Adaptive Threshold Using OpenCV
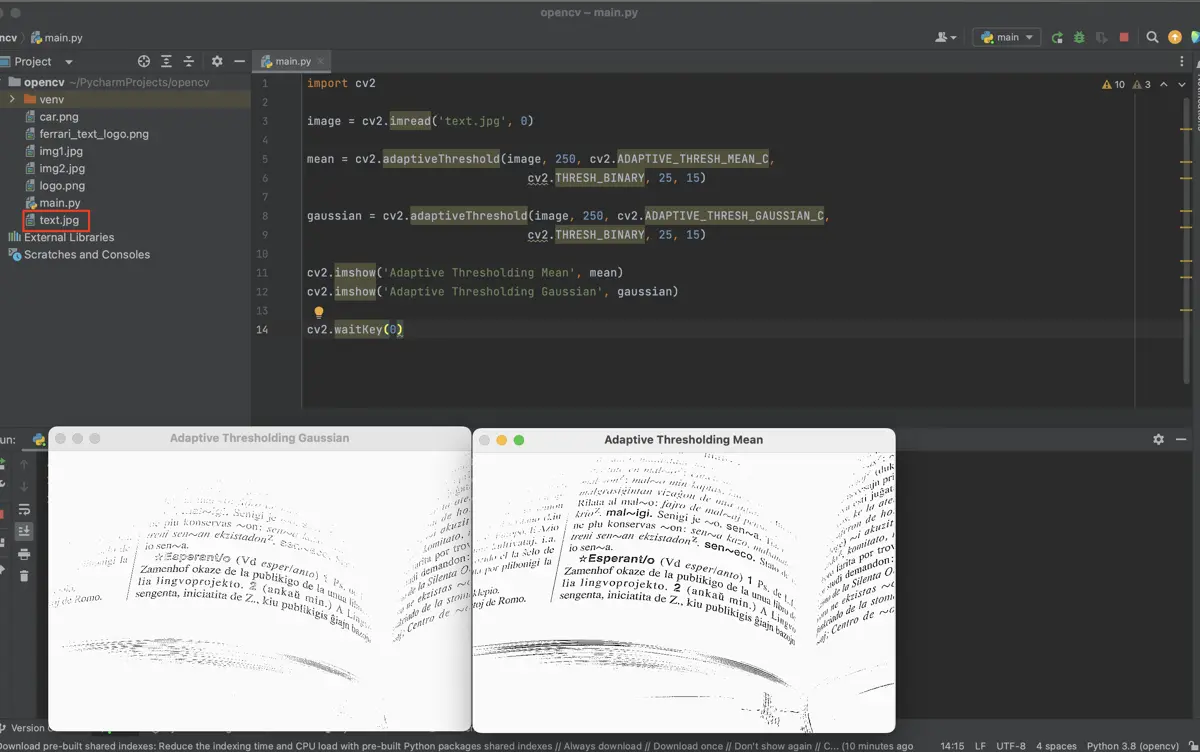
OpenCV has two types of thresholding, simple thresholding and adaptive thresholding.
In this article, we will see what adaptive thresholding is in detail and how to implement it using the OpenCV library.
Adaptive Thresholding Using OpenCV
In OpenCV, adaptive thresholding is the process in which a different threshold value is calculated for every image region. Unlike simple thresholding, the same threshold value is used for all the other pixels in the image.
If a pixel has a value smaller than the threshold, then 0
is set; if the pixel has a value greater than the threshold, then 255
is set.
The adaptive threshold uses two methods: adaptive threshold mean and gaussian. Below is the syntax for the adaptive thresholding.
Syntax:
cv2.adaptiveThreshold(
inputImage,
maximumValue,
thresholdMethod,
typeOfthreshold,
sizeOfBlock,
constantValue,
)
Below are the parameters passed to the adaptiveThreshold()
method.
inputImage
: The source is the input image.maximumValue
: Maximum value that can be assigned to a pixel. This is nothing but the maximum threshold value. At most, it can take 255.adaptiveMethod
: Adaptive method determines how the threshold value is to be calculated. There are two adaptive methods for adaptive thresholding.cv2.ADAPTIVE_THRESH_MEAN_C
: It takes the mean of the neighborhood area values subtracted by the constant value, i.e., the mean of the block size multiplied by to block size neighborhood of a point minus constant value.cv2.ADAPTIVE_THRESH_GAUSSIAN_C
: It takes the Gaussian-weighted sum of the neighborhood values subtracted by the constant value, i.e., it takes the weighted sum of the block size multiplied to block size neighborhood of a point minus constant value.typeOfthreshold
: The type of thresholding to be applied.sizeOfBlock
is the neighboring area’s size.constantValue
: A constant value deducted from the average or weighted sum of the nearby pixels.
Now that we have gone through the syntax and parameters of the adaptiveThreshold()
method let’s now take an example and see how adaptive thresholding works in practice.
First, ensure that you have the OpenCV library installed on your system. After that, you must import the OpenCV library, as shown in the example below.
The adaptive threshold method expects the source image as a greyscale image. So, while reading the image with the imread()
method, we have to pass 0
as the second argument to get a greyscale image and then store that image inside the image
variable.
Code Snippet:
import cv2
image = cv2.imread("text.jpg", 0)
mean = cv2.adaptiveThreshold(
image, 250, cv2.ADAPTIVE_THRESH_MEAN_C, cv2.THRESH_BINARY, 25, 15
)
gaussian = cv2.adaptiveThreshold(
image, 250, cv2.ADAPTIVE_THRESH_GAUSSIAN_C, cv2.THRESH_BINARY, 25, 15
)
cv2.imshow("Adaptive Thresholding Mean", mean)
cv2.imshow("Adaptive Thresholding Gaussian", gaussian)
cv2.waitKey(0)
The image contains text from a book. Now we will apply both adaptive threshold techniques to the above image and pass the same parameters to both these techniques and see the difference in the output images.
First, we will pass the image and then as the second parameter, we will pass 255
(white) and the adaptive method ADAPTIVE_THRESH_MEAN_C
and ADAPTIVE_THRESH_GAUSSIAN_C
respectively, as the third parameter.
For the threshold type, we will pass the THRESH_BINARY
, which will perform basic thresholding on the image. Then you have to specify the size of the neighboring area; in this case, we have passed 25
as the block size.
Now, we will pass some constant value as the last parameter to the adaptiveThreshold()
method and then store the results of both of these operations inside the variables mean
and gaussian
, respectively.
In the end, you can print these resultant images on the output window using the imshow()
method and specify names for the tabs. This is what the output of both the mean and gaussian looks like.
Output:
Conclusion
There are two types of thresholding in OpenCV: simple and adaptive. In simple thresholding, the same threshold value is applied to all the other pixels in the image.
This type of thresholding may work well for some images where the lighting conditions do not vary. But in most of the images, the lighting varies quite a lot.
In such cases using this thresholding technique might not be a good approach.
Therefore, the OpenCV library also has another thresholding technique called adaptive thresholding. In adaptive thresholding, for every region, a different threshold value is calculated, providing better results.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn