在 Python 中執行輸入驗證
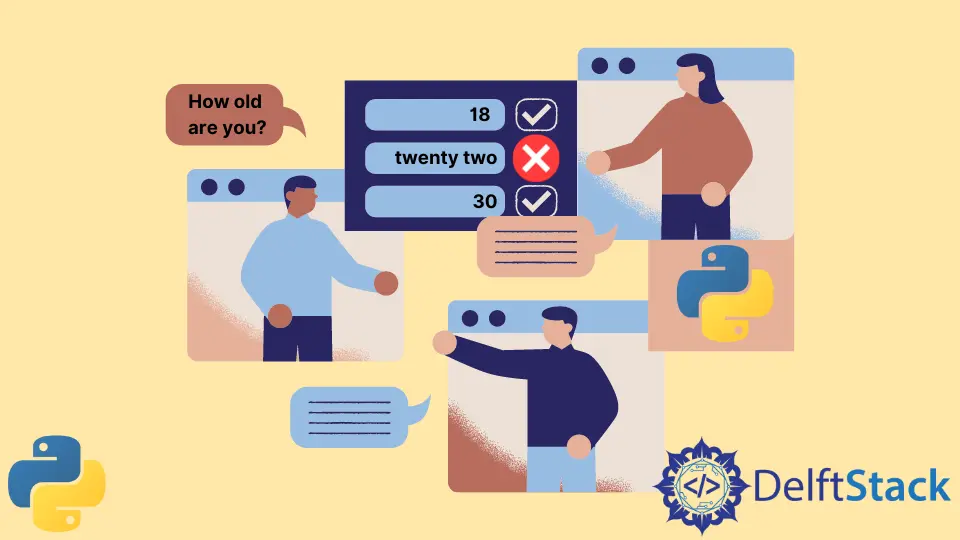
輸入驗證可以定義為檢查使用者從 input()
函式提供的輸入在給定案例或場景中是否既有效又可接受的過程。
在本文中,你將學習如何檢查使用者輸入在 Python 中是否有效。
在 Python 中使用 try...except
語句檢查使用者輸入是否有效
try...except
語句用於 Python 中的異常處理。try...except
語句分為三個塊,所有塊都有不同的用途。
try
塊包含要測試任何錯誤的程式碼群。except
塊用於新增異常和處理程式碼錯誤。finally
塊包含需要執行的語句;它被try
和except
塊忽略。
為了解釋 Python 中的輸入驗證,我們將採用一個程式,其中使用 Python 中的內建 input()
函式要求使用者輸入。
以下程式碼實現了 try...except
語句以檢查使用者輸入在 Python 中是否有效。
while True:
try:
dage = int(input("Enter your age: "))
except ValueError:
print("This is an unaccepted response, enter a valid value")
continue
else:
break
if dage >= 21:
print("You're the legal age to drink in India")
else:
print("You're not the legal age to drink in India")
輸出:
Enter your age: eight
This is an unaccepted response, enter a valid value
Enter your age: 8
You're not the legal age to drink in India
在上面的程式碼中,try
塊包含輸入函式並設定使用者將輸入的值應該是 int
的引數。然後,except
塊處理錯誤。Python 允許我們在一段程式碼中定義任意數量的 except
塊。
當輸入任何其他值時,輸出顯示 except
塊的錯誤訊息,而不是 int
值。程式的後半部分很簡單,它使用 if-else
語句來檢查一個人是否達到法定飲酒年齡。
在 Python 中使用 isdigit()
函式使用者輸入是否有效
isdigit()
函式可用於檢查輸入的值是否為數字 (0-9)。如果字串僅包含數字整數值,則返回 True
值;它不考慮浮點數。
以下程式碼執行使用者定義的函式,該函式使用 isdigit()
函式來檢查使用者輸入在 Python 中是否有效。
def check1(num):
if num.strip().isdigit():
print("The Input is Number")
else:
print("The Input is string")
x = input("Enter your age:")
check1(x)
輸出:
Enter your age:18
The Input is Number
建立使用者定義函式只是為了檢查接收到的使用者輸入是數字還是字串。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn