Python OverflowError: Python Int が大きすぎて C Long に変換できません
-
Python の
OverflowError: Python int too large to convert to C long
-
Python の
OverflowError: Python int too large to convert to C long
を修正
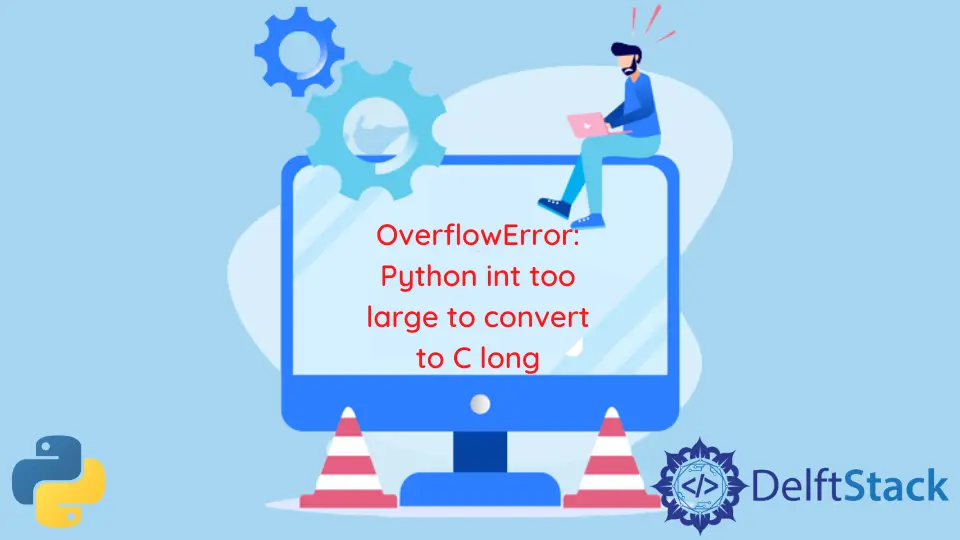
このチュートリアルでは、Python の OverflowError: python int too large to convert to c long
エラーについて説明します。
Python の OverflowError: Python int too large to convert to C long
算術結果が指定されたデータ型の制限を超えると、Python では OverflowError
が発生します。 指定された範囲を超える整数値で操作しようとするため、この特定のエラーが発生します。
コード:
import numpy as np
arr = np.zeros((1, 2), dtype=int)
a = [6580225610007]
arr[0] = a
出力:
OverflowError: Python int too large to convert to C long
上記の例は、int
型の配列を作成します。 int
型の範囲より大きい整数を含むリストを格納しようとします。
最大サイズは、sys
ライブラリの定数を使用してチェックすることもできます。 この定数は sys.maxsize
として利用できます。
Python の OverflowError: Python int too large to convert to C long
を修正
このエラーを回避するには、int
型で使用できる最大範囲に注意する必要があります。 特にnumpy配列に関するこの例では、修正が存在します。
int
型は C 言語の long int
型と同等であり、Python 3 で変更され、int
型は任意精度の型に変換されました。
ただし、numpy
ライブラリは Python 2 で宣言されているとおりに使用します。long int
は通常、プラットフォームに依存しますが、Windows の場合は常に 32 ビットです。
このエラーを修正するには、プラットフォームに依存しない他の型を使用できます。 最も一般的な代替手段は np.int64
型で、これを配列で dtype
パラメータで指定します。
コード:
import numpy as np
arr = np.zeros((1, 2), dtype=np.int64)
a = [6580225610007]
arr[0] = a
print(arr)
出力:
[[6580225610007 6580225610007]]
上記の例は、代替タイプを使用してエラーが解決されることを示しています。
もう 1つの方法は、try
および except
ブロックを使用することです。 これら 2つのブロックは、Python で例外を回避するために使用されます。
try
ブロックで例外がスローされた場合、except
ブロックのコードが実行されます。
コード:
import numpy as np
arr = np.zeros((1, 2), dtype=int)
a = [6580225610007]
try:
arr[0] = a
except:
print("Error in code")
出力:
Error in code
上記の例では、try
および except
ブロックを使用してエラーを回避しています。 これは、特定のエラーの修正ではなく、それを回避する方法であることに注意してください。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn関連記事 - Python Error
- AttributeError の解決: 'list' オブジェクト属性 'append' は読み取り専用です
- AttributeError の解決: Python で 'Nonetype' オブジェクトに属性 'Group' がありません
- AttributeError: 'generator' オブジェクトに Python の 'next' 属性がありません
- AttributeError: 'numpy.ndarray' オブジェクトに Python の 'Append' 属性がありません
- AttributeError: Int オブジェクトに属性がありません
- AttributeError: Python で 'Dict' オブジェクトに属性 'Append' がありません