How to Execute a Script in Python
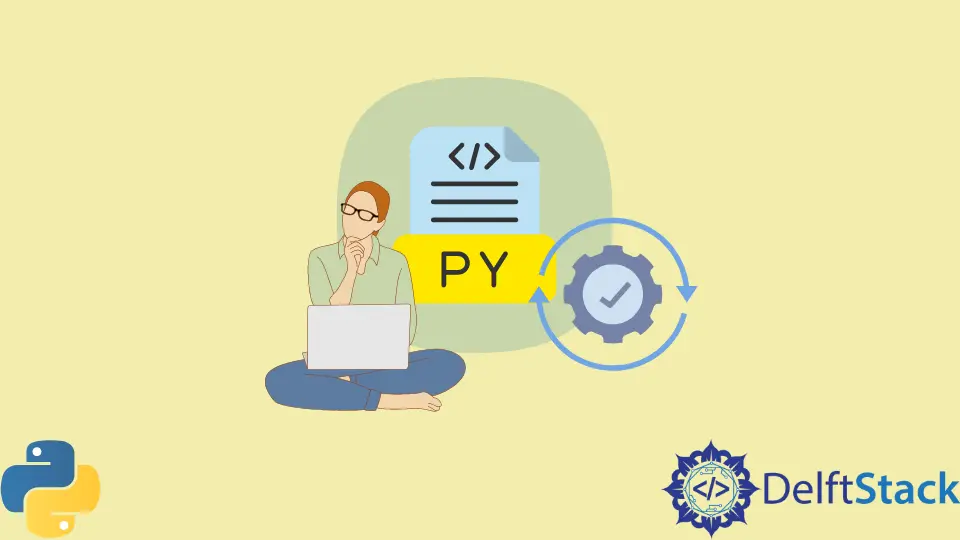
This article will talk about how we can use Python to execute yet another python script.
Execute a Python Script in Python
We can execute a Python script using Python with the help of the exec()
function. The exec()
function is a Python utility that allows the dynamic execution of Python code both in string and byte format. This function accepts three arguments, namely,
object
: The Python code in string or byte formatglobals
: It is an optional argument. It is a dictionary and contains global functions and variables.locals
: It is an optional argument. It is a dictionary and contains local functions and variables.
Refer to the following code for an example.
file_name = "code.py"
content = open(file_name).read()
exec(content)
The above code first opens a file in the working directory by the name of code.py
, reads its content, stores it inside a variable by the name content
, and then passes the read content to the exec()
function. The read content is some Python code, and the exec()
method will execute that Python code.
If we have some local or global variables, we can store them inside dictionaries and pass them to the executable script. The script will then be able to utilize those variables and run the code. Note that if we use some functions inside the executable script that we have not passed as a global variable, the main program will throw an Exception.
The following code depicts the same.
from random import randint
code = "print(randint(1, 6))"
exec(code, {}, {}) # Empty globals and locals
Output:
Traceback (most recent call last):
File "<string>", line 4, in <module>
File "<string>", line 1, in <module>
NameError: name 'randint' is not defined
When the globals
and locals
are not passed to the exec()
, the requirements (to run the script) are automatically handled. Python is smart enough to figure out what all variables or functions are needed to run the script. For example, the following code will run perfectly without any error.
from random import randint
code = "print(randint(1, 6))"
exec(code) # No globals or locals passed
Use of globals
and locals
We can create custom functions and imported functions inside the executable script by passing them to the exec()
function via globals
. As mentioned above, if these dependencies are not found, exceptions will be raised.
Refer to the following code. Here we will define a custom function and import a function from the random
package. Then both functions will be passed to the exec()
function wrapped inside a dictionary via the globals
parameter.
from random import randint
def get_my_age():
return 19
globals = {"randint": randint, "get_my_age": get_my_age}
code = """
print(randint(1, 6))
print(get_my_age())
"""
exec(
code,
globals,
)
Output:
5 # It can be any number between 1 and 6, both inclusive
19
Right now, these functions have been passed via the globals
parameter. We can also pass them through locals
. Since the exec
function doesn’t accept keyword arguments, we have to pass an empty dictionary for globals
and the pass out locals
.
from random import randint
def get_my_age():
return 19
locals = {"randint": randint, "get_my_age": get_my_age}
code = """
print(randint(1, 6))
print(get_my_age())
"""
exec(code, {}, locals)
Output:
2 # It can be any number between 1 and 6, both inclusive
19