How to Download Image in Python
-
Download Image Using the
urllib
Package in Python -
Download Image Using the
requests
Library in Python
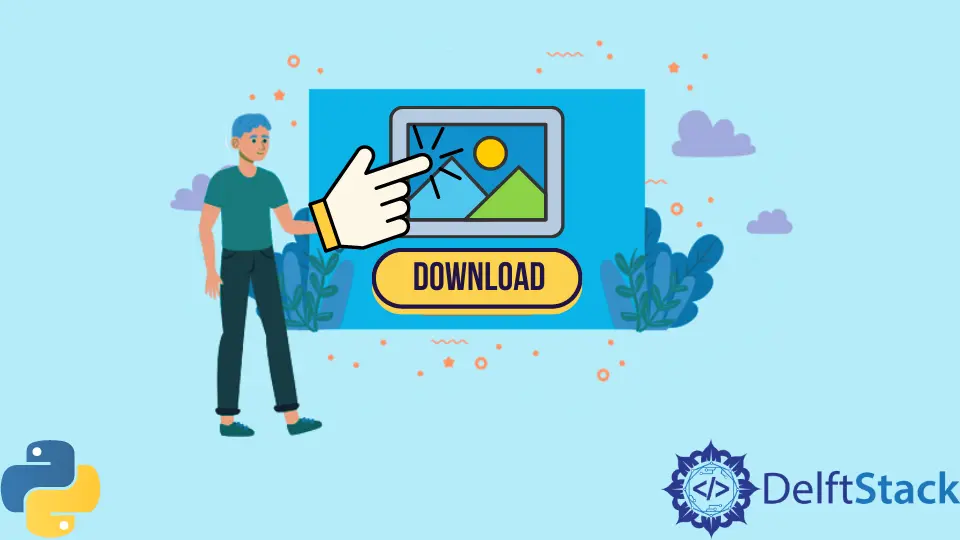
This tutorial will discuss some of the most prominent ways to download an image from the internet using Python.
Download Image Using the urllib
Package in Python
The urllib
package is a collection of several modules for working with URLs. urllib.request
is a module used for opening and reading content on URLs. For this tutorial, we will use Python to download an image file from https://www.python.org/images/success/nasa.jpg. In the urllib.request
module, two methods can be used to download an image, which will be explained below.
Download Image Using urllib.request.urlretrieve(url, filename)
Method
The following code example shows how we can download an image in Python using the urlretrieve(url, filename)
method.
import urllib.request
urllib.request.urlretrieve("https://www.python.org/images/success/nasa.jpg", "NASA.jpg")
print("download successful")
Output:
download successful
The above code downloads the image to the current working directory. The urlretrieve(url, filename)
method takes the image URL and the file name you want to store it as arguments. The urlretrieve(url, filename)
method is listed under the official documentation’s legacy interface, meaning that urlretrieve(url, filename)
method will deprecate in the future.
Download Image Using urllib.request.urlopen(url)
To overcome the inevitable deprecation of urlretrieve(url, filename)
method, urlopen(url)
method can be used with file handling. According to the official Python documentation, the urlopen(url)
method is used to open any URL.
The following code example shows how we can download an image using the urlopen(url)
method with file handling.
import urllib.request
f = open("NASA2.jpg", "wb")
f.write(urllib.request.urlopen("https://www.python.org/images/success/nasa.jpg").read())
f.close()
print("download successful")
Output:
download successful
In the above code, we first open a file named NASA2.jpg
in write binaries
mode using the open(filename, mode)
method. In the next line, we write the content read from the URL in the file using the write()
method. After that, we close the file using the close()
method.
Download Image Using the requests
Library in Python
The requests
is a Python library that we can use to send HTTP/1.1 requests to the server. We can send a GET
request to the URL using the get(url)
method in the requests
library to get the image file from the URL and then save it using the file handling.
The following code example shows how we can download an image using the requests
library with file handling.
import requests
f = open("NASA3.jpg", "wb")
response = requests.get("https://www.python.org/images/success/nasa.jpg")
f.write(response.content)
f.close()
print("download successful")
Output:
download successful
In the above code, we first open a file named NASA3.jpg
in write binaries
mode using the open(filename, mode)
method.
In the next line, we store the response from our GET request in the variable - response
.
After that, we write the binary contents from the response
in the file using the write()
method.
Finally, we close the file using the close()
method.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn