav
2021年12月4日
Python
Python Class
Python Dataclass
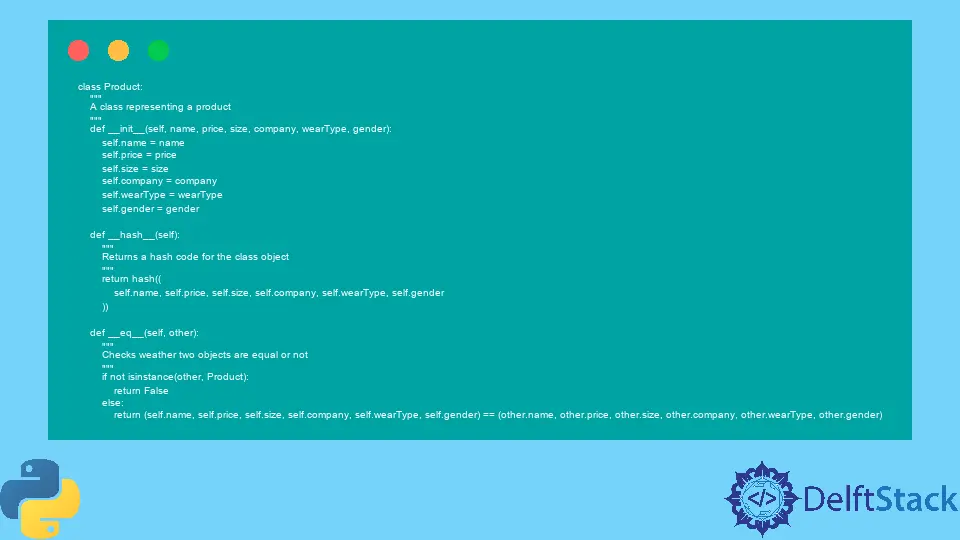
Python 或任何其他编程语言中的通用类旨在表示实体。由于它们代表一个实体,因此它们充满了许多函数、逻辑和属性,其中每个函数执行特定任务,并且使用类函数来操作类属性。
除了通用类之外,还有一种类,即数据类。本文将讨论不仅在 Python 中而且在其他编程语言中都可以找到的数据类。
Python 数据类
与这些常规类不同,数据类旨在存储实体的状态。它们不包含很多逻辑。它们存储一些表示对象或实体的统计数据的数据。
让我们通过一个例子更好地理解数据类。例如,你正在编写一个程序,试图模拟服装店购物车的行为。购物车将放置产品或衣服。考虑有三种类型的衣服,即 Headwear
、Topwear
和 Bottomwear
。由于并非每种性别都可以穿每种布料,因此我们还掌握了有关制造布料的性别的信息。让我们只考虑三个类别:男
、女
和中性(两者都可以穿)
。每个产品都有名称、价格、尺寸和制造公司。
现在,如果我们必须创建一个程序来模拟购物车,我们可以创建两个类,即 ShoppingCart
和 Product
。ShoppingCart
类将保存所有逻辑,例如添加和删除产品、操作产品数量、跟踪购物车内的产品等。并且,单个产品将由 Product
类表示. Product
是一个数据类,它只代表一个产品。
Product
类看起来像这样。
class Product:
"""
A class representing a product
"""
def __init__(self, name, price, size, company, wearType, gender):
self.name = name
self.price = price
self.size = size
self.company = company
self.wearType = wearType
self.gender = gender
def __hash__(self):
"""
Returns a hash code for the class object
"""
return hash(
(self.name, self.price, self.size, self.company, self.wearType, self.gender)
)
def __eq__(self, other):
"""
Checks weather two objects are equal or not
"""
if not isinstance(other, Product):
return False
else:
return (
self.name,
self.price,
self.size,
self.company,
self.wearType,
self.gender,
) == (
other.name,
other.price,
other.size,
other.company,
other.wearType,
other.gender,
)
如你所见,Product
类没有任何逻辑。它所做的只是代表一个产品。这就是数据类的样子,只有属性和最少的逻辑。
如果我们希望数据类是可散列的,那么实现的两个函数是必需的。当一个类是可散列的时,它的对象可以用作字典中的键并且可以映射到固定值。由于需要哈希码来映射值,因此这两个函数是必不可少的。
还可以实现 __repr__
函数,这是一种返回类对象的字符串表示的特定方法。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav