AttributeError の解決: Python で 'Nonetype' オブジェクトに属性 'Group' がありません
-
Python での
AttributeError: 'NoneType' object has no attribute 'group'
の原因 -
try-except
を使用して Python でAttributeError: 'NoneType' object has no attribute 'group'
を解決する -
if-else
を使用して Python でAttributeError: 'NoneType' object has no attribute 'group'
を解決する
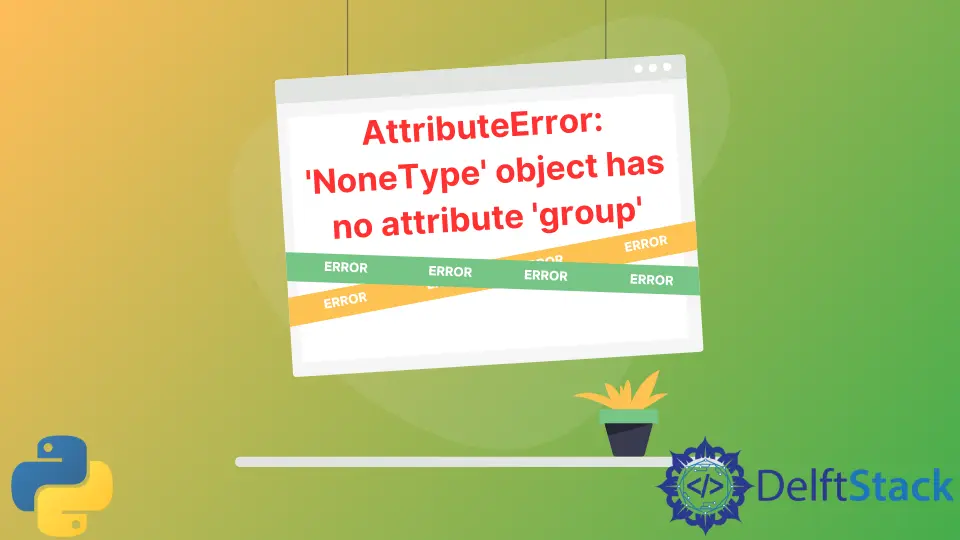
Python 正規表現 (regex) は、特殊文字またはパターンの文字列を照合して抽出します。 Python では、正規表現が指定された文字列と一致しない場合、正規表現 AttributeError: 'NoneType' object has no attribute 'group'
が発生します。
この記事では、このタイプのエラーに対する可能な解決策を見ていきます。
Python での AttributeError: 'NoneType' object has no attribute 'group'
の原因
クラスまたはデータ型を定義するときはいつでも、そのクラスに関連付けられた属性にアクセスできます。 しかし、定義したクラスが所有していないオブジェクトの属性またはプロパティにアクセスするとします。
その場合、'NoneType' オブジェクトに属性 'group' がない
という AttributeError
が発生します。 NoneType
は、None
値を含むオブジェクトを参照します。
このタイプのエラーは、最初に変数を none に設定した場合にも発生します。 次のプログラムは、単語の先頭にある大文字の F
を検索するプログラムです。
コード例:
# Python 3.x
import re
a = "programmig is Fun"
for i in a.split():
b = re.match(r"\bF\w+", i)
print(b.group())
出力:
#Python 3.x
AttributeError Traceback (most recent call last)
C:\Users\LAIQSH~1\AppData\Local\Temp/ipykernel_2368/987386650.py in <module>
3 for i in a.split():
4 b=re.match(r"\bF\w+", i)
----> 5 print(b.group())
AttributeError: 'NoneType' object has no attribute 'group'
このエラーは、正規表現が最初の反復で文字列内の指定された文字と一致しないために発生します。 したがって、group()
にアクセスすると、コンパイラは AttributeError
を表示します。これは、None 型のオブジェクトに属しているためです。
try-except
を使用して Python で AttributeError: 'NoneType' object has no attribute 'group'
を解決する
このエラーを解消する 1つの方法は、コードで例外処理を使用することです。 このようにして、except
ブロックがエラーを処理します。
前のプログラムを考えてみましょう。次のように try-except
ブロックを追加します。
コード例:
# Python 3.x
import re
a = "programmig is Fun"
for i in a.split():
b = re.match(r"\bF\w+", i)
try:
print(b.group())
except AttributeError:
continue
出力:
#Python 3.x
Fun
これで、プログラムが正常に動作することがわかりました。 continue
キーワードは、b
が何も返さない場所をスキップするためにここで使用され、次の繰り返しにジャンプし、F
で始まる単語を検索します。
したがって、Fun
という用語が出力に表示されます。
if-else
を使用して Python で AttributeError: 'NoneType' object has no attribute 'group'
を解決する
'NoneType' object has no attribute 'group'
エラーを回避するもう 1つの簡単な解決策は、プログラムで if-else
ステートメントを使用することです。 次のプログラムは、文字列内の 1 から 5 までの数字をチェックします。
正規表現に一致する番号がないため、AttributeError
になります。 しかし、if-else
ブロックを使用すると、エラーを回避できます。
条件が満たされない場合、else
ブロック内のステートメントは、一致するものが見つからないときに実行されます。
# Python 3.x
import re
a = "foo bar 678 baz"
x = r".* ([1-5]+) .*"
matches = re.match(x, a)
if matches:
print(matches.group(1))
else:
print("No matches!")
出力:
#Python 3.x
No matches!
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn