How to Fix Python OverflowError: Python Int Too Large to Convert to C Long
-
the
OverflowError: Python int too large to convert to C long
in Python -
Fix the
OverflowError: Python int too large to convert to C long
in Python
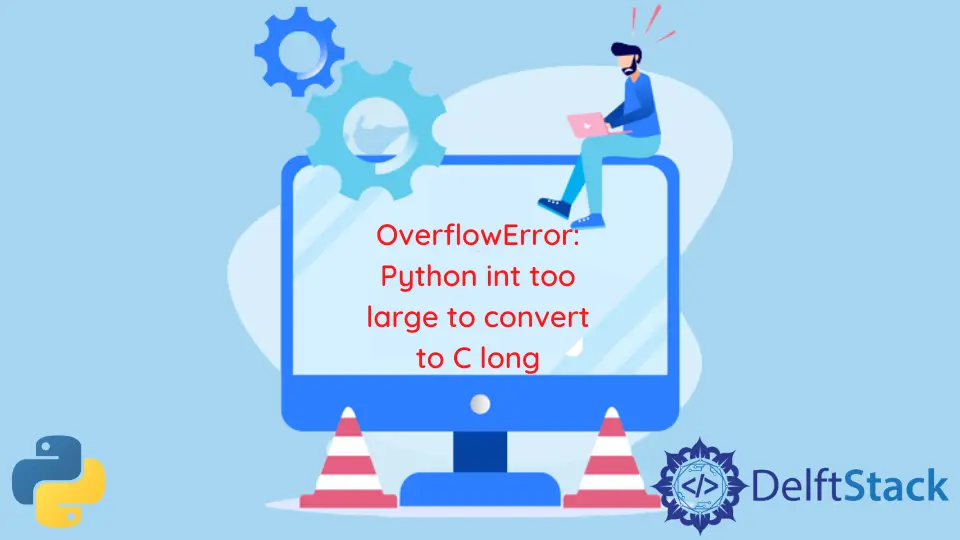
This tutorial will demonstrate the OverflowError: python int too large to convert to c long
error in Python.
the OverflowError: Python int too large to convert to C long
in Python
An OverflowError
is raised in Python when an arithmetic result exceeds the given limit of the data type. We encounter this specified error because we try to operate with an integer’s value more than the given range.
Code:
import numpy as np
arr = np.zeros((1, 2), dtype=int)
a = [6580225610007]
arr[0] = a
Output:
OverflowError: Python int too large to convert to C long
The above example creates an array of type int
. We try to store a list containing an integer greater than the int
type range.
The maximum size can also be checked using a constant from the sys
library. This constant is available as sys.maxsize
.
Fix the OverflowError: Python int too large to convert to C long
in Python
To avoid this error, we must be mindful of the maximum range we can use with the int
type. A fix exists, especially in this example regarding a numpy array.
The int
type was equal to the long int
type of the C language and was changed in Python 3, and the int
type was converted to an arbitrary precision type.
However, the numpy
library still uses it as declared in Python 2. The long int
is usually platform-dependent, but for windows, it is always 32-bit.
To fix this error, we can use other types that are not platform-dependent. The most common alternative is the np.int64
type, and we specify this in the array with the dtype
parameter.
Code:
import numpy as np
arr = np.zeros((1, 2), dtype=np.int64)
a = [6580225610007]
arr[0] = a
print(arr)
Output:
[[6580225610007 6580225610007]]
The above example shows that the error is resolved using the alternative type.
Another way can be to use a try
and except
block. These two blocks are used to work around exceptions in Python.
The code in the except
block is executed if an exception is thrown in the try
block.
Code:
import numpy as np
arr = np.zeros((1, 2), dtype=int)
a = [6580225610007]
try:
arr[0] = a
except:
print("Error in code")
Output:
Error in code
The above example avoids the error using the try
and except
blocks. Remember that this is not a fix to the given error but a method to work around it.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python