How to Convert Dataclass to JSON in Python
-
Understanding Python
Dataclasses
- the Importance of JSON Serialization
- Basic Conversion Using the JSON Module
- Custom JSON Encoder
- Custom Serialization Function
-
Best Practices for
Dataclass
to JSON Conversion - Troubleshooting Common Issues
- Conclusion
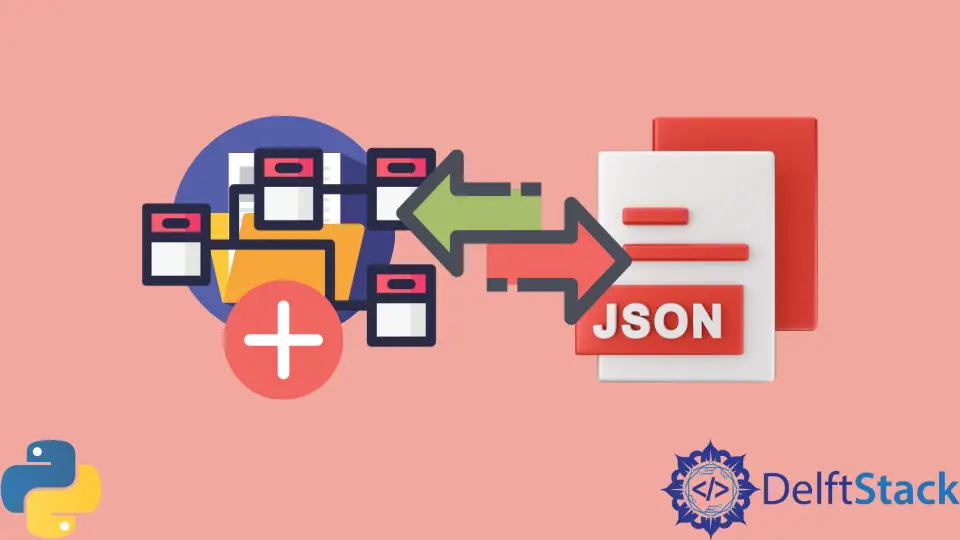
Converting dataclasses
to JSON in Python involves a nuanced understanding of Python classes and the crucial aspect of JSON serialization. To initiate this process, we explore the basics using the native json
module, subsequently delving into advanced techniques with third-party libraries like dataclasses_json
.
Our exploration includes best practices for seamlessly converting dataclass
instances to JSON representations, considering aspects such as default values, diverse data types, and adherence to conventions. Throughout the article, we address common issues and troubleshooting strategies, ensuring a comprehensive guide for efficiently transforming Python data objects into JSON format.
Understanding Python Dataclasses
Python dataclasses
simplify the creation of classes for storing data. By using the dataclass
decorator, developers can effortlessly define classes with default values, type annotations, and more.
These classes are highly readable, reducing boilerplate code. With the asdict()
, converting dataclass
instances to JSON becomes straightforward, allowing seamless integration with JSON-based workflows.
the Importance of JSON Serialization
JSON serialization is crucial in Python for seamless data interchange between dataclasses
and JSON objects. By converting dataclass
instances to JSON strings using asdict()
, developers ensure compatibility with external systems, web services, and file storage.
This process, facilitated by the json
module, allows Python objects to be efficiently represented as JSON, enabling easy integration into a wide range of applications. The simplicity and versatility of this serialization mechanism streamline data handling, promoting effective communication and interoperability within diverse programming environments.
In Python version 3.7 and beyond, JSON serialization serves as a fundamental and valuable feature, emphasizing clarity and ease of use.
Basic Conversion Using the JSON Module
In Python, working with dataclasses
and JSON is a common requirement, especially when dealing with data serialization and interchange between different systems.
One approach to achieve this is using the json.dump()
function along with the dataclasses_json
package. This combination provides a convenient and powerful way to convert dataclasses
to JSON format.
the json.dump()
Method
The json.dump()
method in Python plays a pivotal role in converting Python objects, including dataclasses
, into a JSON-formatted string for serialization. This method is particularly significant when dealing with data interchange, storage, or communication between different systems.
Using the dataclasses_json
Package
The dataclasses_json
package plays a pivotal role in simplifying the process of converting Python dataclasses
to JSON. With this package, the conversion becomes seamless and efficient, addressing complexities that arise when dealing with nested data structure, default value, and datetime object.
By leveraging the package’s capabilities, developers can effortlessly transform dataclass
instance into JSON string or entire JSON objects. The library enhances the default behavior of the dataclass
module, providing a replacement that offers additional features, such as handling fields and supporting custom serialization logic.
