How to Create a QR Code Scanner Using OpenCV in Python
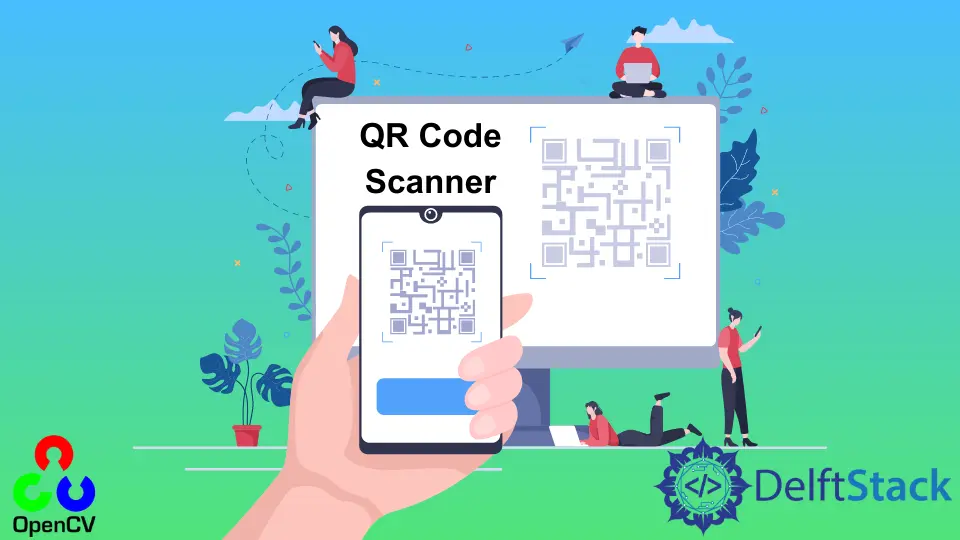
We are all familiar with barcodes that are present in different products. Based on the Morse code, Barcodes store information by representing it using lines and spaces and store the data horizontally.
In recent times, QR Codes have also gained popularity. QR codes are stored in a box and are represented using a combination of black and white squares with some dots.
It is also based on Morse code, so it can be considered a type of matrix of barcodes.
QR codes have the edge over traditional barcodes in terms of storage. It stores data in two directions; hence it can contain more information than barcodes.
This information can be URLs, contacts, and other information up to four thousand characters. There is an application for the QR code scanner in almost every phone nowadays.
This tutorial will demonstrate how to create a QR code scanner using the OpenCV library in Python. If you are interested in further exploring image processing, you can also check out our guide on how to use OCR to extract text from images using OpenCV: How to Use OCR to Extract Text From an Image in OpenCV.
The OpenCV library contains different functionalities and classes that can store and process images efficiently. We can apply a wide range of pre-defined techniques in our frames.
Use the QRCodeDetector
Class to Create a QR Code Scanner Using OpenCV in Python
Version 4.0.0 of the OpenCV library introduced the QRCodeDetector
class. This class contains methods that can be used to detect and scan images for QR codes.
We can scan single and multiple QR codes using different methods.
The detect()
method from this class is used to scan the image for QR codes; it finds the box that contains the QR code. The decode()
method decodes the detected QR code.
To detect multiple QR codes in a given image, we can use the detectMulti()
and decodeMulti()
functions. For detecting and decoding codes on a curved surface, we use the detectCurved()
and decodeCurved()
methods, respectively.
We can use the detectandDecode()
function to simultaneously detect and decode codes from an image. For this method, the detectandDecodeCurved()
and detectandDecodeMulti()
methods also exist.
Let us now create a very simple QR code using this class and will scan the QR code in the following image:
See the code below.
import cv2
img = cv2.imread("2.jpg")
det = cv2.QRCodeDetector()
info, box_coordinates, _ = det.detectAndDecode(img)
if box_coordinates is None:
print("No Code")
else:
print(info)
if box_coordinates is not None:
box_coordinates = [box_coordinates[0].astype(int)]
n = len(box_coordinates[0])
for i in range(n):
cv2.line(
img,
tuple(box_coordinates[0][i]),
tuple(box_coordinates[0][(i + 1) % n]),
(0, 255, 0),
3,
)
cv2.imshow("Output", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
http://sampleurl.com
In the above example, we created an object of the QRCodeDetector
class and used the detectandDecode()
function to scan and decode the QR code in the given image. This function returned the information of the QR code which is displayed.
It also returned the coordinates of the box surrounding the QR code. We used these coordinates to draw its boundaries on the image using the line()
function.
The final image is displayed, and we used the waitKey()
and destroyAllWindows()
functions to prevent this window from closing automatically and wait for the user to press some key before closing it.
In our example, we read an image from the directory and scanned the QR code. We can make this more interactive by using the webcam and reading images from there. For handling image input effectively, you may find our article on how to read text from images using Tesseract in Python helpful: How to Read Text From Images Using Tesseract in Python.
OpenCV provides the VideoCapture
class to capture and read visuals from the webcam.
Conclusion
This tutorial demonstrated using Python’s OpenCV library to create a simple QR code scanner. We started by discussing the basics of a QR code, its comparisons with a barcode, and the information it can store.
We discussed, in brief, the QRCodeDetector
class from the OpenCV library, which provides plenty of methods to detect and decode QR codes from images. We provided an example of a simple QR code scanner using this class to scan and decode the QR code from a given image.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn