在 Python 中执行输入验证
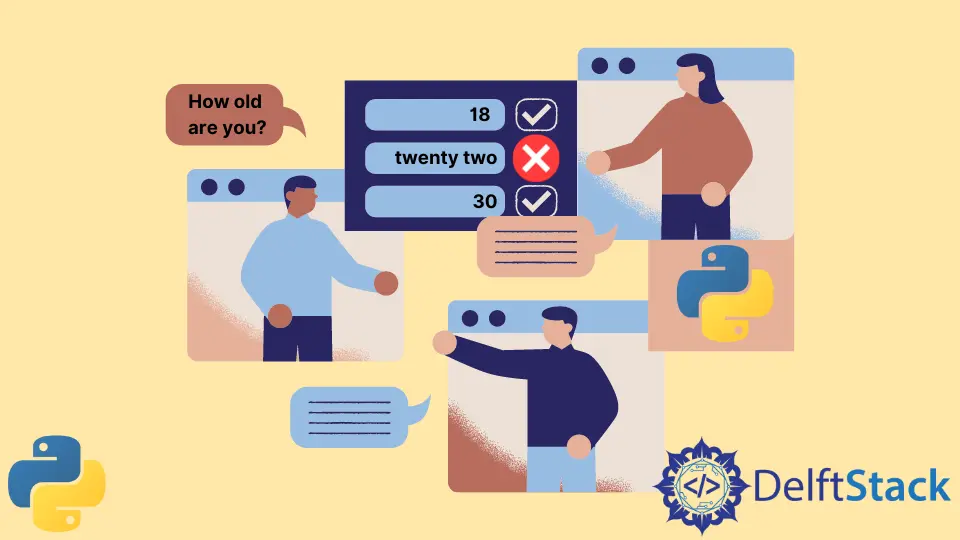
输入验证可以定义为检查用户从 input()
函数提供的输入在给定案例或场景中是否既有效又可接受的过程。
在本文中,你将学习如何检查用户输入在 Python 中是否有效。
在 Python 中使用 try...except
语句检查用户输入是否有效
try...except
语句用于 Python 中的异常处理。try...except
语句分为三个块,所有块都有不同的用途。
try
块包含要测试任何错误的代码群。except
块用于添加异常和处理代码错误。finally
块包含需要执行的语句;它被try
和except
块忽略。
为了解释 Python 中的输入验证,我们将采用一个程序,其中使用 Python 中的内置 input()
函数要求用户输入。
以下代码实现了 try...except
语句以检查用户输入在 Python 中是否有效。
while True:
try:
dage = int(input("Enter your age: "))
except ValueError:
print("This is an unaccepted response, enter a valid value")
continue
else:
break
if dage >= 21:
print("You're the legal age to drink in India")
else:
print("You're not the legal age to drink in India")
输出:
Enter your age: eight
This is an unaccepted response, enter a valid value
Enter your age: 8
You're not the legal age to drink in India
在上面的代码中,try
块包含输入函数并设置用户将输入的值应该是 int
的参数。然后,except
块处理错误。Python 允许我们在一段代码中定义任意数量的 except
块。
当输入任何其他值时,输出显示 except
块的错误消息,而不是 int
值。程序的后半部分很简单,它使用 if-else
语句来检查一个人是否达到法定饮酒年龄。
在 Python 中使用 isdigit()
函数用户输入是否有效
isdigit()
函数可用于检查输入的值是否为数字 (0-9)。如果字符串仅包含数字整数值,则返回 True
值;它不考虑浮点数。
以下代码执行用户定义的函数,该函数使用 isdigit()
函数来检查用户输入在 Python 中是否有效。
def check1(num):
if num.strip().isdigit():
print("The Input is Number")
else:
print("The Input is string")
x = input("Enter your age:")
check1(x)
输出:
Enter your age:18
The Input is Number
创建用户定义函数只是为了检查接收到的用户输入是数字还是字符串。
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn