How to Copy Object in Python
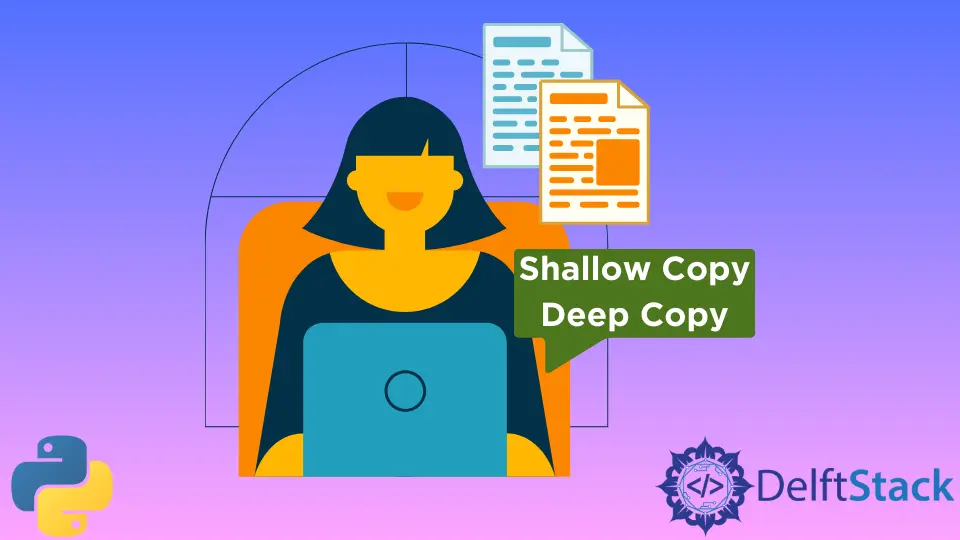
In Python, the assignment statements do not have the power to copy objects but just generate a new variable that shares the reference to that of the original object.
Copying is very useful in programming and is mainly required to edit one copy’s code without damaging the other one.
This tutorial will discuss different methods to copy an object in Python.
Use Shallow Copy to Copy an Object in Python
The copy
module needs to be imported to use the shallow copy operation. The copy
module supplies the programmers with the ability to use generic deep and shallow copy operations.
A shallow copy basically creates a new collecting object that stores the references of the child objects found in the original elements. This process is not recursive and, therefore, does not create any copies of the child objects.
When a shallow copy operation occurs, a reference is copied to the new object. It means that if any change is made to the copy of the object, then these changes will also reflect in the original object.
The following code uses the Shallow copy
operation to copy an object in Python.
import copy
# the original list
ol = [2, 4, [1, 8], 6, 8]
# using copy to shallow copy
nl = copy.copy(ol)
print("Original list before shallow copy")
for x in range(0, len(ol)):
print(ol[x], end=" ")
print("\r")
# modfying the new list
nl[2][0] = 9
print("Original list after shallow copy")
for x in range(0, len(ol)):
print(ol[x], end=" ")
Output:
Original list before shallow copy
2 4 [1, 8] 6 8
Original list after shallow copy
2 4 [9, 8] 6 8
As mentioned earlier, no copies of the child process are created in this operation. Therefore, it can be said that shallow copy is not entirely self-reliant and depends on the original object.
Use Deep Copy to Copy an Object in Python
We need to import the copy
module to the Python code to use both the deep and shallow copy operations.
In the deep copy operation, the copying process is always recursively occurring. The deep copy operation first creates a new collecting object and then adds copies of the child objects found in the original elements.
Basically, in the deep copy
process, a copy of the original object is passed to the new collecting object. Therefore, if any change is made to the copy of the object, these changes will not reflect in the original object. To implement this deep copy operation, we use the deepcopy()
function.
The following code uses the deepcopy()
function to implement the deep copy operation in Python.
import copy
# original list
ol = [2, 4, [1, 8], 6, 8]
# use deepcopy() to deep copy
nl = copy.deepcopy(ol)
# original elements of list
print("Original list before deep copying")
for x in range(0, len(ol)):
print(ol[x], end=" ")
print("\r")
# adding and element to new list
nl[2][0] = 9
# The second list after changes
print("The new list after modifications ")
for x in range(0, len(ol)):
print(nl[x], end=" ")
print("\r")
print("Original list after deep copying")
for x in range(0, len(ol)):
print(ol[x], end=" ")
Output:
Original list before deep copying
2 4 [1, 8] 6 8
The new list after modifications
2 4 [9, 8] 6 8
Original list after deep copying
2 4 [1, 8] 6 8
As dictated in the above sentences, a deep copy can clone the child objects recursively, which means that it is self-reliant and does not depend on the original object.
The only drawback to creating a deep copy operation is that it is comparatively slower and takes more time to achieve than a shallow copy operation.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn