The Walrus Operator := in Python
- Understanding the Walrus Operator
- Use Cases for the Walrus Operator
- Enhancing List Comprehensions with the Walrus Operator
- Conclusion
- FAQ
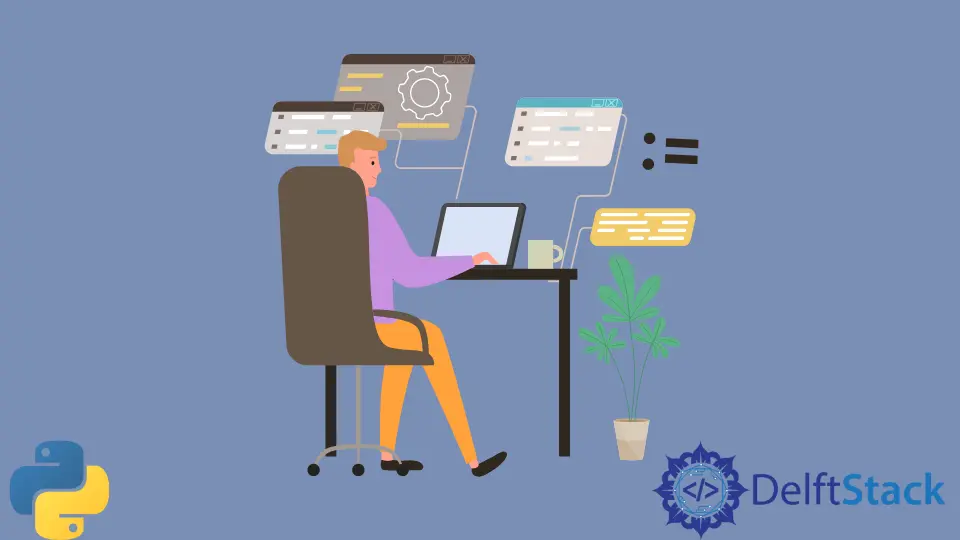
Python has a knack for introducing features that make coding more efficient and enjoyable. One such feature is the walrus operator, introduced in Python 3.8. This unique operator, represented as :=
, allows you to assign values to variables as part of an expression. This can lead to cleaner and more concise code, especially in scenarios where you want to both assign a value and use it immediately.
In this tutorial, we will dive deep into the walrus operator, exploring its syntax, use cases, and how it can enhance your Python programming experience. Whether you are a seasoned developer or just starting, understanding this operator will undoubtedly add a valuable tool to your coding arsenal.
Understanding the Walrus Operator
The walrus operator is primarily used for assignment expressions. Unlike traditional assignment statements, which stand alone, the walrus operator allows you to perform an assignment within an expression. This can be particularly useful in loops, conditional statements, and comprehensions.
For instance, consider a situation where you want to read user input and immediately check its validity. Instead of separating the assignment and the check into two lines, you can use the walrus operator to do both simultaneously.
Here’s a simple example:
if (n := input("Enter a number: ")) != "0":
print(f"You entered: {n}")
In this code, the input is assigned to n
while also being checked if it’s not equal to “0”. This reduces the need for a separate line to store the input, making the code cleaner and more efficient.
Output:
Enter a number: 5
You entered: 5
The walrus operator enhances readability by reducing redundancy. You can see that the input is captured and used in a single line, making the code easier to follow.
Use Cases for the Walrus Operator
The walrus operator shines in various scenarios. One of its most common use cases is within loops, particularly when dealing with data streams or reading large files. By employing the walrus operator, you can streamline your code and avoid unnecessary lines.
For example, consider reading lines from a file until you reach an empty line:
with open("file.txt") as f:
while (line := f.readline().strip()):
print(line)
In this case, the walrus operator allows you to read a line and check if it’s not empty all in one go. This avoids the need for an additional line to store the result of f.readline()
, leading to more concise code.
Output:
This is the first line.
This is the second line.
Using the walrus operator in loops can significantly enhance performance when processing large datasets or streaming data. It minimizes the number of lines of code and promotes clarity, making it easier for others (or even yourself in the future) to understand your logic.
Enhancing List Comprehensions with the Walrus Operator
List comprehensions are another area where the walrus operator can be particularly beneficial. When you want to filter or transform data while simultaneously assigning values, the walrus operator can simplify your code.
Consider the following example where we want to create a list of squared numbers from a given range, but only for even numbers:
squared_evens = [square for num in range(10) if (square := num ** 2) % 2 == 0]
In this case, the walrus operator allows you to calculate num ** 2
and assign it to square
while checking if it’s even. This reduces the need for a separate calculation step and keeps the comprehension concise.
Output:
[0, 4, 16, 36, 64]
By using the walrus operator in list comprehensions, you can create more efficient and readable code. This operator not only improves performance but also enhances the expressiveness of your code, making it easier to convey your intentions.
Conclusion
The walrus operator is a powerful addition to Python that allows for more concise and efficient coding practices. By enabling assignment expressions, it helps streamline your code, particularly in loops and comprehensions. As you incorporate the walrus operator into your programming toolkit, you’ll find that it enhances both readability and performance. Embracing this feature can lead to cleaner code and a more enjoyable coding experience. So, the next time you’re writing Python, consider using the walrus operator to simplify your assignments and expressions.
FAQ
-
What is the walrus operator in Python?
The walrus operator is a new syntax introduced in Python 3.8, allowing assignment within expressions using:=
. -
When should I use the walrus operator?
Use the walrus operator when you want to assign a value and use it immediately within an expression, particularly in loops and conditionals.
-
Is the walrus operator mandatory to use in Python?
No, the walrus operator is optional. It is a convenience feature aimed at improving code readability and efficiency. -
Can I use the walrus operator with functions?
Yes, you can use the walrus operator with functions, especially when you want to assign the output of a function call to a variable while using it in a condition. -
Are there any downsides to using the walrus operator?
While the walrus operator can enhance readability, overusing it or using it in complex expressions may lead to confusion. It’s important to strike a balance for clarity.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn