How to Convert Image to Grayscale in Python
-
Convert an Image to Grayscale in Python Using the
image.convert()
Method of thepillow
Library -
Convert an Image to Grayscale in Python Using the
color.rgb2gray()
Method of thescikit-image
Module -
Convert an Image to Grayscale in Python Using the
cv2.imread()
Method of theOpenCV
Library -
Convert an Image to Grayscale in Python Using the Conversion Formula and the
Matplotlib
Library
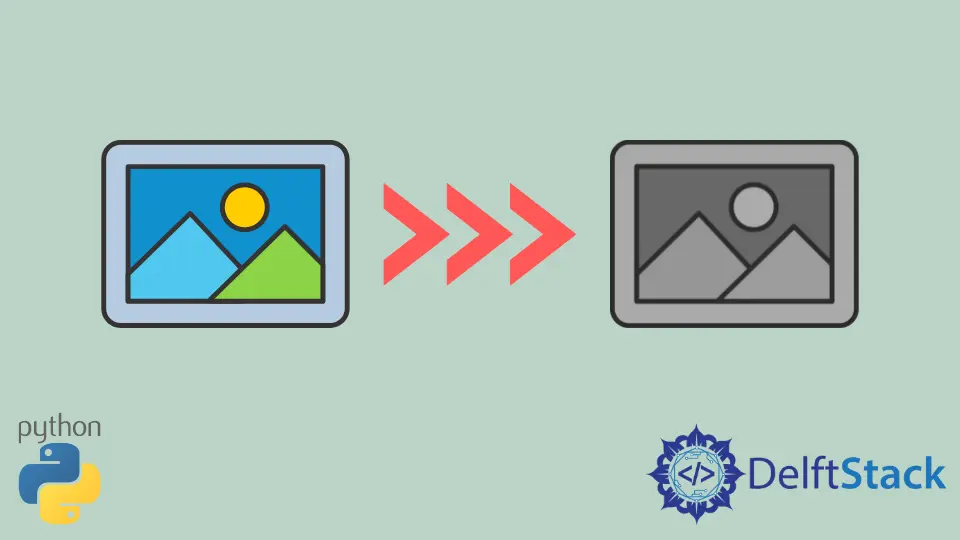
This tutorial will explain various methods to convert an image to grayscale in Python. A grayscale image is an image in which a single pixel represents the amount of light or only contains light intensity information. It is a single-dimensional image and has different shades of gray color only.
As the grayscale images are single-dimensional, they are used to decrease models’ training complexity in various problems and in algorithms like the Canny edge detection.
This article will look into how we can convert an image to grayscale or read an image as grayscale in Python using various methods of Python’s modules.
Convert an Image to Grayscale in Python Using the image.convert()
Method of the pillow
Library
The image.convert(mode, ..)
method takes an image as input and converts it into the desired image type specified in the mode
argument. The mode includes 1-bit and 8-bits pixel black and white images, RGB
images, HSV
images, BGR
images and, LAB
images, etc.
As we want to convert our image to grayscale, we can pass 1
as mode argument for 1-bit black and white mode, L
for 8-bits black and white image, and LA
for alpha mode. The below example code demonstrates how to use the image.convert()
method of the pillow
library to convert an image to grayscale in Python:
from PIL import Image
img = Image.open("test.jpg")
imgGray = img.convert("L")
imgGray.save("test_gray.jpg")
Original image:
Converyted grayscale image:
Convert an Image to Grayscale in Python Using the color.rgb2gray()
Method of the scikit-image
Module
The color.rgb2gray()
takes an image in RGB format as input and returns a grayscale copy of the input image. The below code example demonstrates how to use the color.rgb2gray()
method of the scikit-image
module to get a grayscale image in Python.
from skimage import color
from skimage import io
img = io.imread("test.jpg")
imgGray = color.rgb2gray(img)
Convert an Image to Grayscale in Python Using the cv2.imread()
Method of the OpenCV
Library
Another method to get an image in grayscale is to read the image in grayscale mode directly, we can read an image in grayscale by using the cv2.imread(path, flag)
method of the OpenCV
library.
Suppose the flag
value of the cv2.imread()
method is equal to 1. In that case, it will read the image excluding the alpha channel, if 0
it will read the image as grayscale, and if equal to -1
method will read the image including the alpha channel information.
Therefore, we can read an image as grayscale from a given path using the imread()
method by passing the flag
argument value as 1
.
The below example code demonstrates how to use the cv2.imread()
method to read an image in grayscale in Python:
import cv2
imgGray = cv2.imread("test.jpg", 0)
Convert an Image to Grayscale in Python Using the Conversion Formula and the Matplotlib
Library
We can also convert an image to grayscale using the standard RGB to grayscale conversion formula that is imgGray = 0.2989 * R + 0.5870 * G + 0.1140 * B
.
We can implement this method using the Matplotlib
library in Python, first we need to read the image using the mpimg.imread()
method and then get the red, blue and green dimension matrices of the RGB image after getting the matrices we can apply the formula on them to get the grayscale image. We need to multiply the complete matrices with the values given in the formula to get the grayscale image.
The below code example demonstrates how we can implement the RGB to grayscale conversion formula in Python using the Matplotlib
library.
from matplotlib import pyplot as plt
import matplotlib.image as mpimg
img = mpimg.imread("test.jpg")
R, G, B = img[:, :, 0], img[:, :, 1], img[:, :, 2]
imgGray = 0.2989 * R + 0.5870 * G + 0.1140 * B
plt.imshow(imgGray, cmap="gray")
plt.show()