How to Overload Operator in Python
- Overload Operators in Python
-
Overload the
+
Operator in Python -
Overload the
>
Operator in Python -
Overload the
==
Operator in Python
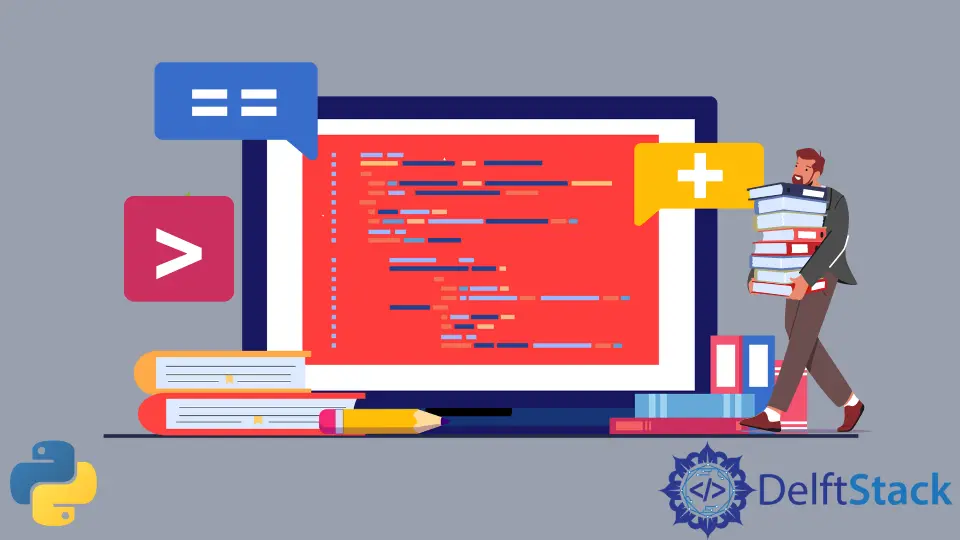
Operator overloading means changing the way operators behave in different situations. It is a type of polymorphism.
With operator overloading, we can add extra meaning or functionality to an operator to perform more than one operation.
For example, the +
operator performs addition with integer operands. But when used with string operands, it performs concatenation because the +
operator is overloaded.
Overload Operators in Python
Python provides a way to overload operators with user-defined data types through the Magic methods. These are special methods automatically invoked when we use the operator associated with them.
They are also known as Dunder methods because they start and end with a double underscore.
For example, when we write the operator +
, the magic method __add__()
is automatically called on the backend. Therefore, if we want to perform operator overloading with certain operators, we only have to change the code of their magic methods.
Overload the +
Operator in Python
In the following code, we have a user-defined class in which we have implemented the magic method __add__()
to overload the +
operator.
When we write int1 + int2
, actually on the back-end the magic method __add()__
is called as int1.__add__(int2)
. The same working goes for the strings as well.
When we write str1 + str2
, internally the magic method is called as str1.__add__(str2)
.
Example Code:
# Python 3.x
class MyAddion:
def __init__(self, a):
self.a = a
def __add__(self, b):
return self.a + b.a
int1 = MyAddion(2)
int2 = MyAddion(2)
str1 = MyAddion("Delft")
str2 = MyAddion("Stack")
print(int1 + int2)
print(str1 + str2)
Output:
#Python 3.x
4
DelftStack
Overload the >
Operator in Python
Here, we have overloaded the >
operator in the MyComp
class. When we write the operator >
, the magic method __gt__()
is invoked internally.
So when we write int1 > int2
, the magic method __gt__()
is called as int1.__gt__(int2)
. This method returns true
if the condition is true; else, it returns false
.
Example Code:
# Python 3.x
class MyComp:
def __init__(self, a):
self.a = a
def __gt__(self, b):
if self.a > b.a:
return True
else:
return False
int1 = MyComp(5)
int2 = MyComp(3)
if int1 > int2:
print(int1.a, "is greater than", int2.a)
else:
print(int2.a, "is greater than", int1.a)
Output:
#Python 3.x
5 is greater than 3
Overload the ==
Operator in Python
Here, we have overloaded the equality operator ==
in our class MyEquality
. When we write int1 == int2
, internally the magic method __eq__()
will be invoked as int1.__eq__(int2)
.
If both operands are equal, it will return Equal
; else, it will return Not Equal
.
Example Code:
# Python 3.x
class MyEquality:
def __init__(self, a):
self.a = a
def __eq__(self, b):
if self.a == b.a:
return "Equal"
else:
return "Not equal"
int1 = MyEquality(3)
int2 = MyEquality(3)
print(int1 == int2)
Output:
#Python 3.x
Equal
It’s worth noting that we cannot change the number of operands for an operator in case of operator overloading. We cannot overload a unary operator as a binary operator and vice versa.
For example, we cannot overload the ~
invert operator (a unary operator) as a binary operator.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn