Pandas read_csv() Function
-
Syntax of
pandas.read_csv()
: -
Example Codes: Pandas Read
CSV
File Usingpandas.read_csv()
Function -
Example Codes:Set
usecols
Parameter inpandas.read_csv()
Function -
Example Codes:
pandas.read_csv()
Function With Header -
Example Codes:
pandas.read_csv()
Function With Skipping Rows
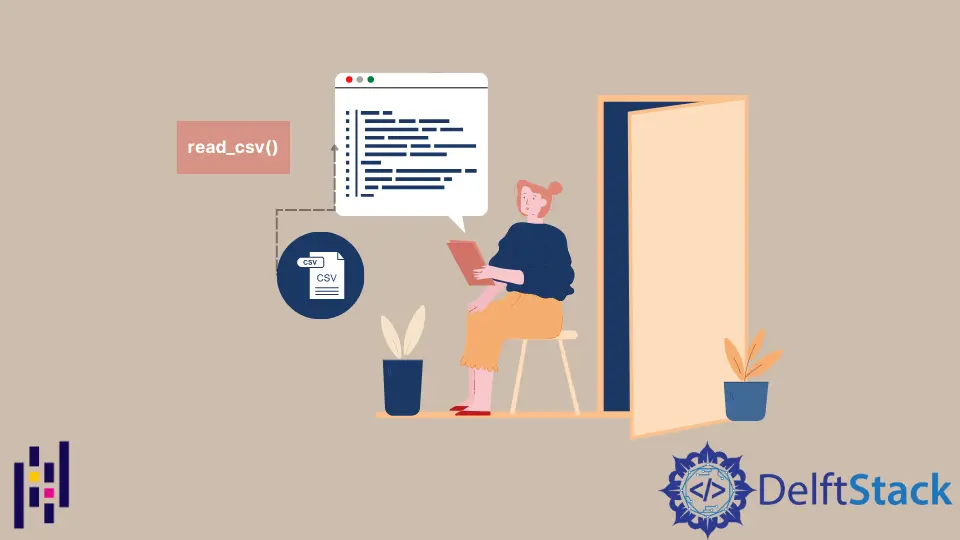
ThePandas read_csv()
method reads the specified comma-separated values (CSV
) file into DataFrame
.
Syntax of pandas.read_csv()
:
pandas.read_csv(filepath_or_buffer: Union[str, pathlib.Path, IO[~ AnyStr]],
sep=',',
delimiter=None,
header='infer',
names=None,
index_col=None,
usecols=None,
squeeze=False,
prefix=None,
mangle_dupe_cols=True,
dtype=None,
engine=None,
converters=None,
true_values=None,
false_values=None,
skipinitialspace=False,
skiprows=None,
skipfooter=0,
nrows=None,
na_values=None,
keep_default_na=True,
na_filter=True,
verbose=False,
skip_blank_lines=True,
parse_dates=False,
infer_datetime_format=False,
keep_date_col=False,
date_parser=None,
dayfirst=False,
cache_dates=True,
iterator=False,
chunksize=None,
compression='infer',
thousands=None,
decimal: str='.',
lineterminator=None,
quotechar='"',
quoting=0,
doublequote=True,
escapechar=None,
comment=None,
encoding=None,
dialect=None,
error_bad_lines=True,
warn_bad_lines=True,
delim_whitespace=False,
low_memory=True,
memory_map=False,
float_precision=None)
Parameters
filepath_or_buffer |
location of csv file to be imported |
delimiter |
Delimiter to use for parsing content of csv file |
usecols |
The column names only to be included while forming DataFrame from the csv file. |
header |
which row/rows to use as the column names of header |
squeeze |
returns Pandas series if the parsed data only contains one column. |
skiprows |
which row/rows to skip |
Return
Dataframe
formed from CSV
file with labeled axes.
Example Codes: Pandas Read CSV
File Using pandas.read_csv()
Function
import pandas as pd
df = pd.read_csv("dataset.csv")
print(df)
Output:
Country Item Type Sales Channel Order Priority
0 Tuvalu Baby Food Offline H
1 East Timor Meat Online L
2 Norway Baby Food Online L
3 Portugal Baby Food Online H
4 Honduras Snacks Online L
5 New Zealand Fruits Online H
6 Moldova Personal Care Online L
This method loads the CSV
file into the DataFrame
. Here, we can use both the absolute and relative paths to provide a file path as an argument to the pandas.read_csv()
function.
In this case, the dataset.csv
is in the same directory as the program file; this means that you can use the name of the CSV
file as a file path.
Example Codes:Set usecols
Parameter in pandas.read_csv()
Function
import pandas as pd
df = pd.read_csv("dataset.csv",usecols=["Country","Sales Channel","Order Priority"])
print(df)
Output:
Country Sales Channel Order Priority
0 Tuvalu Offline H
1 East Timor Online L
2 Norway Online L
3 Portugal Online H
4 Honduras Online L
5 New Zealand Online H
6 Moldova Online L
In this example, it loads the CSV
file into the DataFrame
by including only the specified columns in the usecols
parameter.
The columns Country
, Sales Channel
, and Order Priority
are only passed as parameters, so they are only included in the DataFrame
.
Example Codes: pandas.read_csv()
Function With Header
import pandas as pd
df = pd.read_csv("dataset.csv",header=1)
print(df)
Output:
Tuvalu Baby Food Offline H
0 East Timor Meat Online L
1 Norway Baby Food Online L
2 Portugal Baby Food Online H
3 Honduras Snacks Online L
4 New Zealand Fruits Online H
5 Moldova Personal Care Online L
This process loads the CSV
file into the DataFrame
by setting the first row as a header.
Here, the first row elements serve as the column names for the entire DataFrame
.
Example Codes: pandas.read_csv()
Function With Skipping Rows
import pandas as pd
df = pd.read_csv("dataset.csv",skiprows=3)
print(df)
Output:
Norway Baby Food Online L
0 Portugal Baby Food Online H
1 Honduras Snacks Online L
2 New Zealand Fruits Online H
3 Moldova Personal Care Online L
This procedure loads the CSV
file into the DataFrame
by skipping the first 3 rows.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn