How to Convert CSV Into Dictionary in Python
-
Use the
csv
Module to Convert CSV File to Dictionary in Python - Use Pandas to Convert CSV File to Dictionary in Python
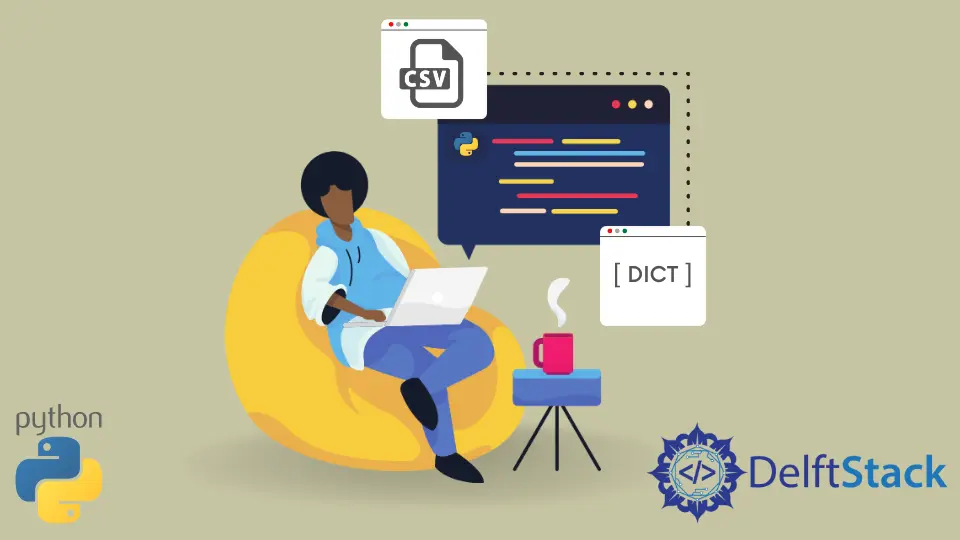
This tutorial will introduce how to convert a csv file into a dictionary in Python wherein the csv file contains two columns. The first column contains the keys, and the second column contains the values.
In this tutorial, the content for the sample CSV is shown below.
The first column contains identifiers that will be used as keys and the second column are the values.
Use the csv
Module to Convert CSV File to Dictionary in Python
Python has a csv
module that contains all sorts of utility functions to manipulate CSV files like conversion, reading, writing, and insertion. To convert a CSV File into a dictionary, open the CSV file and read it into a variable using the csv
function reader()
, which will store the file into a Python object.
Afterward, use dictionary comprehension to convert the CSV object into a dictionary by iterating the reader
object and accessing its first two rows as the dictionary’s key-value pair.
import csv
dict_from_csv = {}
with open("csv_file.csv", mode="r") as inp:
reader = csv.reader(inp)
dict_from_csv = {rows[0]: rows[1] for rows in reader}
print(dict_from_csv)
Output:
{'fruit': 'apple', 'vegetable': 'tomato', 'mammal': 'rabbit', 'fish': 'clownfish', 'bird': 'crow'}
Use Pandas to Convert CSV File to Dictionary in Python
Another way to convert a CSV file to a Python dictionary is to use the Pandas module, which contains data manipulation tools for CSV files.
After importing pandas, make use of its built-in function read_csv()
with a few parameters to specify the csv file format. After calling read_csv()
, convert the result to a dictionary using the built-in pandas function to_dict()
.
import pandas as pd
dict_from_csv = pd.read_csv(
"csv_file.csv", header=None, index_col=0, squeeze=True
).to_dict()
print(dict_from_csv)
The header
parameter specifies that the headers are explicitly passed or declared by another parameter.
index_col
specifies which column is used as the labels for the DataFrame
object that the read_csv()
function returns. In this case, the first column of index 0
is the labels.
Lastly, the squeeze
parameter defines if the data contains only one column for values. In this case, there is only one column since the first column is used as the index column or the labels.
Output:
{'fruit': 'apple', 'vegetable': 'tomato', 'mammal': 'rabbit', 'fish': 'clownfish', 'bird': 'crow'}
No module named 'pandas'
, then make sure that pandas
is installed in your local machine using pip install pandas
or pip3 install pandas
if you’re running Python 3.Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python