How to Concatenate String and Int Values in Python
-
Use the
str()
Function to Implement String and Integer Concatenation in Python -
Use String Formatting With the Modulo
%
Sign for String and Integer Concatenation in Python -
Use String Formatting With the
str.format()
Function for String and Integer Concatenation in Python -
Use the
f-string
for String Formatting in Python
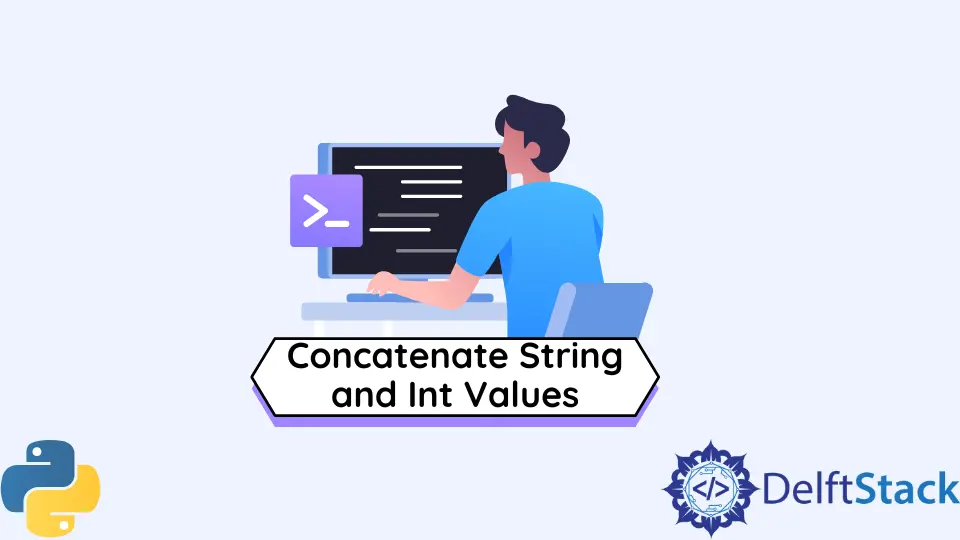
Concatenation can be defined as the integration of two strings into an object. In Python, you can execute concatenation using the +
operator. Here, we’ll discuss how to implement string and integer concatenation in Python successfully.
In most programming languages, you commonly encounter this operation: if a concatenation process is to be carried out between a string and an integer, the language automatically converts the integer value to a string value first and then continues the string concatenation process.
Python is an exception to this action and throws an error if a string is to be concatenated with an integer.
The following code takes a try at implementing string and integer concatenation in Python.
x = "My crypto portfolio amount in dollars is "
y = 5000
print(x + y)
Output:
Traceback (most recent call last):
File "<string>", line 3, in <module>
TypeError: can only concatenate str (not "int") to str
As seen in the code above, the direct concatenation of a string and an integer is not possible in the Python programming language.
In the following parts of this guide, we’ll focus on the different ways you can successfully implement the concatenation of an integer and a string.
Use the str()
Function to Implement String and Integer Concatenation in Python
The easiest and simplest way to successfully implement the concatenation between a string and an integer is to manually convert the integer value into a string value by using the str()
function.
The following code uses the str()
function to implement string and integer concatenation in Python.
x = "My crypto portfolio amount in dollars is "
y = 5000
print(x + str(y))
Output:
My crypto portfolio amount in dollars is 5000
Use String Formatting With the Modulo %
Sign for String and Integer Concatenation in Python
String formatting provides a wide variety of customization options for the user to choose from in the print
statement. The %
sign is sometimes also referred to as the interpolation or string formatting operator.
There are many ways to implement string formatting, with the %
sign being the oldest of the available methods that work on almost all versions of Python.
The %
sign and a letter representing the conversion type are marked as a placeholder for the variable. The following code uses the modulo %
sign to implement a string and integer concatenation in Python.
x = "My crypto portfolio amount in dollars is "
y = 5000
print("%s%s" % (x, y))
Output:
My crypto portfolio amount in dollars is 5000
Use String Formatting With the str.format()
Function for String and Integer Concatenation in Python
This method is another way to achieve string formatting, in which brackets {}
mark the places in the print
statement where the variables need to be substituted.
The str.format()
function was introduced in Python 2.6 and is available to use in all Python versions released after Python 2.6 to Python 3.5.
The following code uses the str.format()
function to implement string and integer concatenation in Python.
x = "My crypto portfolio amount in dollars is "
y = 5000
print("{}{}".format(x, y))
Output:
My crypto portfolio amount in dollars is 5000
Use the f-string
for String Formatting in Python
This method is relatively the newest one in Python to implement string formatting. It’s introduced in Python 3.6 and can be used for the newer and latest versions of Python.
Being faster and easier than its other two peers, %
sign and str.format()
, it is more efficient and has the speed advantage when implementing string formatting in Python.
The following code uses the fstring
formatting to implement string and integer concatenation in Python.
x = "My crypto portfolio amount in dollars is "
y = 5000
print(f"{x}{y}")
Output:
My crypto portfolio amount in dollars is 5000
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python