在 Python 中根據函式的字串名稱呼叫函式
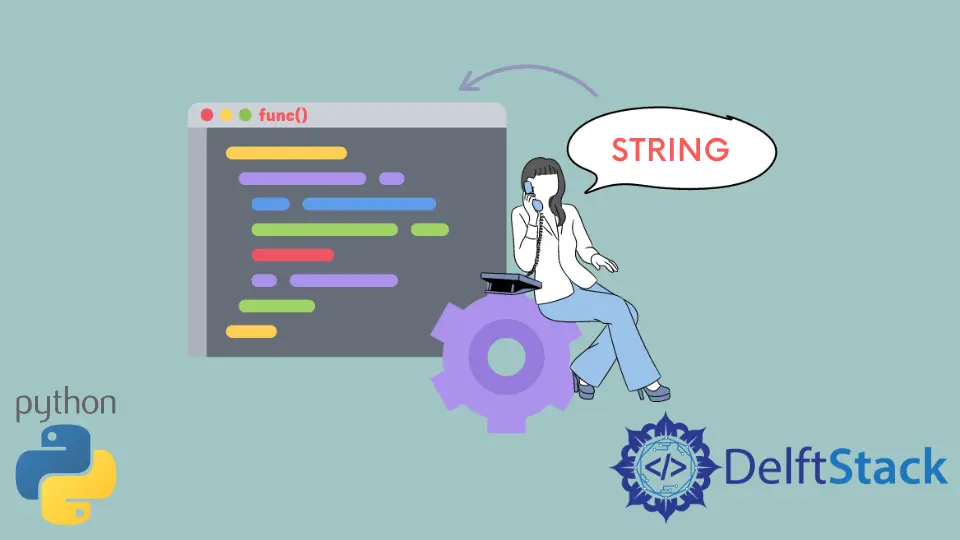
本教程將介紹如何在 Python 中根據函式的字串名稱呼叫函式。
這個問題的用例是將一個模組或類中的函式賦值到一個變數中,不管它有什麼用途。
在 Python 中使用 getattr()
將一個函式賦值到一個變數中
函式 getattr()
從物件或模組中返回一個屬性值。這個函式有兩個必要的引數,第一個引數是物件或模組的名稱,第二個引數是一個包含屬性名稱的字串值。
有關的屬性可以是一個變數、一個函式或一個子類的形式。
假設我們有一個名為 User
的類,具有給定的屬性:
# Filename: user.py
class User:
name = "John"
age = 33
def doSomething():
print(name + " did something.")
現在,我們想把屬性函式 doSomething()
儲存到一個方法中並呼叫它。要做到這一點,我們將使用 getattr()
函式。
from user import User as user
doSomething = getattr(user, "doSomething")
doSomething(user)
輸出:
John did something.
現在,函式 user.doSomething()
被封裝在變數 doSomething
中。這樣一來,呼叫函式時就不需要指定物件 user
了。
在 Python 中使用 locals()
和 globals()
從一個字串中呼叫一個函式
另一種從字串呼叫函式的方法是使用內建函式 locals()
和 globals
。這兩個函式返回一個 Python 字典,表示當前給定原始碼的符號表。
這兩個函式的區別在於名稱空間。如名稱所示,locals()
返回包括區域性變數的字典,globals()
返回包括區域性變數的字典。函式名也會以字串的格式返回。
讓我們將這些方法作為示例。宣告兩個隨機函式,並使用兩個內建函式呼叫它。
def myFunc():
print("This is a function.")
def myFunc2():
print("This is another function.")
locals()["myFunc"]()
globals()["myFunc2"]()
輸出:
This is a function.
This is another function.
總而言之,根據字串來呼叫一個函式,需要使用 getattr()
、locals()
和 globals()
等函式。getattr()
會要求你知道函式所在的物件或模組,而 locals()
和 globals()
會在自己的範圍內定位函式。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn相關文章 - Python Function
- 在 Python 中退出函式
- Python 中的可選引數
- 在 Python 中模仿 ode45() 函式
- Python 中的 zip() 函式
- Python 中的 filter() 方法和 Lambda 函式
- Python 中的 maketrans 函式