在 Python 中的鏈式比較運算子
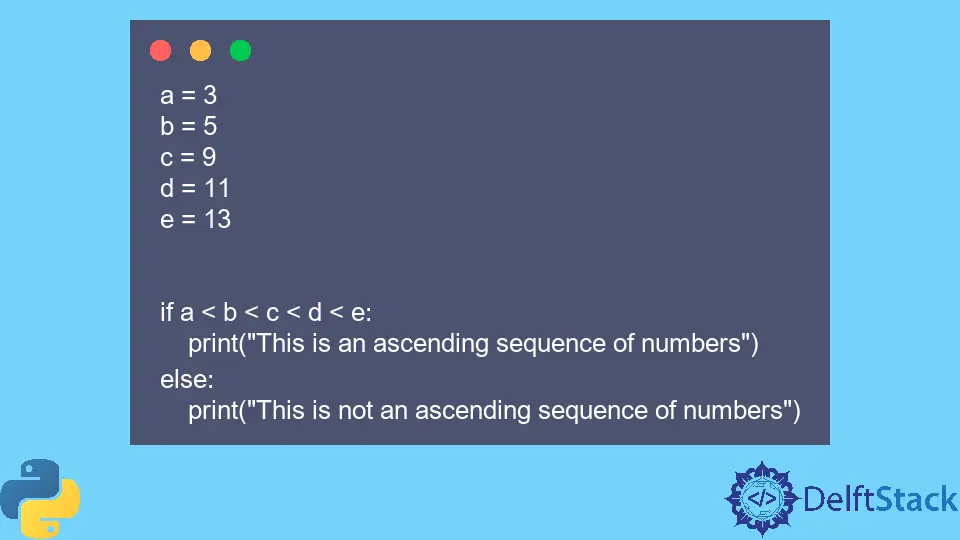
比較是所有程式語言的重要組成部分。
在 Python 中,比較運算子用於比較值並根據被測試的條件返回 True
或 False
。這些條件包括確定左側或右側的值是否等於、大於或小於某個值。
與具有優先順序的算術運算子不同,比較運算子具有相同的優先順序,其優先順序低於其他運算子,例如位運算子和算術運算子。
比較運算子不僅限於數字型別,還可以比較序列型別、集合型別和布林值。
通常,比較運算子使用邏輯運算子組合以形成條件語句。比較運算子也可以與 if
語句和迴圈一起使用,例如 for
和 while
迴圈。
在下面的示例中,比較運算子已與邏輯運算子一起使用以形成測試兩個算術值是否相等的條件語句。
x = 5
y = 7
z = 10
if y > x and z > y:
print("z is the greatest number")
else:
print("z is not the greatest number")
輸出:
z is the greatest number
如下例所示,我們可以將運算子連結在一起,而不是同時使用邏輯運算子和比較運算子。這種方法更精確,更容易理解。
x = 5
y = 7
z = 10
if x < z > y:
print("z is the greatest number")
else:
print("z is not the greatest number")
輸出:
z is the greatest number
在上面的示例中,我們將比較運算子連結起來以確定 z
是否為最大數。類似地,比較運算子可以使用等於和不等於運算子確定某些數字是否相等。
length = 23
height = 23
width = 6
if length == height and height == width and width == length:
print("This is a cube")
else:
print("This is a cuboid")
輸出:
This is a cuboid
在上面的程式碼中,height
、width
和 length
在完成所有比較週期之前被計算了兩次。
但是,通過任意連結運算子,我們可以更精確地重寫上面的程式碼,如下例所示。下面的示例僅對表示式求值一次。
if length == height == width:
print("This is a cube ")
else:
print("This is a cuboid ")
輸出:
This is a cuboid
我們還可以使用連結運算子的概念來確保某些值的序列是相等的。在下面給出的示例中,我們通過將值連結在一起來輕鬆測試三個不同值的相等性。
a = 3
b = 5
c = 9
if a == b == c:
print("All the variables are equal")
else:
print("Variable are not the same")
輸出:
Variable are not the same
由於等式運算子是傳遞的,我們可以得出結論,如果 a == b
和 b == c
,b
的值必須等於 c
。
但是,我們必須小心,因為在非傳遞比較運算子(例如用於測試不相等性的運算子!=
)中不能隱含相同的等價或不等價。
類似地,我們也可以連結 <
運算子來確定序列中的數字是否嚴格升序。
a = 3
b = 5
c = 9
d = 11
e = 13
if a < b < c < d < e:
print("This is an ascending sequence of numbers")
else:
print("This is not an ascending sequence of numbers")
輸出:
This is an ascending sequence of numbers
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn