How to Convert Integer to String in Python
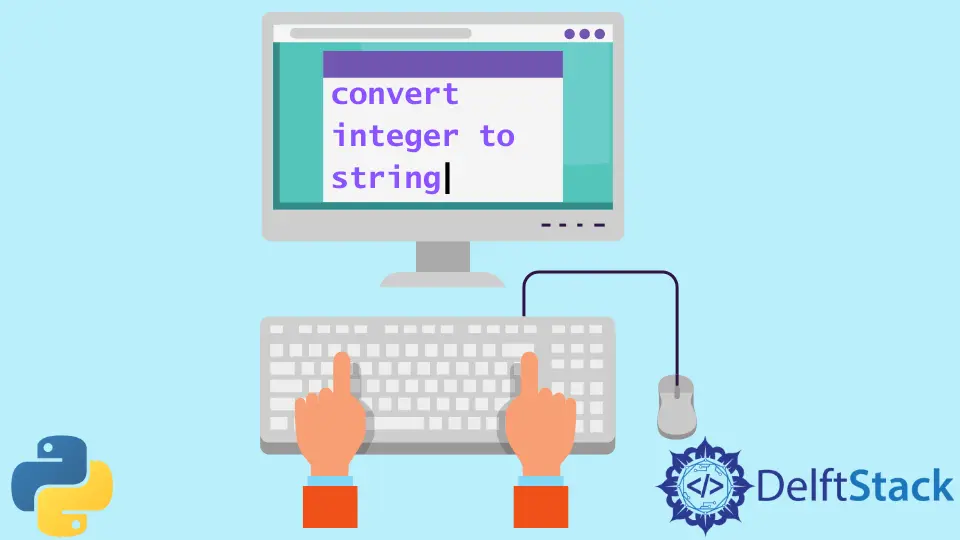
Converting an integer to a string in Python is a fundamental skill that every programmer should master. Whether you’re working with user input, displaying messages, or formatting data for output, knowing how to make this conversion is essential.
In this article, we will explore two primary methods to convert integers to strings: using the built-in str()
function and employing f-string formatting. Both techniques have their unique advantages, and understanding them will enhance your coding efficiency. Let’s dive into these methods and see how you can seamlessly convert integers to strings in Python.
Using the str()
Function
The most straightforward way to convert an integer to a string in Python is by using the built-in str()
function. This function takes an integer as an argument and returns its string representation. It’s simple, effective, and widely used in various programming scenarios.
Here’s how you can use the str()
function for conversion:
number = 42
string_number = str(number)
print(string_number)
Output:
42
In this example, we define a variable number
with an integer value of 42. When we pass this variable to the str()
function, it converts the integer into a string and assigns it to the variable string_number
. Finally, we print string_number
, which outputs “42” as a string.
The str()
function is versatile, making it suitable for various data types. You can use it not only with integers but also with floats, lists, and other objects. This flexibility is particularly useful when you need to concatenate strings with numbers or format output for display. It’s important to remember that the conversion is not in-place; instead, it creates a new string object. This method is widely accepted and is often the go-to solution for many programmers.
Using f-String Formatting
Another modern and efficient way to convert an integer to a string in Python is through f-string formatting, introduced in Python 3.6. F-strings allow you to embed expressions inside string literals, making the code cleaner and more readable.
Here’s a quick example of how to use f-string formatting for conversion:
number = 42
string_number = f"{number}"
print(string_number)
Output:
42
In this snippet, we again define a variable number
with the value 42. The f-string f"{number}"
converts the integer to a string by embedding the variable directly within the curly braces. The result is assigned to string_number
, which we print afterward. The output is “42”, just like before.
F-string formatting is particularly powerful because it can include more complex expressions and even format numbers in various ways. For example, you can control the number of decimal places for floats or format integers with leading zeros. This method not only converts integers to strings but also enhances the overall readability of your code. It’s a favorite among many Python developers for its simplicity and effectiveness.
Conclusion
Converting integers to strings in Python is a straightforward process, thanks to the built-in str()
function and the modern f-string formatting. Both methods are easy to implement and serve different purposes depending on your specific needs. The str()
function is great for direct conversions, while f-strings offer enhanced readability and flexibility for embedding expressions. By mastering these techniques, you can improve your code’s clarity and functionality, making it easier to manage data and output.
As you continue to develop your Python skills, remember that understanding these basic conversions will serve as a foundation for more complex programming tasks. Happy coding!
FAQ
-
How do I convert a float to a string in Python?
You can use thestr()
function in the same way as with integers. For example,string_float = str(3.14)
will convert the float 3.14 to a string. -
Can I use f-strings with other data types?
Yes, f-strings can be used with various data types, including lists, dictionaries, and even custom objects. -
What version of Python introduced f-strings?
F-strings were introduced in Python 3.6, so you need to be using this version or later to utilize them. -
Is there a performance difference between str() and f-strings?
Generally, f-strings are faster than thestr()
function, especially when formatting complex expressions or concatenating multiple variables. -
Can I convert multiple integers to strings at once?
Yes, you can use a list comprehension or a loop to convert multiple integers to strings. For example,string_list = [str(i) for i in int_list]
will convert all integers inint_list
to strings.
Related Article - Python Integer
- How to Convert Int to Binary in Python
- How to Convert Roman Numerals to Integers in Python
- How to Convert Integer to Roman Numerals in Python
- Integer Programming in Python
- How to Convert Boolean Values to Integer in Python
- How to Convert String to Integer in Python