在 Python 中的链式比较运算符
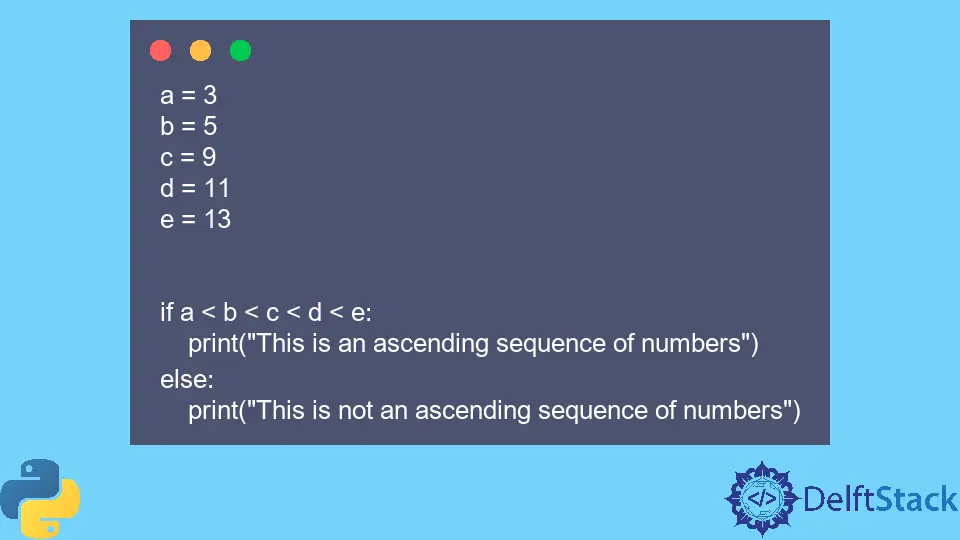
比较是所有编程语言的重要组成部分。
在 Python 中,比较运算符用于比较值并根据被测试的条件返回 True
或 False
。这些条件包括确定左侧或右侧的值是否等于、大于或小于某个值。
与具有优先级的算术运算符不同,比较运算符具有相同的优先级,其优先级低于其他运算符,例如位运算符和算术运算符。
比较运算符不仅限于数字类型,还可以比较序列类型、集合类型和布尔值。
通常,比较运算符使用逻辑运算符组合以形成条件语句。比较运算符也可以与 if
语句和循环一起使用,例如 for
和 while
循环。
在下面的示例中,比较运算符已与逻辑运算符一起使用以形成测试两个算术值是否相等的条件语句。
x = 5
y = 7
z = 10
if y > x and z > y:
print("z is the greatest number")
else:
print("z is not the greatest number")
输出:
z is the greatest number
如下例所示,我们可以将运算符链接在一起,而不是同时使用逻辑运算符和比较运算符。这种方法更精确,更容易理解。
x = 5
y = 7
z = 10
if x < z > y:
print("z is the greatest number")
else:
print("z is not the greatest number")
输出:
z is the greatest number
在上面的示例中,我们将比较运算符链接起来以确定 z
是否为最大数。类似地,比较运算符可以使用等于和不等于运算符确定某些数字是否相等。
length = 23
height = 23
width = 6
if length == height and height == width and width == length:
print("This is a cube")
else:
print("This is a cuboid")
输出:
This is a cuboid
在上面的代码中,height
、width
和 length
在完成所有比较周期之前被计算了两次。
但是,通过任意链接运算符,我们可以更精确地重写上面的代码,如下例所示。下面的示例仅对表达式求值一次。
if length == height == width:
print("This is a cube ")
else:
print("This is a cuboid ")
输出:
This is a cuboid
我们还可以使用链接运算符的概念来确保某些值的序列是相等的。在下面给出的示例中,我们通过将值链接在一起来轻松测试三个不同值的相等性。
a = 3
b = 5
c = 9
if a == b == c:
print("All the variables are equal")
else:
print("Variable are not the same")
输出:
Variable are not the same
由于等式运算符是传递的,我们可以得出结论,如果 a == b
和 b == c
,b
的值必须等于 c
。
但是,我们必须小心,因为在非传递比较运算符(例如用于测试不相等性的运算符!=
)中不能隐含相同的等价或不等价。
类似地,我们也可以链接 <
运算符来确定序列中的数字是否严格升序。
a = 3
b = 5
c = 9
d = 11
e = 13
if a < b < c < d < e:
print("This is an ascending sequence of numbers")
else:
print("This is not an ascending sequence of numbers")
输出:
This is an ascending sequence of numbers
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn