OpenCV Threshold
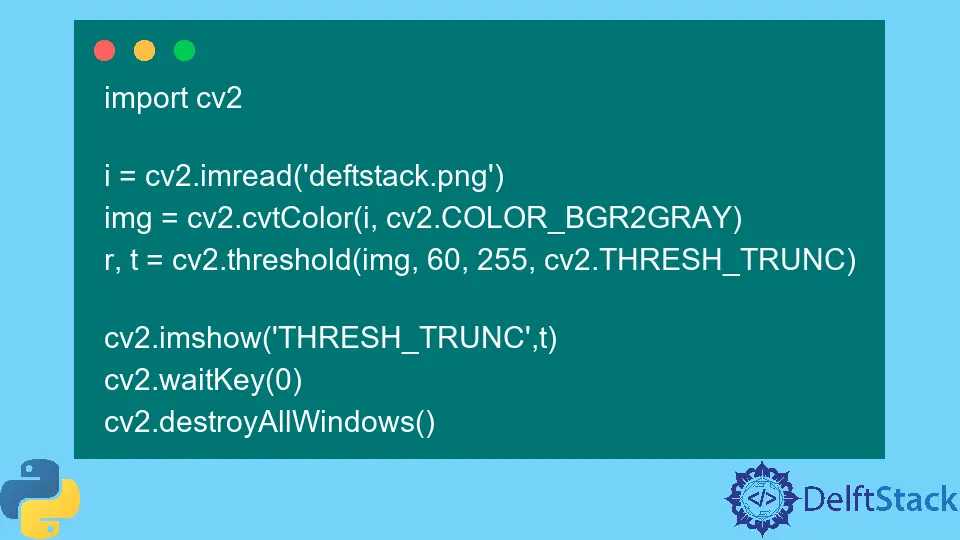
This tutorial will tackle using the cv2.threshold()
function in Python.
Thresholding Technique
Thresholding is an image processing technique done with black and white images and is useful in removing the noise and filtering pixels with extreme values. Here, we use an existing image to create a binary image by adjusting the value of pixels based on some threshold value.
Every pixel value is compared to the given threshold. If the value is less than a threshold, it is set to 0
; otherwise, it is set to maximum (255
).
We can use the OpenCV library in Python to read and process images for computer vision techniques. We can use the cv2.threshold()
function to perform thresholding with this library.
Use the cv.threshold()
Function for Thresholding in OpenCV
The cv2.threshold()
function implements the basic binary thresholding technique for images in Python. It replaces the pixel value to 0 or maximum based on its comparison with the given threshold value and returns the threshold value with the processed image as a tuple.
We provide the image for processing using the src
parameter. The threshold value for comparison is provided using the thresh
parameter.
The maximum value can be provided using the maxval
parameter. This is used depending on the type of thresholding technique which is discussed below.
The main parameter associated with this function is the type
parameter. This determines the type of binary thresholding to be used.
The type
parameter can accept five values. The first type is cv2.THRESH_BINARY
, which follows the basics of binary thresholding by assigning the pixel value 0
when it is less than the threshold and 255
when it is greater.
Example:
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2GRAY)
r, t = cv2.threshold(img, 60, 255, cv2.THRESH_BINARY)
cv2.imshow("THRESH_BINARY", t)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
We use the cv2.imread()
function to read the image in the example above. Then, convert it to a grayscale image using the cv2.cvtColor()
function, and this process will remain the same for the other types as well.
Then, we apply the cv2.threshold()
function and provide the required parameters. The threshold value is 60
, and we apply the cv2.THRESH_BINARY
technique. The final result is displayed in a window using the cv2.imshow()
function.
The cv2.waitKey()
and cv2.destroyAllWindows()
functions prevent the window from closing immediately and close them when the user presses any key. Let’s discuss the other types.
We have the cv2.THRESH_BINARY_INV
type, the opposite of the previous type. It assigns maximum value to a pixel when it is less than the threshold and assigns 0
.
Example:
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2GRAY)
r, t = cv2.threshold(img, 60, 255, cv2.THRESH_BINARY_INV)
cv2.imshow("THRESH_BINARY_INV", t)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
We process the thresholding technique by using the cv2.THRESH_BINARY_INV
type in the cv2.threshold()
function. The procedure is similar to the last one.
The third type is cv2.THRESH_TRUNC
if the pixel value is assigned as the threshold when it exceeds the threshold value.
All pixels smaller than the threshold remain the same.
Example:
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2GRAY)
r, t = cv2.threshold(img, 60, 255, cv2.THRESH_TRUNC)
cv2.imshow("THRESH_TRUNC", t)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
We implement the cv2.THRESH_TRUNC
thresholding technique using the cv2.threshold()
function in Python.
There is also the cv2.THRESH_TOZERO
type. All the pixel values less than the threshold are changed to zero, whereas the remaining are unchanged.
Example:
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2GRAY)
r, t = cv2.threshold(img, 60, 255, cv2.THRESH_TOZERO)
cv2.imshow("THRESH_TOZERO", t)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
The inverse to the previous type is the cv2.THRESH_TOZERO_INV
type, where pixel values greater than the threshold is changed to zero. We can use it similarly.
Example:
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2GRAY)
r, t = cv2.threshold(img, 120, 255, cv2.THRESH_TOZERO_INV)
cv2.imshow("THRESH_TOZERO_INV", t)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
Conclusion
We discussed implementing simple thresholding in this article using the cv2.threshold()
function. Thresholding is the technique of altering the pixel value compared to some threshold value.
We discussed how to use the cv2.threshold()
function and its parameters. There are five types of thresholding possible with this using different values for the type
parameter.
There also exists adaptive thresholding, which can be achieved using the OpenCV library.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn