How to Call a Function From String Name in Python
-
Use
getattr()
to Call a Function From a String in Python -
Use
locals()
andglobals()
to Call a Function From a String in Python -
Use
eval()
to Call a Function From a String in Python - Use Dictionary Mapping to Call a Function From a String in Python
- Conclusion
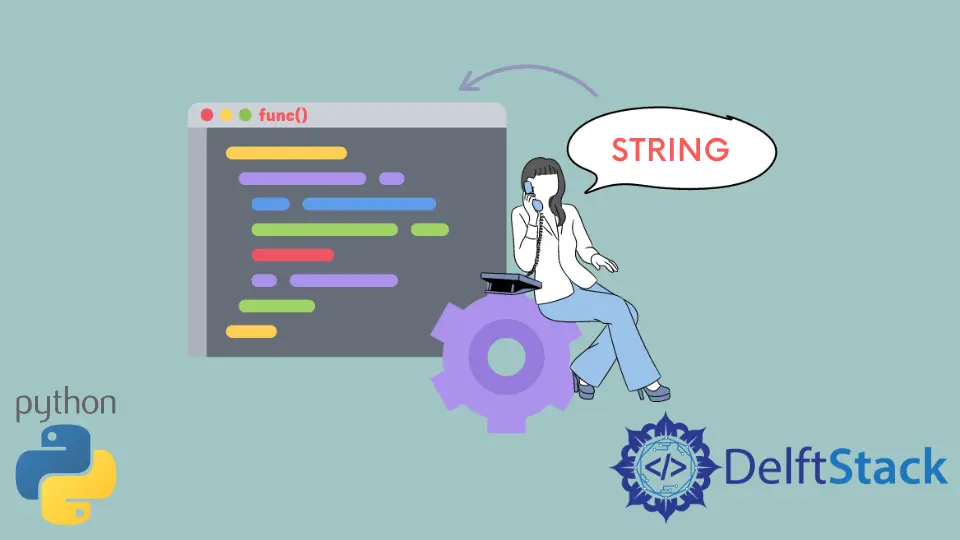
This tutorial is crafted to guide you through the intriguing process of calling a function using its name in string format in Python.
Such a capability is not just a fascinating aspect of Python’s versatility but also a powerful tool in various programming scenarios. From handling dynamic function calls in a modular application to implementing command pattern designs, the techniques covered here open up a world of possibilities.
Use getattr()
to Call a Function From a String in Python
The getattr()
function in Python is primarily used to retrieve the value of an attribute from an object. However, since functions in Python are also objects, getattr()
can be employed to reference a function dynamically using its name as a string.
Let’s look at a concrete example.
class MathOperations:
def add(x, y):
return x + y
def multiply(x, y):
return x * y
# Function name as a string
function_name = "add"
x, y = 10, 5
# Using getattr to get the function
operation = getattr(MathOperations, function_name, None)
# Check if the function exists and call it
if operation:
result = operation(x, y)
print(f"The result of {function_name} is: {result}")
else:
print(f"Function {function_name} not found.")
We define a class MathOperations
with two methods, add
and multiply
.
We have a variable function_name
holding the name of the function we want to call as a string.
The getattr()
function is used to retrieve the function object from the MathOperations
class using function_name
.
We check if the function exists (i.e., operation
is not None
). If it does, we call the function with arguments and print the result.
If the function does not exist, a message is printed indicating the same.
Output:
Use locals()
and globals()
to Call a Function From a String in Python
Another way to call a function from a string is by using the built-in functions locals()
and globals
. These two functions return a Python dictionary that represents the current symbol table of the given source code.
The difference between the two functions is the namespace. As the names indicate, locals()
returns a dictionary including local variables, and globals()
returns a dictionary including local variables. Function names are also returned in the format of the string.
Let’s put these methods into an example.
def add(x, y):
return x + y
def multiply(x, y):
return x * y
def call_function(func_name, *args):
# Attempt to fetch the function from local scope
func = locals().get(func_name)
if not func:
# Attempt to fetch from global scope
func = globals().get(func_name)
if func:
return func(*args)
return None
# Test the functions
print(call_function("add", 5, 3)) # Local function call
print(call_function("multiply", 4, 2)) # Global function call
We start by defining two functions: add
and multiply
. These functions will be our targets for dynamic calling.
Next, we craft a function named call_function
. This function is designed to accept a string func_name
, which represents the name of the function we wish to call, along with *args
, a list of arguments to be passed to that function.
The process within call_function
begins by searching for the function within the local scope. We achieve this using locals().get(func_name)
.
However, if the function isn’t found in the local scope, our next step is to search within the global scope. This is where globals().get(func_name)
comes into play.
It expands our search to the entire global scope of the program, ensuring that no stone is left unturned.
Finally, if we successfully locate the function, we call it using the arguments provided in *args
. If the function is not found in either scope, our call_function
gracefully returns None
, indicating the absence of the specified function.
Output:
Use eval()
to Call a Function From a String in Python
One of the more powerful, albeit controversial, features of Python is its ability to evaluate and execute Python expressions from a string-based input using the eval()
function. This capability extends to calling functions dynamically based on string values, which can be incredibly useful in certain programming scenarios.
Let’s create a simple example to illustrate the use of eval()
.
def add(x, y):
return x + y
def subtract(x, y):
return x - y
# Function name and arguments in string form
expression = "add(5, 3)"
# Use eval to call the function
result = eval(expression)
print(f"Result of {expression}: {result}")
We define two functions, add
and subtract
.
We create a string, expression
, which contains the name of the function to call (add
) and its arguments (5, 3
).
We then use eval(expression)
to evaluate this string. Python parses the string, identifies the function to be called along with its arguments, and executes it.
Finally, we print the result.
Output:
Use Dictionary Mapping to Call a Function From a String in Python
In Python, the flexibility of data structures like dictionaries can be harnessed to create mappings between strings and functions. This method is an efficient and safe way to dynamically call functions based on string names.
Let’s create a simple example demonstrating this concept.
def add(x, y):
return x + y
def subtract(x, y):
return x - y
# Creating a dictionary to map function names to function objects
function_map = {"add": add, "subtract": subtract}
# Function name as a string
func_name = "add"
# Arguments for the function
args = (5, 3)
# Calling the function from the dictionary
if func_name in function_map:
result = function_map[func_name](*args)
print(f"Result of {func_name}: {result}")
else:
print(f"Function '{func_name}' not found.")
We define two simple functions, add
and subtract
.
We then create a dictionary named function_map
, where the keys are string representations of the function names, and the values are the actual function objects.
We store the desired function name as a string in func_name
and arguments in a tuple args
.
To call the function, we first check if func_name
exists in function_map
. If it does, we retrieve the function using function_map[func_name]
and call it with the unpacked arguments *args
.
If the function name is not found in the map, we print an error message.
Output:
Conclusion
Throughout this tutorial, we have explored several methods to call functions dynamically using string names in Python. Each method, whether it’s the object-oriented approach of getattr()
, the scope-aware usage of locals()
and globals()
, the direct yet cautious application of eval()
, or the structured and safe strategy of dictionary mapping, serves a unique purpose and fits different use cases.
Understanding these methods not only enhances your Python coding toolkit but also opens up creative ways to design and structure your code. As we conclude, remember that the choice of method largely depends on the specific requirements and constraints of your project, and each approach offers a blend of power, safety, and flexibility in its own right.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python Function
- How to Exit a Function in Python
- Optional Arguments in Python
- How to Fit a Step Function in Python
- Built-In Identity Function in Python
- Arguments in the main() Function in Python
- Python Functools Partial Function