在 Python 中連線字串和整數值
-
在 Python 中使用
str()
函式實現字串和整數連線 -
在 Python 中使用帶有模
%
符號的字串格式進行字串和整數連線 -
在 Python 中將字串格式與
str.format()
函式用於字串和整數連線 -
在 Python 中使用
f-string
進行字串格式化
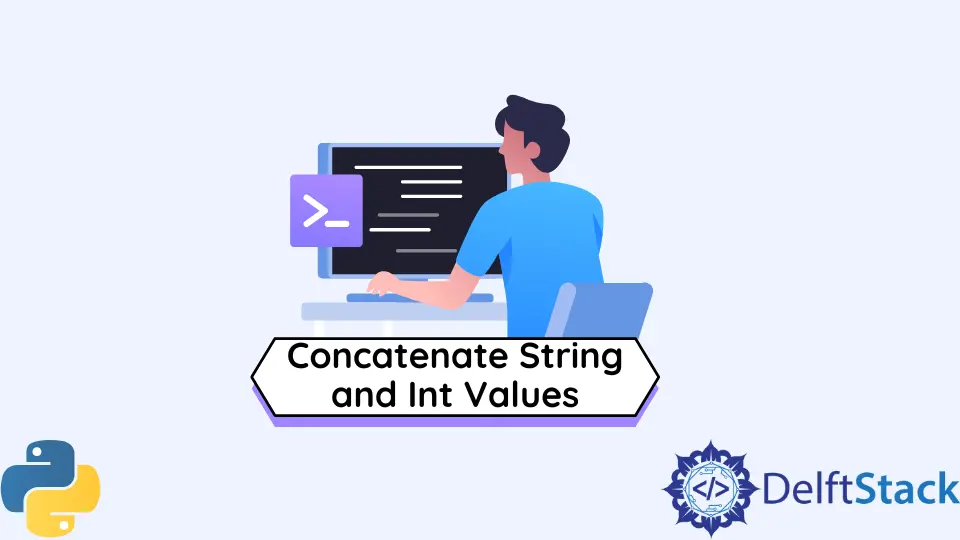
連線可以定義為將兩個字串整合到一個物件中。在 Python 中,你可以使用 +
運算子執行連線。在這裡,我們將討論如何在 Python 中成功實現字串和整數連線。
在大多數程式語言中,你經常會遇到這樣的操作:如果要在字串和整數之間進行連線過程,語言會自動先將整數值轉換為字串值,然後再繼續進行字串連線過程。
Python 是此操作的一個例外,如果將字串與整數連線,則會引發錯誤。
以下程式碼嘗試在 Python 中實現字串和整數連線。
x = "My crypto portfolio amount in dollars is "
y = 5000
print(x + y)
輸出:
Traceback (most recent call last):
File "<string>", line 3, in <module>
TypeError: can only concatenate str (not "int") to str
從上面的程式碼可以看出,在 Python 程式語言中,字串和整數的直接連線是不可能的。
在本指南的以下部分中,我們將重點介紹可以成功實現整數和字串連線的不同方法。
在 Python 中使用 str()
函式實現字串和整數連線
成功實現字串和整數之間的連線的最簡單和最簡單的方法是使用 str()
函式手動將整數值轉換為字串值。
以下程式碼使用 str()
函式在 Python 中實現字串和整數連線。
x = "My crypto portfolio amount in dollars is "
y = 5000
print(x + str(y))
輸出:
My crypto portfolio amount in dollars is 5000
在 Python 中使用帶有模 %
符號的字串格式進行字串和整數連線
字串格式提供了多種自定義選項供使用者在 print
語句中進行選擇。%
符號有時也稱為插值或字串格式化運算子。
有很多方法可以實現字串格式化,%
符號是最古老的可用方法,幾乎適用於所有版本的 Python。
%
符號和代表轉換型別的字母被標記為變數的佔位符。以下程式碼使用模 %
符號在 Python 中實現字串和整數連線。
x = "My crypto portfolio amount in dollars is "
y = 5000
print("%s%s" % (x, y))
輸出:
My crypto portfolio amount in dollars is 5000
在 Python 中將字串格式與 str.format()
函式用於字串和整數連線
這種方法是另一種實現字串格式化的方法,其中括號 {}
標記了 print
語句中需要替換變數的位置。
str.format()
函式是在 Python 2.6 中引入的,可用於 Python 2.6 到 Python 3.5 之後釋出的所有 Python 版本。
以下程式碼使用 str.format()
函式在 Python 中實現字串和整數連線。
x = "My crypto portfolio amount in dollars is "
y = 5000
print("{}{}".format(x, y))
輸出:
My crypto portfolio amount in dollars is 5000
在 Python 中使用 f-string
進行字串格式化
這種方法是 Python 中實現字串格式化的最新方法。它是在 Python 3.6 中引入的,可用於更新和最新版本的 Python。
它比其他兩個同行,%
符號和 str.format()
更快更容易,在 Python 中實現字串格式時效率更高並且具有速度優勢。
以下程式碼使用 fstring
格式在 Python 中實現字串和整數連線。
x = "My crypto portfolio amount in dollars is "
y = 5000
print(f"{x}{y}")
輸出:
My crypto portfolio amount in dollars is 5000
Vaibhhav is an IT professional who has a strong-hold in Python programming and various projects under his belt. He has an eagerness to discover new things and is a quick learner.
LinkedIn相關文章 - Python String
- 在 Python 中從字串中刪除逗號
- 如何以 Pythonic 方式檢查字符串是否為空
- 在 Python 中將字串轉換為變數名
- Python 如何去掉字串中的空格/空白符
- 如何在 Python 中從字串中提取數字
- Python 如何將字串轉換為時間日期 datetime 格式