How to Fix Python NameError: Name Execfile Is Not Defined
-
Alternative to
execfile()
in Python 3 -
Use
exec()
to Execute a Python File -
Use
with
Block to Execute a Python File Usingexec()
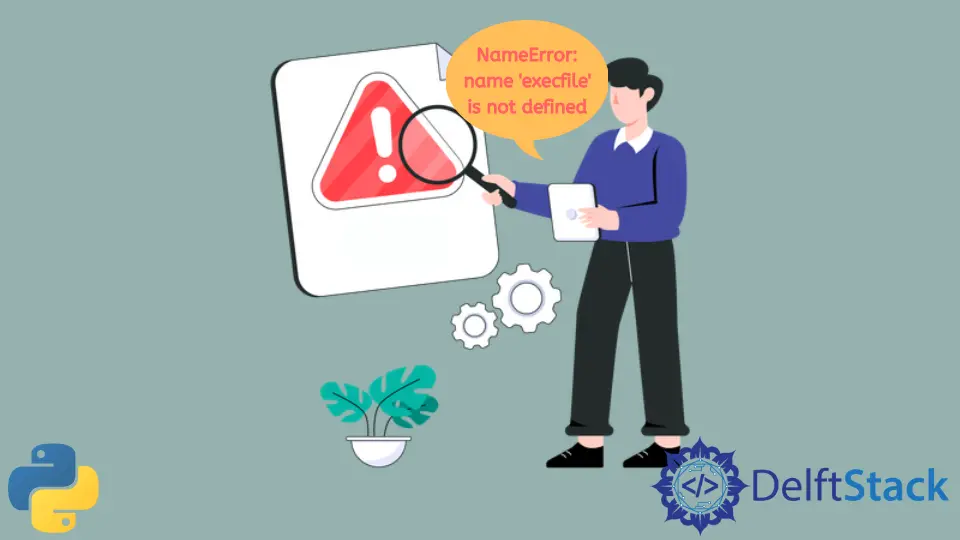
In Python 2, there is an inbuilt function execfile()
in which a file is parsed and evaluated as Python statements. This function is no longer in Python 3.
This article demonstrates the possible alternative to executing a file in Python 3.
Alternative to execfile()
in Python 3
In Python 2, we use the function execfile()
to execute a file. In Python 3, we have a similar function called exec()
.
First, we will open and read the file and pass it as an argument to the exec()
function.
the exec()
Function in Python 3
Python exec()
function executes dynamically created code block, passed as a string.
General Syntax:
# Python 3.x
exec(object, global, local)
Three parameters are required for the exec()
function.
- An
object
can be a string or multiple-line code. - A
global
parameter can be a dictionary. - A
local
can be a mapping dictionary.
Both local
and global
parameters are optional. Also, the exec()
is a void function and does not return any value.
The below code demonstrates the basic working of this function. The string "programming is fun"
is printed in the following code.
Three mathematical operations are performed and are passed individually to the exec()
function.
Similarly, we can add multiple lines to a string, and each line is parsed, considered as Python statements, and executed.
Example Code:
# Python 3.x
exec('print("programming is fun")')
exec('x=4; y=9; print("Multiplication:", x*y)')
w = 200
exec("print(w == 200)")
exec("print(w / 100)")
Output:
programming is fun
Multiplication: 36
True
2.0
Use exec()
to Execute a Python File
Now, if we want to execute an external Python file in our code, we first need to open the file, read it and pass it to the exec()
function as an argument.
Here we have created a file, myfile.py
in our relative directory, which contains the following code.
# Python 3.x
print("Delftstack")
To execute this file in our Python code, we will open it first using open()
, then read it using the read()
function, and finally, run it using the exec()
function.
Example Code:
# Python 3.x
exec(open("myfile.py").read())
Output:
Delftstack
Use with
Block to Execute a Python File Using exec()
The with
block safely closes the file (automatically) when it reaches the end of the block, ensuring that none of the files remains open.
Example Code:
# Python 3.x
with open("myfile.py", "r") as f:
exec(f.read())
Output:
Delftstack
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python