Its compatibility with Python 3.7 and above, along with its user-friendly design, makes it a valuable tool for ensuring full support for a wide range of data types. Through a straightforward installation process using pip install
, developers can integrate this library into their projects, making the conversion from dataclasses
to JSON a streamlined and error-resistant task.
The dataclasses_json
package thus stands as a valuable asset, simplifying the serialization of Python objects and promoting code clarity and conciseness.
Using json.dump()
and dataclasses_json
In modern Python development, efficient serialization and deserialization of data are crucial tasks, especially when dealing with complex data structures. The dataclasses
module, introduced in Python 3.7, simplifies the creation of classes that primarily store data.
When it comes to converting these dataclasses
to JSON, developers have several approaches, and two popular methods include using the json.dump()
method and the dataclasses_json
package.
Let’s delve into a practical example using a Person
dataclass
to understand the conversion process. The code employs both dataclasses_json
and the standard json
module.
Code Example:
from dataclasses import dataclass
from dataclasses_json import dataclass_json
import json
# Define a data class with the @dataclass_json decorator
@dataclass_json
@dataclass
class Person:
name: str
age: int
# Create an instance of the data class
person_instance = Person(name="John", age=30)
# Convert the data class instance to a JSON string using dataclasses_json
json_dataclasses_json = person_instance.to_json()
# Convert the data class instance to a JSON string using json.dumps
json_json_dumps = json.dumps(person_instance, default=lambda o: o.__dict__, indent=2)
# Print the results
print("JSON using dataclasses_json:")
print(json_dataclasses_json)
print("\nJSON using json.dumps:")
print(json_json_dumps)
In the first step, we define a straightforward dataclass
named Person
by applying the @dataclass
decorator. To leverage automatic serialization and deserialization capabilities, we enhance it with the @dataclass_json
decorator.
Moving on to the second step, we instantiate the Person
class, creating an object that represents an individual named John
with an age of 30.
The third step involves the utilization of the to_json()
method from the dataclasses_json
package. This method facilitates the conversion of the dataclass
instance into a JSON-formatted string, streamlining the serialization process.
For comparison, the fourth step employs the standard json.dumps()
method. A lambda function is passed as the default
argument, enabling the conversion of the dataclass
instance to a Python dictionary suitable for JSON serialization.
In the final step, we print the results obtained from both methods, showcasing the JSON representations of the dataclass
instance produced by dataclasses_json
and the traditional json.dumps()
method. This comprehensive process highlights the ease and effectiveness of utilizing the dataclasses_json
package for converting dataclasses
to JSON in Python.
The output showcases the JSON representation of the Person
instance using both dataclasses_json
and json.dumps
. This comparison illustrates the simplicity and advantages offered by each method in converting dataclasses
to JSON.
Running this code would produce the following output:
By exploring these techniques, developers can make informed decisions based on their specific use cases, considering factors such as simplicity, extensibility, and overall compatibility with their projects.
Custom JSON Encoder
In the realm of Python programming, the concept of converting dataclasses
to JSON holds paramount importance, especially in scenarios where data interchange and serialization become key considerations. Dataclasses
provide an elegant and concise way to define classes meant for storing data.
To harness the power of these classes for interoperability, developers often need to convert them into JSON format.
This article explores a method to achieve this conversion using a custom JSON encoder. The json
module in Python provides the means to serialize Python objects into JSON strings, and by employing a custom encoder, developers can tailor the serialization process to suit the needs of dataclasses
.
Let’s dive into a comprehensive example that demonstrates the conversion of a dataclass
to a JSON string using a custom JSON encoder.
Code Example:
from dataclasses import dataclass, asdict, is_dataclass
import json
class DataClassEncoder(json.JSONEncoder):
def default(self, obj):
if is_dataclass(obj):
return asdict(obj)
return super().default(obj)
@dataclass
class Person:
name: str
age: int
person_instance = Person(name="John", age=30)
json_data = json.dumps(person_instance, cls=DataClassEncoder)
print(json_data)
In this example, we demonstrate the conversion of a Python dataclass
named Person
into a JSON string using a custom JSON encoder. The custom encoder, defined as the DataClassEncoder
class, inherits from json.JSONEncoder
and includes an overridden def default
method.
This method checks if it is a dataclass
object using is_dataclass(obj)
and, if true, converts it to a dictionary with asdict(obj)
.
The Person
dataclass
is declared with the @dataclass
decorator, specifying two attributes: name
of type string and age
of type integer.
To showcase the serialization process, we create an instance of the Person
class named person_instance
with specific values. The serialization is performed using json.dumps(person_instance, cls=DataClassEncoder)
, where the cls=DataClassEncoder
argument ensures the utilization of the custom encoder.
The resulting JSON-formatted string is printed with print(json_data)
, demonstrating the successful conversion of the dataclass
to a JSON representation.
Running this code would produce the following output:
This JSON string represents the data stored in the Person
dataclass
instance, showcasing the effectiveness of the custom JSON encoder in converting dataclasses
to a JSON format tailored to your needs.
In conclusion, the ability to convert dataclasses
to JSON using a custom encoder enhances the flexibility of your Python programs, enabling seamless integration with other systems and simplifying data storage in a widely supported format.
Custom Serialization Function
In the ever-evolving landscape of Python programming, dataclasses
have become an essential tool for creating structured and readable data models. As data interchange plays a crucial role in many applications, the ability to seamlessly convert dataclasses
to JSON format is of utmost importance.
This article delves into a powerful approach: custom serialization.
Custom serialization allows developers to tailor the process of converting dataclasses
to JSON, providing a flexible and controlled way to represent Python dataclass
objects in a format suitable for data exchange. We’ll explore a practical example, demonstrating how to achieve this using a custom serialization.
Code Example:
from dataclasses import dataclass, asdict, is_dataclass
import json
def serialize_dataclass(instance):
if is_dataclass(instance):
return asdict(instance)
return instance
@dataclass
class Person:
name: str
age: int
person_instance = Person(name="John", age=30)
json_data = json.dumps(person_instance, default=serialize_dataclass)
print(json_data)
In the provided code, we introduce a custom serialization function, serialize_dataclass
, designed to handle the conversion of dataclass
to dictionaries. This function begins by checking whether the given instance is a dataclass
using is_dataclass(instance)
.
If the check is affirmative, the function utilizes asdict(instance)
to transform the dataclass
into a dictionary. Importantly, the function includes a conditional check to ensure it exclusively attempts to serialize dataclasses
; if the instance is not a dataclass
, it is returned unchanged.
The dataclass
employed for this demonstration is named Person
and is defined using the @dataclass
decorator. This class features two attributes: name
of type string and age
of type integer.
To exemplify the serialization process, an instance of the Person
class, named person_instance
, is created with specific values. The actual serialization takes place with the use of json.dumps(person_instance, default=serialize_dataclass)
.
Here, the default=serialize_dataclass
argument ensures the incorporation of the custom serialization function during the JSON serialization process.
The resulting JSON-formatted string is then printed using print(json_data)
, showcasing the successful conversion of the dataclass
to a JSON representation with the assistance of the custom serialization function.
Running this code would produce the following output:
The output demonstrates the successful conversion of the Person
dataclass
instance to a JSON-formatted string. The custom serialization function provides a controlled and tailored approach to handling dataclass
instances during the serialization process, showcasing the effectiveness of this concept.
In conclusion, leveraging custom serialization methods offers a fine-grained way to control how dataclasses
are represented in JSON, providing developers with flexibility and control over the serialization process in their Python applications.
Best Practices for Dataclass
to JSON Conversion
Following best practices in converting dataclasses
JSON is essential for maintaining code readability, reliability, and compatibility across different Python environments. When converting a Python dataclass
to a JSON string, adhering to best practices ensures that the serialization process is both efficient and free from errors.
Import dataclass
Correctly
When converting a Python dataclass
to JSON, a fundamental best practice is to correctly import the dataclass
module using from dataclasses import dataclass
. Additionally, ensure to import field
from the dataclasses
module by using the statement dataclasses import field
.
These import statements allow you to utilize the @dataclass
decorator efficiently. With this in place, you can define your dataclass
, specifying attributes and types using annotations.
Utilize Type Annotations
For optimal dataclass
JSON conversion in Python, a key best practice is to leverage annotations for your dataclass
attributes. By importing the dataclass
module and using the @dataclass
decorator, you can succinctly define your dataclass
with clear type hints.
This not only enhances code readability but also guides the serialization process, ensuring the resulting JSON maintains a well-defined structure.
Date
and Time
Objects
When handling date and time objects in the context of converting a Python dataclass
to JSON, it is essential to import the appropriate modules and follow best practices.
If your dataclass
involves date or time attributes, import the necessary modules for these types, such as datetime
.
Use Default Value
The best practice for dataclass
to JSON conversion involves utilizing default values. By setting default values for data class attributes, you enhance flexibility in handling missing or optional fields during serialization, ensuring a more robust and predictable transformation.
Handle Optional Value
If your dataclass
involves attributes that can be optional, use the typing
module and import optional
to indicate this explicitly.
Nest Data Classes
If your data class includes attributes that are themselves instances of other data classes, leverage the power of nested data classes for a clean and organized structure.
Use asdict
for Entire JSON Object
By employing this practice, you simplify the conversion process, ensuring that the entire dataclass
structure is accurately reflected in the resulting JSON object. This approach is concise, readable, and aligns with the default behavior of asdict
, making it a straightforward and effective solution for dataclass
to JSON conversion in Python.
Handle Lists in Data Classes
By incorporating this best practice, you ensure that your import list within your data classes is correctly handled during the dataclass
to JSON conversion. This results in a clean and accurate representation of your data in the JSON format.
Maintain Python 3.7 and Above
By adhering to this best practice, you guarantee that your dataclass
to JSON conversion is compatible with the 3.7 version and benefits from the advancements in annotations and dataclass
support introduced in later Python versions. This ensures a simple, concise, and forward-compatible approach to handling data classes in your Python applications.
Testing and Error Handling
Thoroughly test your dataclass
to JSON conversion, considering various scenarios and edge cases. By thoroughly testing your conversion logic and incorporating error handling mechanisms, you ensure that your dataclass
to JSON conversion is robust and capable of handling unexpected situations, leading to more reliable and stable code.
Documentation and Code Comments
By incorporating thorough documentation and code comments, you make a more transparent and accessible codebase. Clearly explain the purpose of your data classes, their attributes, and any specific considerations for JSON conversion.
This is crucial for both understanding the dataclass
to JSON conversion logic and facilitating collaboration among developers.
Troubleshooting Common Issues
Troubleshooting common issues in converting dataclasses
to JSON is crucial for ensuring the smooth integration of these features in Python applications. The process of transforming a dataclass
into a JSON object involves handling various elements, such as default value, datetime
object, and nested structure.
Addressing potential errors during this conversion ensures that the resulting JSON output accurately represents the original data class. Additionally, considering factors like Python version compatibility (3.7 and above) and the use of appropriate libraries helps maintain a reliable and data-to-JSON transformation that is free from errors.
By proactively troubleshooting and resolving these issues, developers can create robust and efficient code, contributing to a seamless data interchange between Python object and JSON representation.
Install the dataclasses-json
Package
When a ModuleNotFoundError
appears, it indicates that you have not installed dataclasses-json
package. To resolve this error, you need to install the dataclasses_json
package.
You can install it using the following command in your terminal or command prompt:
pip install dataclasses-json
Once the installation is complete, you should be able to run the code without encountering the ModuleNotFoundError
related to dataclasses_json
.
Importing Necessary Modules
The first step is to ensure that the required modules are imported correctly. Importing the dataclasses
module is essential for creating dataclasses
, and the import json
module is necessary for handling JSON-related operations.
Developers must also import field
from the dataclasses
module to specify field attributes.
from dataclasses import dataclass, field
import json
Handling DateTime
Objects
When working with dataclasses
that include datetime
object, such as dates, special care is needed. It’s crucial to import the date
class from the datetime
module and consider the serialization of these objects properly.
from datetime import date
Dealing With Optional Fields
In some scenarios, dataclass
fields might be optional. To address this, developers should import the Optional
class from the typing
module and appropriately annotate the fields.
from typing import Optional
Nested Dataclasses
When dealing with nested dataclasses
, issues may arise in preserving the hierarchical structure during the conversion to JSON. Developers should ensure that they are appropriately defined and handled to avoid unexpected errors.
Default Values and the default
Function
Setting default values for fields in dataclasses
can lead to unexpected behavior when converting to JSON. Developers should be cautious about relying on the default behavior and consider using the default
argument in the json.dumps
function to handle specific scenarios.
Snake Case Conversion
Dataclass
fields are typically defined using this, while JSON keys often use camel case. Developers may need to implement a custom JSON encoder class or use existing libraries to ensure proper conversion between the two naming conventions.
Union Type and Supported Type
When using union types in dataclasses
, it’s essential to consider the supported types for JSON serialization. Certain types may not be directly JSON serializable, requiring additional handling or conversion logic.
Full Support for Python 3.7 and Above
Ensure that the codebase and libraries used are compatible with 3.7 and later versions, as the dataclasses
feature is available from 3.7 onwards.
Conclusion
Converting a Python dataclass
to JSON involves a comprehensive understanding of Python classes and the significance of JSON serialization. While the basics can be achieved using the native json
module, leveraging third-party libraries, such as the dataclasses_json
package, enhances the process.
Best practices include considering default values, handling various data types, and adhering to conventions for optimal dataclass
-to-JSON conversion. Troubleshooting common issues ensures a seamless transition. Through a brief example, we illustrated the simplicity of the conversion process.
Whether dealing with primitive types or more complex data, employing libraries like dataclasses_json
streamlines the conversion, providing a robust and efficient way to serialize Python object into JSON format.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn