Chaining Comparison Operators in Python
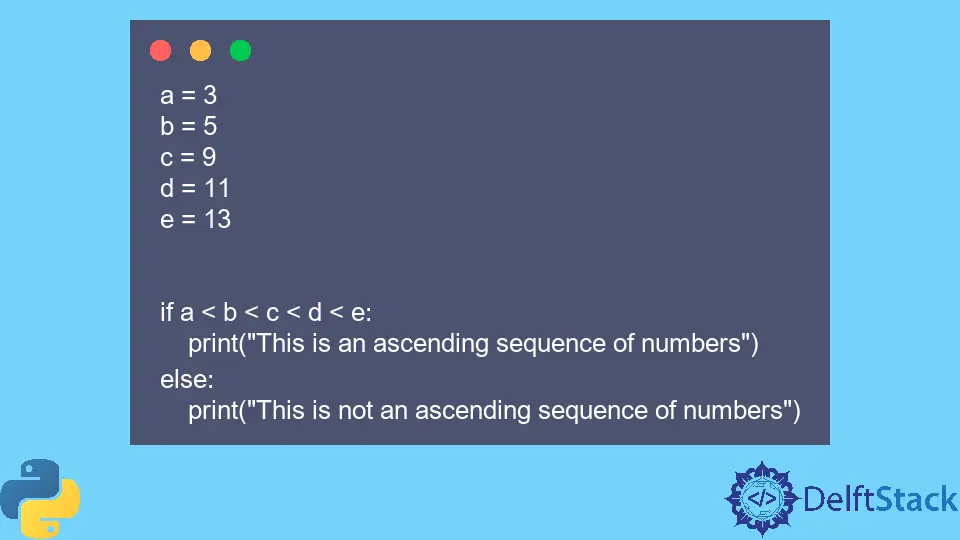
Comparisons are an essential part of all programming languages.
In Python, comparison operators are used in comparing values and return True
or False
depending on the condition being tested. These conditions include ascertaining whether values on the left or right side are equal, greater than, or less than a certain value.
Unlike arithmetic operators, which have precedence, comparison operators have the same priority, which is less than that of other operators such as bitwise operators and arithmetic operators.
Comparison operators are not restricted to numeric types but can also compare sequence types, set types, and boolean values.
Usually, comparison operators are combined using logical operators to form conditional statements. Comparison operators can also be used together with if
statements and loops such as for
and while
loops.
In the example below, comparison operators have been used with logical operators to form conditional statements that test the equality of two arithmetic values.
x = 5
y = 7
z = 10
if y > x and z > y:
print("z is the greatest number")
else:
print("z is not the greatest number")
Output:
z is the greatest number
As shown in the example below, we can chain the operators together instead of simultaneously using the logical operators and comparison operators. This method is more precise and easy to understand.
x = 5
y = 7
z = 10
if x < z > y:
print("z is the greatest number")
else:
print("z is not the greatest number")
Output:
z is the greatest number
In the example above, we have chained the comparison operators to determine if z
is the greatest number. Similarly, comparison operators can determine if certain numbers are equal using the equal to and not equal to operator.
length = 23
height = 23
width = 6
if length == height and height == width and width == length:
print("This is a cube")
else:
print("This is a cuboid")
Output:
This is a cuboid
In the code above, height
, width
, and length
are evaluated twice before completing all comparison cycles.
However, by chaining operators arbitrarily, we can rewrite the code above more precisely, as shown in the example below. The example below only evaluated the expression once.
if length == height == width:
print("This is a cube ")
else:
print("This is a cuboid ")
Output:
This is a cuboid
We can also use the concept of chaining operators to make sure that a sequence of certain values is equal. In the example given below, we effortlessly test the equality of three different values by chaining the values together.
a = 3
b = 5
c = 9
if a == b == c:
print("All the variables are equal")
else:
print("Variable are not the same")
Output:
Variable are not the same
Since the equality operator is transitive, we can conclude that, if a == b
and b == c
, the value of b
must be equal to c
.
However, we must be careful as the same equivalence or non-equivalence cannot be implied in non-transitive comparison operators such as the operator !=
used to test for non-equality.
Similarly, we can also chain the <
operator to determine if numbers in a sequence are strictly ascending or not.
a = 3
b = 5
c = 9
d = 11
e = 13
if a < b < c < d < e:
print("This is an ascending sequence of numbers")
else:
print("This is not an ascending sequence of numbers")
Output:
This is an ascending sequence of numbers
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn