在 Python 中转换图像为灰度
-
在 Python 中使用
pillow
库的image.convert()
方法将图像转换为灰度图像 -
在 Python 中使用
scikit-image
模块的color.rgb2gray()
方法将图像转换为灰度 -
在 Python 中使用
OpenCV
库的cv2.imread()
方法将图像转换为灰度 -
在 Python 中使用转换公式和
Matplotlib
库将图像转换为灰度图像
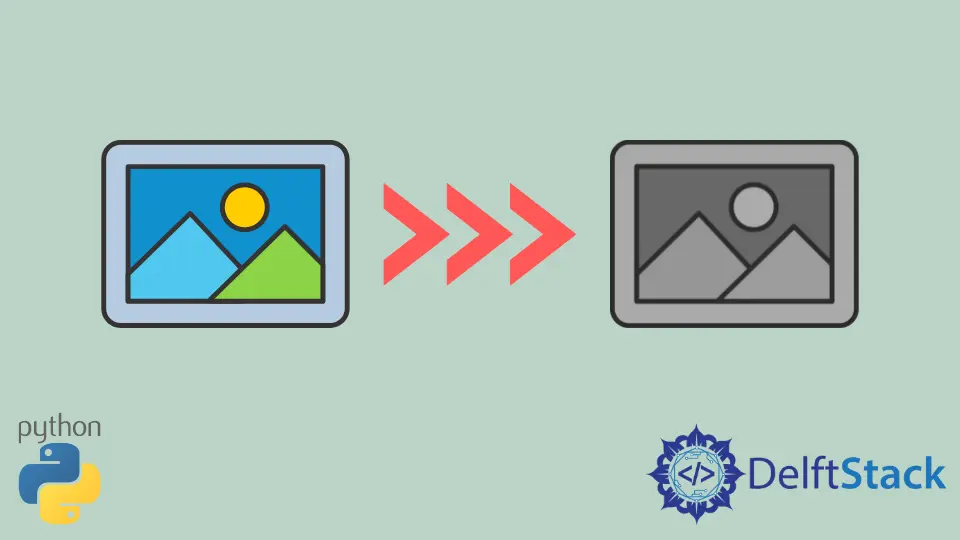
本教程将讲解用 Python 将图像转换为灰度图像的各种方法。灰度图像是指单个像素代表光量或只包含光强度信息的图像。它是一种单维图像,只具有不同的灰度颜色。
由于灰度图像是单维的,所以在各种问题中以及 Canny 边缘检测等算法中,灰度图像被用来降低模型的训练复杂性。
本文将探讨我们如何在 Python 中使用 Python 模块的各种方法将图像转换为灰度或将图像读为灰度。
在 Python 中使用 pillow
库的 image.convert()
方法将图像转换为灰度图像
image.convert(mode, ..)
方法将图像作为输入,并将其转换为 mode
参数中指定的所需图像类型。模式包括 1 位和 8 位像素的黑白图像、RGB
图像、HSV
图像、BGR
图像和 LAB
图像等。
由于我们要将图像转换为灰度图像,我们可以传递 1
作为模式参数,表示 1 位黑白模式,L
表示 8 位黑白图像,LA
表示 alpha 模式。下面的示例代码演示了如何在 Python 中使用 pillow
库的 image.convert()
方法将图像转换为灰度。
from PIL import Image
img = Image.open("test.jpg")
imgGray = img.convert("L")
imgGray.save("test_gray.jpg")
原始图像。
转换的灰度图像。
在 Python 中使用 scikit-image
模块的 color.rgb2gray()
方法将图像转换为灰度
color.rgb2gray()
将 RGB 格式的图像作为输入,并返回输入图像的灰度副本。下面的代码示例演示了如何使用 scikit-image
模块中的 color.rgb2gray()
方法在 Python 中获取一个灰度图像。
from skimage import color
from skimage import io
img = io.imread("test.jpg")
imgGray = color.rgb2gray(img)
在 Python 中使用 OpenCV
库的 cv2.imread()
方法将图像转换为灰度
另一种获取灰度图像的方法是直接读取灰度模式下的图像,我们可以使用 OpenCV
库中的 cv2.imread(path, flag)
方法来读取灰度图像。
假设 cv2.imread()
方法的 flag
值等于 1,在这种情况下,它将读取不包括 alpha 通道的图像,如果 0
则读取灰度图像,如果等于 -1
方法则读取包括 alpha 通道信息的图像。
因此,我们可以使用 imread()
方法,通过将 flag
参数值传递为 1
,从给定的路径中读取图像为灰度图像。
下面的示例代码演示了如何使用 cv2.imread()
方法在 Python 中读取灰度图像。
import cv2
imgGray = cv2.imread("test.jpg", 0)
在 Python 中使用转换公式和 Matplotlib
库将图像转换为灰度图像
我们还可以使用标准的 RGB 到灰度的转换公式将图像转换为灰度,即 imgGray = 0.2989 * R + 0.5870 * G + 0.1140 * B
。
我们可以使用 Python 中的 Matplotlib
库来实现这个方法,首先我们需要使用 mpimg.imread()
方法来读取图像,然后得到 RGB 图像的红、蓝、绿三个维度的矩阵,得到矩阵后我们就可以对它们应用公式来得到灰度图像。我们需要将完整的矩阵与公式中给出的值相乘,得到灰度图像。
下面的代码示例演示了我们如何在 Python 中使用 Matplotlib
库实现 RGB 到灰度的转换公式。
from matplotlib import pyplot as plt
import matplotlib.image as mpimg
img = mpimg.imread("test.jpg")
R, G, B = img[:, :, 0], img[:, :, 1], img[:, :, 2]
imgGray = 0.2989 * R + 0.5870 * G + 0.1140 * B
plt.imshow(imgGray, cmap="gray")
plt.show()