Python 複製物件
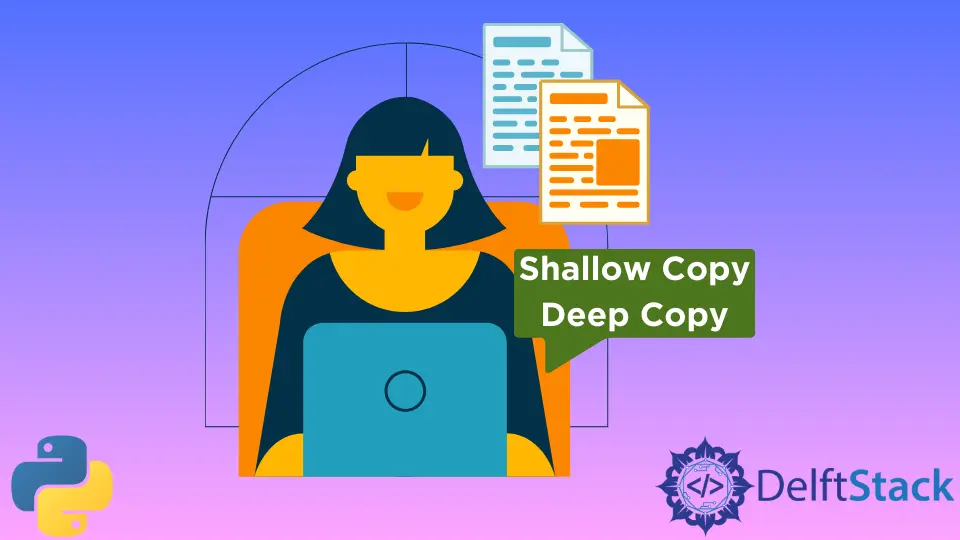
在 Python 中,賦值語句不能複製物件,而只是生成一個新變數,該變數共享對原始物件的引用。
複製在程式設計中非常有用,主要需要在不損壞另一個副本的情況下編輯一個副本的程式碼。
本教程將討論在 Python 中複製物件的不同方法。
在 Python 中使用淺拷貝複製物件
需要匯入 copy
模組才能使用淺拷貝操作。copy
模組為程式設計師提供了使用通用深拷貝和淺拷貝操作的能力。
淺拷貝基本上會建立一個新的收集物件,該物件儲存在原始元素中找到的子物件的引用。此過程不是遞迴的,因此不會建立子物件的任何副本。
當發生淺複製操作時,將引用複製到新物件。這意味著如果對物件的副本進行任何更改,那麼這些更改也會反映在原始物件中。
以下程式碼使用 Shallow copy
操作在 Python 中複製物件。
import copy
# the original list
ol = [2, 4, [1, 8], 6, 8]
# using copy to shallow copy
nl = copy.copy(ol)
print("Original list before shallow copy")
for x in range(0, len(ol)):
print(ol[x], end=" ")
print("\r")
# modfying the new list
nl[2][0] = 9
print("Original list after shallow copy")
for x in range(0, len(ol)):
print(ol[x], end=" ")
輸出:
Original list before shallow copy
2 4 [1, 8] 6 8
Original list after shallow copy
2 4 [9, 8] 6 8
如前所述,在此操作中不會建立子程序的副本。因此,可以說淺拷貝並不是完全自力更生,而是依賴於原始物件。
在 Python 中使用 Deep Copy 複製物件
我們需要將 copy
模組匯入 Python 程式碼以使用深拷貝和淺拷貝操作。
在深拷貝操作中,拷貝過程總是遞迴發生的。深度複製操作首先建立一個新的收集物件,然後新增在原始元素中找到的子物件的副本。
基本上,在深度複製
過程中,原始物件的副本被傳遞給新的收集物件。因此,如果對物件的副本進行任何更改,這些更改將不會反映在原始物件中。為了實現這種深度複製操作,我們使用 deepcopy()
函式。
下面的程式碼使用 deepcopy()
函式在 Python 中實現深度複製操作。
import copy
# original list
ol = [2, 4, [1, 8], 6, 8]
# use deepcopy() to deep copy
nl = copy.deepcopy(ol)
# original elements of list
print("Original list before deep copying")
for x in range(0, len(ol)):
print(ol[x], end=" ")
print("\r")
# adding and element to new list
nl[2][0] = 9
# The second list after changes
print("The new list after modifications ")
for x in range(0, len(ol)):
print(nl[x], end=" ")
print("\r")
print("Original list after deep copying")
for x in range(0, len(ol)):
print(ol[x], end=" ")
輸出:
Original list before deep copying
2 4 [1, 8] 6 8
The new list after modifications
2 4 [9, 8] 6 8
Original list after deep copying
2 4 [1, 8] 6 8
如上句所述,深拷貝可以遞迴地克隆子物件,這意味著它是自力更生的,不依賴於原始物件。
建立深拷貝操作的唯一缺點是它比淺拷貝操作相對較慢並且需要更多時間來實現。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn