How to Execute Input Validation in Python
-
Use the
try...except
Statement to Check if the User Input Is Valid in Python -
Uses the
isdigit()
Function to Check if the User Input Is Valid in Python
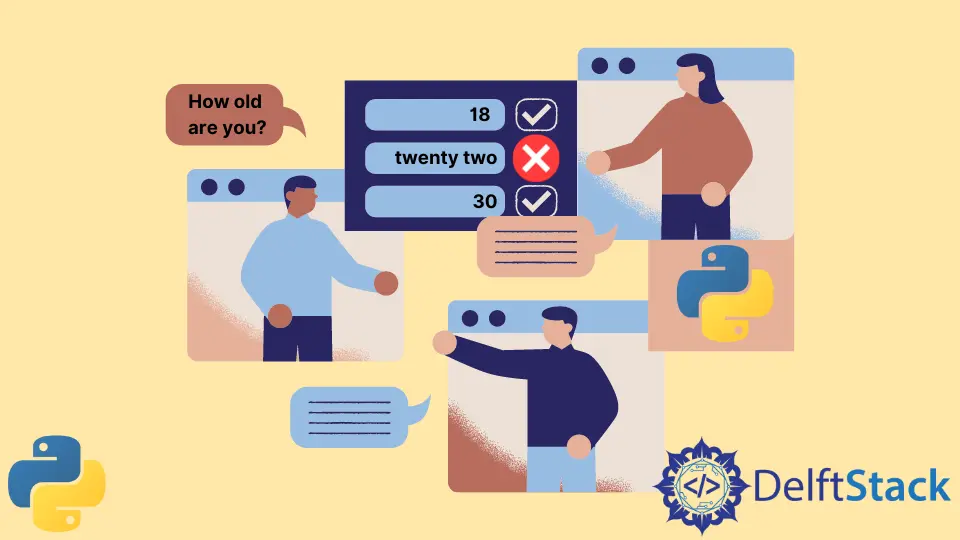
Input validation can be defined as the process of checking if the input provided by the user from the input()
function is both valid and acceptable in a given case or scenario.
In this article, you’ll learn how to check if the user input is valid in Python.
Use the try...except
Statement to Check if the User Input Is Valid in Python
The try...except
statement is utilized for the purpose of exception handling in Python. The try...except
statement is divided into three blocks, all of which have different uses.
- The
try
block contains the cluster of code to be tested for any errors. - The
except
block is used to add exceptions and handle the errors of the code. - The
finally
block contains the statements that need to be executed; it’s ignored by thetry
andexcept
blocks.
To explain input validation in Python, we will take a program where the user is asked for input using the built-in input()
function in Python.
The following code implements the try...except
statement to check if the user input is valid in Python.
while True:
try:
dage = int(input("Enter your age: "))
except ValueError:
print("This is an unaccepted response, enter a valid value")
continue
else:
break
if dage >= 21:
print("You're the legal age to drink in India")
else:
print("You're not the legal age to drink in India")
Output:
Enter your age: eight
This is an unaccepted response, enter a valid value
Enter your age: 8
You're not the legal age to drink in India
In the code above, the try
block contains the input function and sets the parameter that the value the user will input should be an int
. Then, the except
block handles the error. Python allows us to define as many except
blocks as we want in a chunk of code.
The output shows the error message of the except
block when any other value is inputted, instead of an int
value. The latter part of the program is easy and uses the if-else
statement to check whether a person is at a legal drinking age or not.
Uses the isdigit()
Function to Check if the User Input Is Valid in Python
The isdigit()
function can be utilized to check whether the value provided of an input is a digit (0-9) or not. It returns a True
value if the string contains numeric integer values only; it does not consider floating-point numbers.
The following code executes the user-defined function that uses the isdigit()
function to check if the user input is valid in Python.
def check1(num):
if num.strip().isdigit():
print("The Input is Number")
else:
print("The Input is string")
x = input("Enter your age:")
check1(x)
Output:
Enter your age:18
The Input is Number
The user-defined function is created just to check whether the user input received is a number or a string.
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn